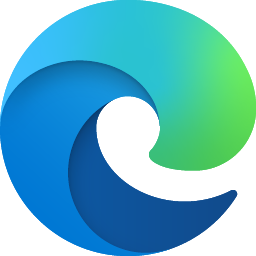
Thanks for all your support.
Issue was with protected mode for IE has to be disabled. It was restricting script to process further in my case.
And script started working further.
This browser is no longer supported.
Upgrade to Microsoft Edge to take advantage of the latest features, security updates, and technical support.
I am using selenium 3.141.59 to automate gmail application as an example in Edge in IE Mode.
If I use Selenium 4.0.0 or greater , PageFactory.initElements doesn't support .
I have to execute script for below environment :
Windows 11 (64 bit) Edge 105.0.1343.50 ( Open in IE mode. Its requirement of an application)
DriverManager.java
package managers;
import java.io.IOException;
import java.net.InetAddress;
import java.net.UnknownHostException;
import java.util.Map;
import java.util.concurrent.TimeUnit;
import org.openqa.selenium.UnexpectedAlertBehaviour;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.edge.EdgeDriver;
import org.openqa.selenium.ie.InternetExplorerDriver;
import org.openqa.selenium.ie.InternetExplorerOptions;
import org.openqa.selenium.remote.DesiredCapabilities;
import org.testng.annotations.AfterClass;
import org.testng.annotations.AfterTest;
import org.testng.annotations.BeforeClass;
import org.testng.annotations.BeforeTest;
import org.testng.annotations.Parameters;
public class DriverManager {
public WebDriver driver;
DesiredCapabilities capabilities;
@SuppressWarnings("unchecked")
@Parameters({ "browserName" })
@BeforeTest(alwaysRun = true)
public void initialize(String browser) throws IOException, InterruptedException {
if (browser.equalsIgnoreCase("edge")) {
System.setProperty("webdriver.edge.driver",
"D:\\My_Workspace\\EdgeInIEModeTest\\drivers\\IEDriverServer32bit.exe\\msedgedriver.exe");
capabilities = new DesiredCapabilities();
// Creating an object of EdgeDriver
driver = new EdgeDriver();
driver.manage().window().maximize();
// Deleting all the cookies
driver.manage().deleteAllCookies();
// Specifiying pageLoadTimeout and Implicit wait
driver.manage().timeouts().pageLoadTimeout(40, TimeUnit.SECONDS);
driver.manage().timeouts().implicitlyWait(30, TimeUnit.SECONDS);
} else if (browser.equalsIgnoreCase("edgeForWindows11")) {
// Selenium 4 + version
// System.out.println("edgeForWindows11 IN");
// System.setProperty("webdriver.ie.driver",
//
// "D:\\My_Workspace\\EdgeInIEModeTest\\drivers\\IEDriverServer32bit.exe\\IEDriverServer32bit.exe");
//
// InternetExplorerOptions ieOptions = new InternetExplorerOptions();
// ieOptions.attachToEdgeChrome();
// ieOptions.withEdgeExecutablePath("C:\\Program Files (x86)\\Microsoft\\Edge\\Application\\msedge.exe");
//
// driver = new InternetExplorerDriver(ieOptions);
// Selenium 3.141.59
System.setProperty("webdriver.ie.driver",
"D:\\IEDriver\\IEDriverServer.exe");
InternetExplorerOptions edgeIe11Options = new InternetExplorerOptions();
Map<String, Object> ops = (Map<String, Object>) edgeIe11Options.getCapability("se:ieOptions");
ops.put("ie.edgechromium", true);
ops.put("ie.edgepath", "C:\\Program Files (x86)\\Microsoft\\Edge\\Application\\msedge.exe"); // Edge Browser
// directory
// path
edgeIe11Options.introduceFlakinessByIgnoringSecurityDomains();
edgeIe11Options.ignoreZoomSettings();
edgeIe11Options.enablePersistentHovering();
edgeIe11Options.takeFullPageScreenshot();
edgeIe11Options.disableNativeEvents();
edgeIe11Options.requireWindowFocus();
edgeIe11Options.destructivelyEnsureCleanSession();
edgeIe11Options.setCapability("ignoreProtectedModeSettings", true);
edgeIe11Options.setUnhandledPromptBehaviour(UnexpectedAlertBehaviour.IGNORE);
driver = new InternetExplorerDriver(edgeIe11Options);
}
}
public static String getIPAddress() {
InetAddress IP = null;
try {
IP = InetAddress.getLocalHost();
} catch (UnknownHostException e) {
e.printStackTrace();
}
return IP.getHostAddress();
}
public void launchGmailApplication() throws InterruptedException, IOException {
System.out.println("In launchApplication method for EdgeInIEMode Windows 11");
driver.manage().window().maximize();
driver.manage().timeouts().implicitlyWait(30, TimeUnit.SECONDS);
driver.get("http://www.google.com");
}
@AfterTest(alwaysRun = true)
public void TeardownTest() {
System.out.println(driver.getCurrentUrl());
if (null != driver) {
driver.close();
driver.quit();
}
}
}
BasePage.Java
package pages;
import org.openqa.selenium.WebDriver;
public abstract class BasePage {
public WebDriver driver;
public BasePage(WebDriver driver) {
// TODO Auto-generated constructor stub
this.driver = driver;
}
}
LogInPage.Java
package pages;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.support.FindBy;
import org.openqa.selenium.support.PageFactory;
public class LogInPage extends BasePage {
@FindBy(xpath = "//input[@aria-label='Email or phone']")
WebElement userNameInput;
@FindBy(xpath = "//input[@name='Passwd']")
WebElement passwordInput;
@FindBy(xpath = "")
WebElement signInButton;
@FindBy(xpath = "//a[text()='Gmail']")
WebElement gmailMenuButotn;
@FindBy(xpath = "//span[text()='Next']")
WebElement nextButtton;
@FindBy(xpath = "//a[text()='Sign in']")
WebElement gmailSignInButton;
public void clickOnGmailMenuOption() {
gmailMenuButotn.click();
}
public void clickOnSignInButton() {
gmailSignInButton.click();
}
public void logInToGmailApplication() {
userNameInput.sendKeys("username");
nextButtton.click();
passwordInput.sendKeys("password");
}
public LogInPage(WebDriver driver) {
super(driver);
PageFactory.initElements(driver, this);
}
}
LogInTest.Java
import java.io.IOException;
import org.openqa.selenium.support.PageFactory;
import org.testng.annotations.BeforeClass;
import org.testng.annotations.BeforeTest;
import org.testng.annotations.Test;
import managers.DriverManager;
import pages.LogInPage;
public class LogInTest extends DriverManager {
public LogInPage loginPage;
@BeforeTest(alwaysRun = true)
public void launchApplication() throws InterruptedException, IOException {
loginPage = PageFactory.initElements(driver, LogInPage.class);
launchGmailApplication();
System.out.println("BeforeTest End");
}
@Test(priority = 0)
public void invokeDriver() {
System.out.println("Hello");
loginPage.clickOnGmailMenuOption();
loginPage.clickOnSignInButton();
loginPage.logInToGmailApplication();
}
}
POM.xml
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.cemtrex.com</groupId>
<artifactId>EdgeInIEModeTest</artifactId>
<version>0.0.1-SNAPSHOT</version>
<dependencies>
<dependency>
<groupId>org.testng</groupId>
<artifactId>testng</artifactId>
<version>7.1.0</version>
<scope>test</scope>
</dependency>
<dependency>
<groupId>org.testng</groupId>
<artifactId>testng</artifactId>
<version>7.3.0</version>
<scope>compile</scope>
</dependency>
<dependency>
<groupId>org.seleniumhq.selenium</groupId>
<artifactId>selenium-java</artifactId>
<version>3.141.59</version>
</dependency>
</dependencies>
<profiles>
<profile>
<id>if-suite-exists</id>
<activation>
<property>
<name>!env.SUITES</name>
</property>
</activation>
<properties>
<suites>GlobalSuite</suites>
</properties>
</profile>
<!-- browsers -->
<profile>
<id>firefox</id>
<properties>
<capabilities>/firefox.capabilities</capabilities>
</properties>
<activation>
<activeByDefault>true</activeByDefault>
</activation>
</profile>
<profile>
<id>chrome</id>
<properties>
<capabilities>/chrome.capabilities</capabilities>
</properties>
</profile>
<profile>
<id>ie</id>
<properties>
<capabilities>/ie.capabilities</capabilities>
</properties>
</profile>
</profiles>
<properties>
<suites>${env.SUITES}</suites>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
<maven.compiler.source>1.8</maven.compiler.source>
<maven.compiler.target>1.8</maven.compiler.target>
</properties>
<build>
<resources>
<resource>
<directory>src/main/java/resources</directory>
<filtering>true</filtering>
</resource>
</resources>
<testResources>
<testResource>
<directory>src/test/java/resources</directory>
<filtering>true</filtering>
</testResource>
</testResources>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-compiler-plugin</artifactId>
<version>3.1</version>
<configuration>
<source>1.8</source>
<target>1.8</target>
</configuration>
</plugin>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-surefire-plugin</artifactId>
<version>2.20</version>
<!--<inherited>true</inherited> -->
<configuration>
<!--<testFailureIgnore>false</testFailureIgnore> -->
<suiteXmlFiles>
<suiteXmlFile>src/test/resources/suites/${suites}.xml</suiteXmlFile>
</suiteXmlFiles>
</configuration>
</plugin>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-dependency-plugin</artifactId>
<version>3.1.2</version>
<configuration>
<encoding>UTF-8</encoding>
</configuration>
</plugin>
</plugins>
</build>
</project>
After Execute:
Thanks for all your support.
Issue was with protected mode for IE has to be disabled. It was restricting script to process further in my case.
And script started working further.