How to implement the in-app purchase feature in xamarin forms ios project
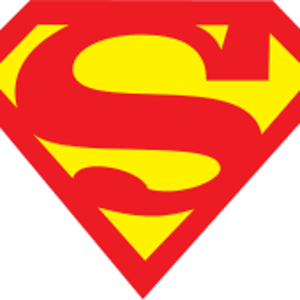
I am trying to implement the in-app purchase inside my Xamarin forms ios application. I have created one in-app purchase product on the app store. I need to do the payment when subscribing the application. I choose Auto-Renewable Subscription
as the in-app purchase type.
After that how can I implement that feature on the app side? Do I need to use any Dependency Service
for this? Which NuGet package do we need to use, is it Plugin.InAppBilling
? I researched this and was confused about the app side integration. Any other setup I need to do to implement this feature.
I am looking for the specific codes that connect the application and the in-app purchase property created on the AppStore.
Only for ios, I am planning to implement the in-app purchase and for android, I am planning to do the payment with stripe. Is google accept stripe payment for this or are they also forced to implement in-app purchases?
Following is my scenario:
The subscription is for the usage of applications from the app/play store. The admin will subscribe to it and all the other users can use it for free.
I tried the below codes from this blog. But it looks likes for the android part, and not mentioned the ios part implementation.
// Connect to the service here
await CrossInAppBilling.Current.ConnectAsync();
// Check if there are pending orders, if so then subscribe
var purchases = await CrossInAppBilling.Current.GetPurchasesAsync(ItemType.InAppPurchase);
if (purchases?.Any(p => p.State == PurchaseState.PaymentPending) ?? false)
{
Plugin.InAppBilling.InAppBillingImplementation.OnAndroidPurchasesUpdated = (billingResult, purchases) =>
{
// decide what you are going to do here with purchases
// probably acknowledge
// probably disconnect
};
}
else
{
await CrossInAppBilling.Current.DisconnectAsync();
}
As per the same blog, I have updated AppDelegate
like below:
public override bool FinishedLaunching(UIApplication app, NSDictionary options)
{
global::Xamarin.Forms.Forms.Init();
LoadApplication(new App());
//initialize current one.
Plugin.InAppBilling.InAppBillingImplementation.OnShouldAddStorePayment = OnShouldAddStorePayment;
var current = Plugin.InAppBilling.CrossInAppBilling.Current;
return base.FinishedLaunching(app, options);
}
bool OnShouldAddStorePayment(SKPaymentQueue queue, SKPayment payment, SKProduct product)
{
// true in app purchase is initiated, false cancels it.
// you can check if it was already purchased.
return true;
}
On the MainPage, they have added the below code to purchase:
private async void ButtonNonConsumable_Clicked(object sender, EventArgs e)
{
var id = "iaptest";
try
{
await CrossInAppBilling.Current.ConnectAsync();
var purchase = await CrossInAppBilling.Current.PurchaseAsync(id, ItemType.InAppPurchase);
if (purchase == null)
{
await DisplayAlert(string.Empty, "Did not purchase", "OK");
}
else
{
if (!purchase.IsAcknowledged && Device.RuntimePlatform == Device.Android)
await CrossInAppBilling.Current.AcknowledgePurchaseAsync(purchase.PurchaseToken);
await DisplayAlert(string.Empty, "We did it!", "OK");
}
}
catch (Exception ex)
{
await DisplayAlert(string.Empty, "Did not purchase: " + ex.Message, "OK");
Console.WriteLine(ex);
}
finally
{
await CrossInAppBilling.Current.DisconnectAsync();
}
}
On this code they are checking the platform is android: Device.RuntimePlatform == Device.Android
. This code is on the portable project, so how I can do the same for ios? And my purchase type is auto renewable subscription, that part is empty on this blog.
private async void ButtonRenewingSub_Clicked(object sender, EventArgs e)
{
}