Hi,
I know that it was asked a lot, but it seems that nobody figured it out.
I'm trying to do a simple thing. Get the calendar events from a user. I followed the instructions here: https://learn.microsoft.com/en-us/graph/api/calendar-list-events?view=graph-rest-1.0&tabs=http
and It just does not work. I have all the IDs I need, I opened a personal Microsoft account (currently I'm on the trial), and the account is connect to the user in the Azure AD.
This is my code:
import msal
import r
CLIENT_SECRET = "client secret"
APP_ID = "app id"
TENANT_ID = 'tenant id'
USER_ID = 'user id'
AUTHORITY_URL = f'https://login.microsoftonline.com/{TENANT_ID}'
SCOPES = ['https://graph.microsoft.com/.default']
GRAPH_ENDPOINT = f'https://graph.microsoft.com/v1.0/users/{USER_ID}/calendar/events'
GRAPH_ENDPOINT2 = f'https://graph.microsoft.com/v1.0/users/{USER_ID}'
def get_access_token():
app = msal.ConfidentialClientApplication(
CLIENT_ID,
authority=AUTHORITY_URL,
client_credential=CLIENT_SECRET,
)
result = app.acquire_token_for_client(scopes=SCOPES)
if "access_token" in result:
print(result['access_token'])
return result['access_token']
else:
print("Error obtaining access token:")
print(result.get("error"))
print(result.get("error_description"))
print(result.get("correlation_id")) # You might need this when reporting a bug
raise Exception("Could not acquire access token")
def get_calendar_events(access_token):
headers = {
'Authorization': f'Bearer {access_token}'
}
response = requests.get(GRAPH_ENDPOINT, headers=headers)
if response.status_code == 200:
return response.json()
else:
print(f"API call failed with status code {response.status_code}")
print(response.json())
return None
def make_graph_api_call2(access_token):
headers = {
'Authorization': f'Bearer {access_token}'
}
response = requests.get(GRAPH_ENDPOINT2, headers=headers)
if response.status_code == 200:
return response.json()
else:
print(f"API call failed with status code {response.status_code}")
print(response.text)
return None
if __name__ == '__main__':
token = get_access_token()
users_info = make_graph_api_call2(token)
print(users_info)
events_info = get_calendar_events(token)
print(events_info)
The results for make_graph_api_call2 are working fine (just getting my details), but for the make_graph_api_call (the events) I get an error:
{'error': {'code': 'OrganizationFromTenantGuidNotFound', 'message': "The tenant for tenant guid 'xxxxxxx' does not exist.", 'innerError': {'oAuthEventOperationId': 'xxxxxx', 'oAuthEventcV': 'xxxxxxx', 'errorUrl': 'https://aka.ms/autherrors#error-InvalidTenant', 'requestId': 'xxxxxx', 'date': '2024-06-19T17:33:21'}}}
I gave the permission from Azure:
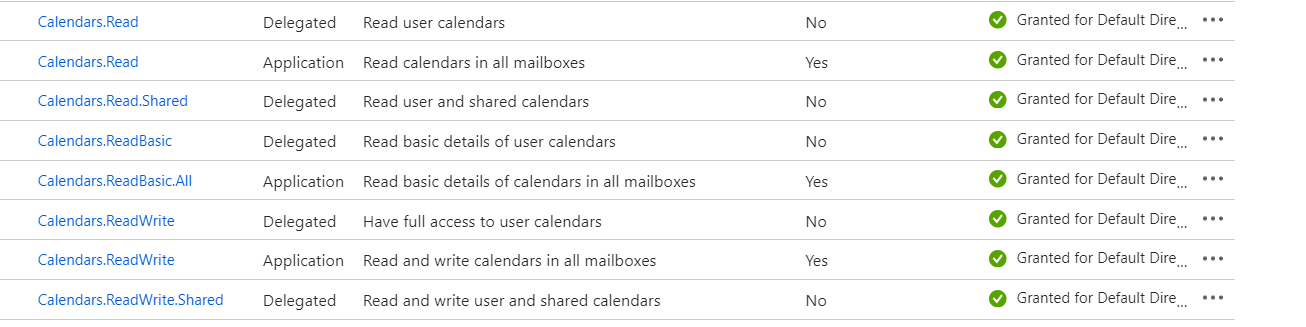
Can someone please help me to understand how to configure this? Maybe give me a guide for all the steps I need to make? It needs to be simple and I stuck on it over a week now.
Thank you!