Can you please advise on how I can close the modal after retrieving the products?
How about:
(1) Add button like below to your modal and click it,
<button type="button"
class="btn btn-secondary"
data-bs-dismiss="modal">
Close
</button>
or
(2) Use javascript mothods as shown below?
let divModal = document.getElementById('templatemo_search');
let myModal = new bootstrap.Modal(divModal);
myModal.show();
myModal.hide();
As @Bruce (SqlWork.com) mentioned in his comment below, myModal.hide();
of item (2) in my answer above does not work in Blazor WASM.
Sorry about oversight. myModal.hide();
of item (2) in my answer above works when the reference to modal is properly obtained. See exampleJsInterop.js below for correction.
However, item (1) can close the modal as expected. Shown below is sample. Blazor WASM project created using the template of Visual Studio 2022 version 17.12.2 is used. Target framework is .NET 9.0.
exampleJsInterop.js
//window.showBootstrapModal = () => {
// let divModal = document.getElementById('staticBackdrop');
// let myModal = new bootstrap.Modal(divModal);
// myModal.show();
//}
//window.closeBootstrapModal = () => {
// let divModal = document.getElementById('staticBackdrop');
// let myModal = new bootstrap.Modal(divModal);
// myModal.hide();
//}
// closeBootstrapModal method above does not work because
// myModal obtained in closeBootstrapModal method is
// different from myModal in showBootstrapModal method.
// Correction:
// Modal can be closed by closeBootstrapModal method
// once Modal has been opened by showBootstrapModal method.
let myModal;
window.showBootstrapModal = () => {
let divModal = document.getElementById('staticBackdrop');
myModal = new bootstrap.Modal(divModal);
myModal.show();
}
window.closeBootstrapModal = () => {
if (myModal != undefined) {
myModal.hide();
}
}
index.html
Script tags are added as shown below. Please note that bootstrap.js is included in the project created using template of Visual Studio.
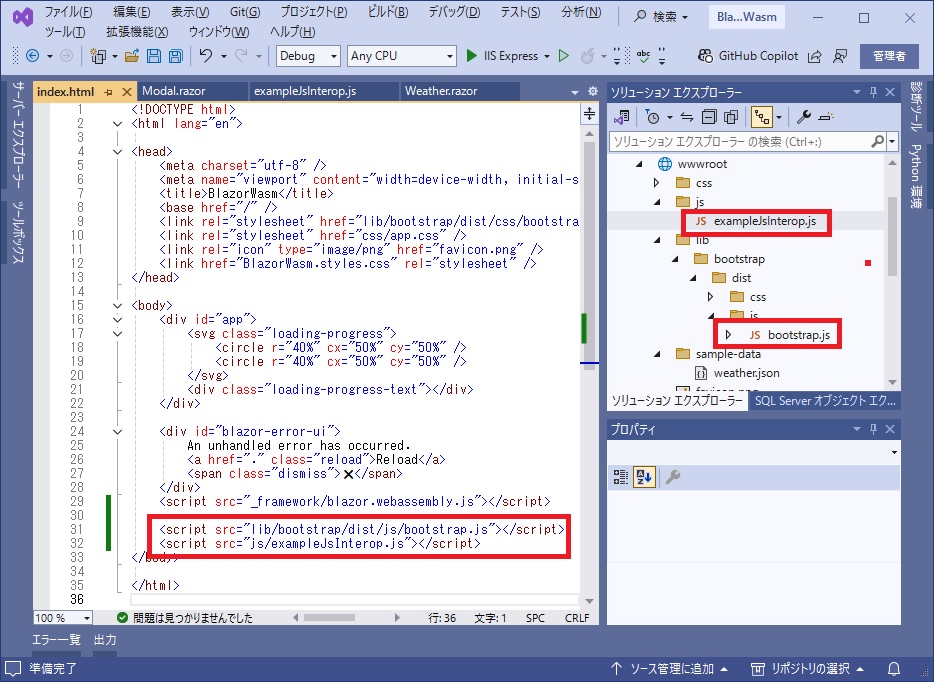
Modal.Razor
@page "/modal"
@inject IJSRuntime JSRuntime;
@inject HttpClient Http
<PageTitle>Bootstrap Modal</PageTitle>
<!-- Modal -->
<div class="modal fade"
id="staticBackdrop"
data-bs-backdrop="static"
data-bs-keyboard="false" tabindex="-1"
aria-labelledby="staticBackdropLabel"
aria-hidden="true">
<div class="modal-dialog">
<div class="modal-content">
<div class="modal-header">
<h5 class="modal-title"
id="staticBackdropLabel">
Modal title
</h5>
<button type="button"
class="btn-close"
data-bs-dismiss="modal"
aria-label="Close">
</button>
</div>
<div class="modal-body">
@if (forecasts == null)
{
<p><em>Loading...</em></p>
}
else
{
<table class="table">
<thead>
<tr>
<th>Date</th>
<th aria-label="Temperature in Celsius">Temp. (C)</th>
<th aria-label="Temperature in Farenheit">Temp. (F)</th>
<th>Summary</th>
</tr>
</thead>
<tbody>
@foreach (var forecast in forecasts)
{
<tr>
<td>@forecast.Date.ToShortDateString()</td>
<td>@forecast.TemperatureC</td>
<td>@forecast.TemperatureF</td>
<td>@forecast.Summary</td>
</tr>
}
</tbody>
</table>
}
</div>
<div class="modal-footer">
<button type="button"
class="btn btn-secondary"
data-bs-dismiss="modal">
Close
</button>
<button type="button" class="btn btn-danger"
@onclick="CloseBootstrapModal">
Close by JavaScript (does not work)
</button>
</div>
</div>
</div>
</div>
<h1>Bootstrap Modal</h1>
<!-- Via JavaScript -->
<button type="button" class="btn btn-primary"
@onclick="ShowBootstrapModal">
Open by JavaScript
</button>
<br />
<br />
<!-- Button trigger modal -->
<button type="button" class="btn btn-primary"
data-bs-toggle="modal"
data-bs-target="#staticBackdrop"
@onclick="LoadForecastData">
Launch static backdrop modal
</button>
@code {
private WeatherForecast[]? forecasts;
// protected override async Task OnInitializedAsync()
// {
// forecasts = await Http.GetFromJsonAsync<WeatherForecast[]>("sample-data/weather.json");
// }
private async Task LoadForecastData()
{
forecasts = await Http.GetFromJsonAsync<WeatherForecast[]>("sample-data/weather.json");
}
public class WeatherForecast
{
public DateOnly Date { get; set; }
public int TemperatureC { get; set; }
public string? Summary { get; set; }
public int TemperatureF => 32 + (int)(TemperatureC / 0.5556);
}
private async Task ShowBootstrapModal()
{
forecasts = await Http.GetFromJsonAsync<WeatherForecast[]>("sample-data/weather.json");
await JSRuntime.InvokeVoidAsync("showBootstrapModal");
}
// this does not work
private async Task CloseBootstrapModal()
{
await JSRuntime.InvokeVoidAsync("closeBootstrapModal");
}
}
Result
Model can be closed by cliking [Close] botton or X icon. Modal can also be closed by clicking [Close by JabaScript (does not work)] button once Modal has been opened by showBootstrapModal method.
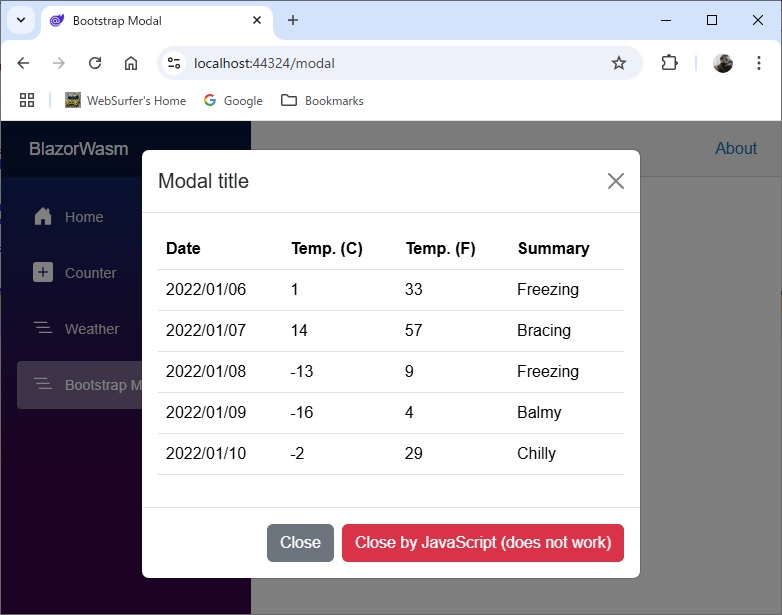