In one of your route constraints, you defined a parameter type as :string. This is not valid see
How to fix "Register the constraint type with 'Microsoft.AspNetCore.Routing.RouteOptions.ConstraintMap"?
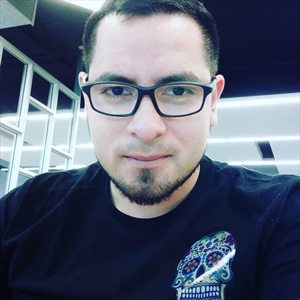
Hello!
I know this question has several posts with multiple answers but in my case I haven't found the solution.
- I'm building a Web Api with NET 8
- My Web Api has swagger
- I'm using Dependency Injection, Service layer, UnityOfWork and Repository pattern
- I'm not using CORS in this moment
So the stack trace pattern to get the data it looks like this:
_myService.GetUsers() => _unityOfWork.UsersRepository.GetAll() => _context.Users.ToList()
In my case, I have only 3 controllers performing simple CRUD operations
In every class I have something like this:
[Produces("application/json")]
[Route("api/[controller]/[action]")]
[ApiController]
public class UsersController : ControllerBase
{
private readonly IUserService _userService;
private readonly IUserTypeService _userTypeService;
private readonly IMapper _mapper;
/*Constructor with injections*/
public UsersController(IUserService userService,
IUserTypeService userTypeService,
IMapper mapper)
{
_userService = userService;
_mapper = mapper;
_userTypeService = userTypeService;
}
[HttpPost]
public IActionResult TestAction([FromBody] UserRegisterDto registeredUserDto)
{
/*---Some code snippet here ---*/
}
}
Swagger is displaying my Controllers and Endpoints as expected
But it doesn't matter what actions I execute, it throws the same error for all the actions; some of them are HttpGet, HttpPost, HttpPatch, etc.
Body request:
Note: All the properties are string types in the backend class (UserRegisterDto.cs)
{
"username": "string",
"name": "string",
"password": "string",
"role": "string"
}
This is my Program.cs file
using Microsoft.AspNetCore.Builder;
using Microsoft.AspNetCore.Hosting;
using Microsoft.Extensions.Configuration;
using Microsoft.Extensions.DependencyInjection;
using Microsoft.Extensions.Hosting;
using System; using System.Reflection;
using Sat.Recruitment.Infrastructure.Extensions;
using Microsoft.AspNetCore.Mvc;
var builder = WebApplication.CreateBuilder(args);
builder.Services.AddOptions(builder.Configuration);
builder.Services.AddDbContexts(builder.Configuration);
builder.Services.AddServices(builder.Configuration);
builder.Services.AddSingleton(builder.Configuration); builder.Services.AddSwagger($"
{Assembly.GetExecutingAssembly().GetName().Name}.xml");
builder.Services.AddAutoMapper(AppDomain.CurrentDomain.GetAssemblies());
builder.Services.AddControllers(options => {
options.CacheProfiles.Add("Default30seconds", new CacheProfile { Duration = 30
}); }).AddNewtonsoftJson();
var app = builder.Build(); // Configure the HTTP request pipeline.
if (app.Environment.IsDevelopment())
{
app.UseDeveloperExceptionPage();
app.UseSwagger();
app.UseSwaggerUI();
}
app.UseHttpsRedirection();
app.UseRouting();
app.UseAuthentication();
app.UseAuthorization();
app.UseEndpoints(endpoints => { _ = endpoints.MapControllers(); });
app.Run();
I don't know what exactly I have to fix. I already checked my dependencies and it seems everything it's ok. I have a breakpoint in the UserController constructor but it's not reached by the request.
Dependencies file
Does anyone have any idea what is happening?
Regards!
-David from México