How to remove Prism boiler plate code
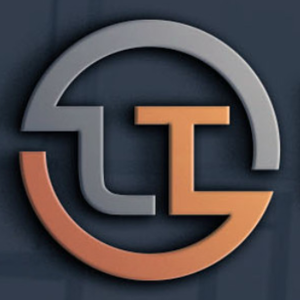
In my app I use both Prism and ReactiveUI for different reasons and it's working really well.
For ReactiveUI there is a package that you can use to remove "boiler plate code":
https://www.reactiveui.net/docs/handbook/view-models/boilerplate-code
For example this:
private string name;
public string Name
{
get => name;
set => this.RaiseAndSetIfChanged(ref name, value);
}
Can be replaced by this:
[Reactive]
public string Name { get; set; }
thanks to that [Reactive]
attribute.
In Prism we also define properties with a few lines of code:
private string name;
public string Name
{
get { return name; }
set { SetProperty(ref name, value); }
}
I was wondering if anybody knows how to make a custom attribute to obtain this result for Prism too, or if there is already something out there:
[Prism]
public string Name { get; set; }
It would be so great to just use an attribute and not have to create private members etc.