Hi,@Ajay Gera.
First, with Document, you could listen to whether the theme has changed and how to get the current Visual Studio theme.
You could switch different themes in WebView2 by using the following code.(Note: The following method could only change the theme of the local web page)
Project Structure
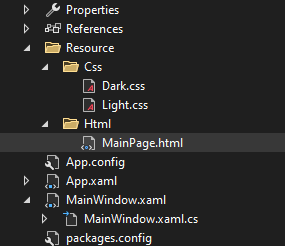
MainWindow.xaml
<Window
xmlns:vm="clr-namespace:Microsoft.Web.WebView2.Wpf;assembly=Microsoft.Web.WebView2.Wpf"
…
>
<Grid>
<Grid.RowDefinitions>
<RowDefinition Height="*" />
<RowDefinition Height="13*" />
</Grid.RowDefinitions>
<Button Content="Change Theme" Click="Button_Click" HorizontalAlignment="Right"></Button>
<vm:WebView2 Grid.Row="1" x:Name="MyWebView" Source="https://www.microsoft.com"></vm:WebView2>
</Grid>
</Window>
MainWindow.xaml.cs
public partial class MainWindow : Window
{
public MainWindow()
{
InitializeComponent();
InitializeWebView();
}
private async void InitializeWebView()
{
MyWebView.NavigationCompleted += WebView_NavigationCompleted;
MyWebView.Source = new Uri("The address of MainPage.html");
}
private void WebView_NavigationCompleted(object sender, Microsoft.Web.WebView2.Core.CoreWebView2NavigationCompletedEventArgs e)
{
ChangeTheme("Dark");
}
bool theme = false;
private void Button_Click(object sender, RoutedEventArgs e)
{
if(theme)
{
ChangeTheme("Dark");
theme = false;
}
else
{
ChangeTheme("Light");
theme = true;
}
}
// Load different css files to change different themes of the web page
private void ChangeTheme(string Theme)
{
string css = string.Empty;
if (Theme == "Dark")
{
css = System.IO.File.ReadAllText("Dark.css address");
}
else if (Theme == "Light")
{
css = System.IO.File.ReadAllText("Light.css address");
}
string script = $"var style = document.createElement('style'); style.innerHTML = `{css}`; document.head.appendChild(style);";
MyWebView.ExecuteScriptAsync(script);
}
}
Refer to the theme of Visual Studio to write CSS to provide themes for your own web pages.
In Visual Studio, you could install Visual Studio Color Theme Designer 2022
through Extensions->Manage Extensions...
Then you could create a VSTheme Project
. Open Custom Theme.vstheme
and select Dark
under Select a base theme
under Quick start
.You could now view how the style of this theme is designed. In addition, you could open Custom Theme.vstheme
through a file editor, which will make it easier to design your css
for different themes.