how to create a link that initiates a method
You will be able to call static web method in the .aspx.cs. See "Calling Static Methods in an ASP.NET Web Page" section in the following Microsoft document:
Exposing Web Services to Client Script
I'm looking for a way to display a link in a grid column, where the the user can click on the link which sends the value (a string) to a method, and does work.
Add the TemplateFiled to the GridView. Put the LinkButton control in the ItemTemplate of TemplateField.
Add the static methods to the .aspx.cs
Set javascript to the OnClientClick property of the LinkButton so that the static method is called on click.
Disable the user friendly url if you use it. Otherwise the static method will not work.
Shown below is a sample:
.aspx.cs
using System;
using System.Web.Services;
namespace WebForms1
{
public partial class GridView : System.Web.UI.Page
{
protected void Page_Load(object sender, EventArgs e)
{
}
[WebMethod]
public static string MyWebMethod(int id)
{
return $"WebMethod called with id={id}";
}
protected string SetOnClientClick(int id)
{
return $"CallWebMthod({id}); return false;";
}
}
}
.aspx
<%@ Page Language="C#" AutoEventWireup="true"
CodeBehind="GridView.aspx.cs"
Inherits="WebForms1.GridView" %>
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml">
<head runat="server">
<meta http-equiv="Content-Type" content="text/html; charset=utf-8"/>
<title></title>
<script src="Scripts/jquery-3.7.0.js"></script>
<script type="text/javascript">
function CallWebMthod(productId) {
$.ajax({
type: "POST",
url: "GridView.aspx/MyWebMethod",
contentType: "application/json; charset=utf-8",
data: `{ "id": ${productId} }`
}).done(response => {
$("#result").empty;
$("#result").text(response.d);
});
}
</script>
</head>
<body>
<form id="form1" runat="server">
<asp:SqlDataSource ID="SqlDataSource1"
runat="server"
ConnectionString="<%$ ConnectionStrings:NORTHWINDConnectionString %>"
SelectCommand="SELECT TOP(10) [ProductID], [ProductName] FROM [Products]">
</asp:SqlDataSource>
<asp:GridView ID="GridView1"
runat="server"
AutoGenerateColumns="False"
DataKeyNames="ProductID"
DataSourceID="SqlDataSource1">
<Columns>
<asp:BoundField DataField="ProductID"
HeaderText="ProductID"
InsertVisible="False"
ReadOnly="True"
SortExpression="ProductID" />
<asp:BoundField DataField="ProductName"
HeaderText="ProductName"
SortExpression="ProductName" />
<asp:TemplateField>
<ItemTemplate>
<asp:LinkButton ID="LinkButton1"
runat="server"
OnClientClick='<%# SetOnClientClick((int)Eval("ProductID")) %>'>
LinkButton
</asp:LinkButton>
</ItemTemplate>
</asp:TemplateField>
</Columns>
</asp:GridView>
<div id="result"></div>
</form>
</body>
</html>
Result:
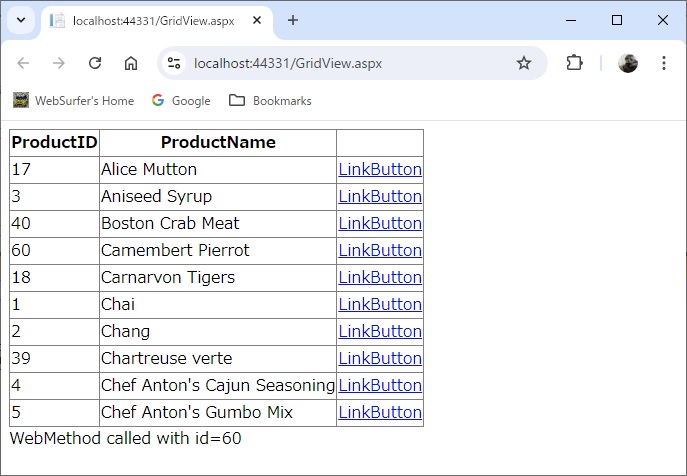