I have two classes
public class Turbine {
public int Id { get; set; }
public string? Name { get; set; }
public string? Label { get; set; }
public string? Address { get; set; }
public Location? Location { get; set; }
public ObservableCollection<string>? Images { get; set; }
public ObservableCollection<TurbineData>? DataCollection { get; set; }
}
}
public class TurbinePin {
public ICommand? PinClickedCommand { get; set; }
public Turbine? Turbine { get; set; }
}
I also created a custom control, since the map doesn't have a command for the pins
namespace METROWIND.Controls {
public class CustomMapPin : Pin {
public static readonly BindableProperty MarkerClickedCommandProperty =
BindableProperty.Create(
nameof(MarkerClickedCommand),
typeof(ICommand),
typeof(CustomMapPin),
null);
public ICommand? MarkerClickedCommand {
get => (ICommand)GetValue(MarkerClickedCommandProperty);
set => SetValue(MarkerClickedCommandProperty, value);
}
public static readonly BindableProperty CustomPinLabelProperty = BindableProperty.Create(
nameof(CustomPinLabel), typeof(string), typeof(CustomMapPin));
public string CustomPinLabel {
get => (string)GetValue(CustomPinLabelProperty);
set => SetValue(CustomPinLabelProperty, value);
}
public static readonly BindableProperty CustomPinAddressProperty = BindableProperty.Create(
nameof(CustomPinAddress), typeof(string), typeof(CustomMapPin));
public string CustomPinAddress {
get => (string)GetValue(CustomPinAddressProperty);
set => SetValue(CustomPinAddressProperty, value);
}
public static readonly BindableProperty TurbineImagesProperty = BindableProperty.Create(
nameof(TurbineImages),
typeof(IEnumerable),
typeof(CustomMapPin));
public IEnumerable TurbineImages {
get => (IEnumerable)GetValue(TurbineImagesProperty);
set => SetValue(TurbineImagesProperty, value);
}
public static readonly BindableProperty ChartDataProperty = BindableProperty.Create(
nameof(ChartData), typeof(IEnumerable),
typeof(CustomMapPin));
public IEnumerable ChartData {
get => (IEnumerable)GetValue(ChartDataProperty);
set => SetValue(ChartDataProperty, value);
}
public static readonly BindableProperty TurbineProperty = BindableProperty.Create(
nameof(Turbine), typeof(Turbine), typeof(CustomMapPin));
public Turbine Turbine {
get => (Turbine)GetValue(TurbineProperty);
set => SetValue(TurbineProperty, value);
}
public CustomMapPin() {
MarkerClicked += CustomPin_MarkerClicked;
}
private void CustomPin_MarkerClicked(object? sender, PinClickedEventArgs e) {
// Ensure info window is shown
e.HideInfoWindow = true;
// Execute command if any
if (MarkerClickedCommand?.CanExecute(this) == true) {
MarkerClickedCommand.Execute(this);
}
}
}
}
and I have my vm
public ChargingStationsMapPageViewModel() {
OnPinMarkerClickedCommand = new Command<object>(OnPinMarkerClicked);
Turbines = TurbinesService.GetTurbinePins(OnPinMarkerClickedCommand);
}
[RelayCommand]
private void Appearing(Microsoft.Maui.Controls.Maps.Map map) {
if (map != null) {
MapView = map;
}
}
[RelayCommand]
void ItemSelected(Turbine Turbine) {
var mapSpan = MapSpan.FromCenterAndRadius(
Turbine.Location!,
Distance.FromKilometers(0.4));
MapView!.MoveToRegion(mapSpan);
IsExpanded = false;
}
void OnPinMarkerClicked(object turbine) {
if (turbine != null) {
// Handle the pin click event
Shell.Current.GoToAsync($"{nameof(TurbineDetailPage)}",
true,
new Dictionary<string, object> {
{ "SelectedTurbine", turbine }
});
};
}
[RelayCommand]
void OpenMenu() {
IsOptionsOpen = true;
}
[RelayCommand]
void ChangeMapType(int mapType) {
if (mapType == 0) {
MapView!.MapType = MapType.Street;
} else {
MapView!.MapType = MapType.Satellite;
}
IsOptionsOpen = false;
}
}
}
<maps:Map
x:Name="ChargingStationMap"
ItemsSource="{Binding Turbines}"
VerticalOptions="FillAndExpand">
<maps:Map.ItemTemplate>
<DataTemplate x:DataType="model:TurbinePin">
<controls:CustomMapPin
Address="{Binding Turbine.Address}"
ChartData="{Binding Turbine.DataCollection}"
CommandParameter="{Binding Turbine}"
Label="{Binding Turbine.Label}"
Location="{Binding Turbine.Location}"
MarkerClickedCommand="{Binding PinClickedCommand}"
Turbine="{Binding Turbine}"
TurbineImages="{Binding Turbine.Images}" />
</DataTemplate>
</maps:Map.ItemTemplate>
</maps:Map>
but when I click a pin, I am trying to receive the Turbine object
but I am getting the
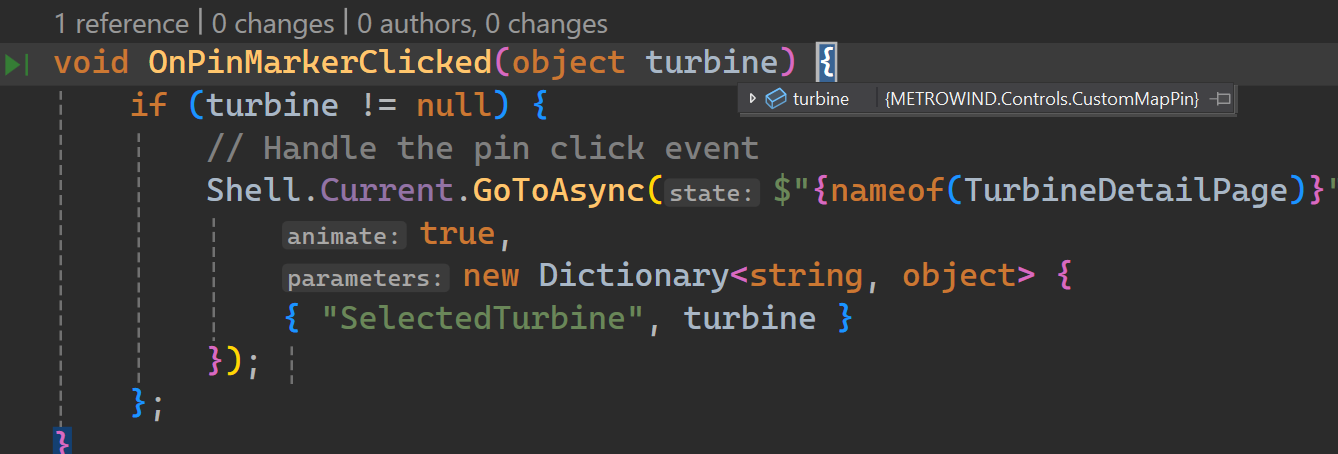
the customMap
I already tried to change my command to <Turbine> but is always the same (I just put object, to make sure it was hitting the breakpoint, because command are picky and if you do not send what they want they do not work)