The MaxProficiencies property's Setter does not always fire, even when I change the value. Why is it doing that?
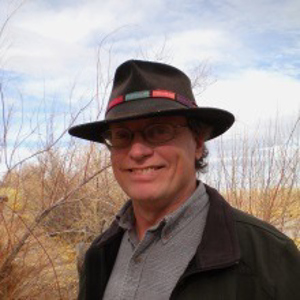
Rod At Work
866
Reputation points
We're using MVVM Light. Here's the MaxProficiencies definition:
public int? MaxProficiencies
{
get
{
if (Solution != null)
{
return Solution.MaxProficiencies;
}
IsMaxProficienciesCompleted = false;
return 0;
}
set
{
if (value == null)
{
IsMaxProficienciesCompleted = false;
}
else
{
var tmpMaxProfs = value.Value;
Solution.MaxProficiencies = tmpMaxProfs;
solutionDirty = true;
AvailProficiencies = CalculateAvailableProficiencies();
if (value > 0)
{
IsMaxProficienciesCompleted = true;
}
else
{
IsMaxProficienciesCompleted = false;
}
}
RaisePropertyChanged();
}
}
I have a strong suspicious that this validation rule has a lot to do with the Max Proficiencies Setter not firing the second time Max Proficiencies is changed:
public class MaxProficienciesValidationRule : ValidationRule
{
/// <summary>
/// The minimum integer value that MaxProficiences can be
/// </summary>
public long Min { get; set; }
/// <summary>
/// The value of the current Solution ID
/// </summary>
public long SolutionID { get; set; }
public override ValidationResult Validate(object value, CultureInfo cultureInfo)
{
if (value == null)
{
return new ValidationResult(false, "Empty string invalid");
}
if (SolutionID > 0)
{
//this is an existing Solution, so it must have been previously validated
return new ValidationResult(true, "");
}
long proposedMaxProficiencies;
try
{
if (((string)value).Length > 0)
{
proposedMaxProficiencies = Int64.Parse((string)value);
}
else
{
return new ValidationResult(false, "Empty string invalid");
}
}
catch (Exception ex)
{
return new ValidationResult(false, $"Illegal character(s) or {ex.Message}");
}
if (proposedMaxProficiencies < Min)
{
return new ValidationResult(false, $"MaxProficiencies must be greater than or equal to {Min}");
}
return new ValidationResult(true, "");
}
public MaxProficienciesValidationRuleWrapper Wrapper { get; set; }
}
And finally, here's the code snippet from the ViewModel, where I've defined the MaxProficiencies property. I've placed a breakpoint at the start of the Set. If I enter in a value, say 1. It fires. However, if I clear the value, from that point onward it no longer goes to the Set, even if I put in 0, or 1, or 10. It just stops every being set again.
public int? MaxProficiencies
{
get
{
if (Solution != null)
{
return Solution.MaxProficiencies;
}
IsMaxProficienciesCompleted = false;
return 0;
}
set
{
if (value == null)
{
IsMaxProficienciesCompleted = false;
}
else
{
var tmpMaxProfs = value.Value;
Solution.MaxProficiencies = tmpMaxProfs;
solutionDirty = true;
AvailProficiencies = CalculateAvailableProficiencies();
if (value > 0)
{
IsMaxProficienciesCompleted = true;
}
else
{
IsMaxProficienciesCompleted = false;
}
}
RaisePropertyChanged();
}
}