as they are separate requests, the the detail request would need to rebuild the list. you would need to add the required parameters to the detail request (page number, page size).
.net 6 Core MVC Paging for assigned data
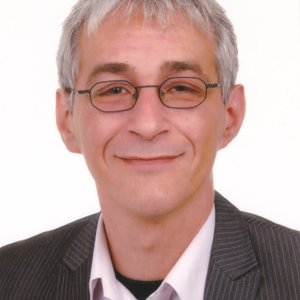
I am trying to build an application like in the tutorial in Microsoft: https://learn.microsoft.com/en-us/aspnet/core/data/ef-mvc/sort-filter-page?view=aspnetcore-6.0
So i am using the PaginatedList.cs as helper:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Threading.Tasks;
using Microsoft.EntityFrameworkCore;
namespace ContosoUniversity
{
public class PaginatedList<T> : List<T>
{
public int PageIndex { get; private set; }
public int TotalPages { get; private set; }
public PaginatedList(List<T> items, int count, int pageIndex, int pageSize)
{
PageIndex = pageIndex;
TotalPages = (int)Math.Ceiling(count / (double)pageSize);
this.AddRange(items);
}
public bool HasPreviousPage => PageIndex > 1;
public bool HasNextPage => PageIndex < TotalPages;
public static async Task<PaginatedList<T>> CreateAsync(IQueryable<T> source, int pageIndex, int pageSize)
{
var count = await source.CountAsync();
var items = await source.Skip((pageIndex - 1) * pageSize).Take(pageSize).ToListAsync();
return new PaginatedList<T>(items, count, pageIndex, pageSize);
}
}
}
and it runs fine for the Index.
In the Detail i would like to have the fields and a pagnated list from the assigned data:
public async Task<IActionResult> Details(int? id, int? seiteBL)
{
if (id == null || _context.Länder == null)
{
return NotFound();
}
var land = await _context.Länder
.AsNoTracking()
.Include(a => a.Bundesländer.Where(s => s.Aktiv.Equals(true)))
.FirstOrDefaultAsync(m => m.Id == id);
if (land == null)
{
return NotFound();
}
var pageSizeBL = Configuration.GetValue("PageSize", 16);
double AnzahlTrefferBL = land.Bundesländer.Count();
int? aktuelleSeiteBL = seiteBL;
if (aktuelleSeiteBL == null)
{
aktuelleSeiteBL = 1;
}
ViewData["AnzahlTrefferBL"] = land.Bundesländer.Count();
ViewData["AnzahlSeitenBL"] = (int)Math.Ceiling(AnzahlTrefferBL / pageSizeBL);
ViewData["AktuelleSeiteBL"] = aktuelleSeiteBL;
//how do i get the paging of land.Bundesländer by using the PaginatedList?
return View(land);
}
how do i get the paging of land.Bundesländer by using the PaginatedList?
Developer technologies | ASP.NET | ASP.NET Core
Developer technologies | C#
2 answers
Sort by: Most helpful
-
Bruce (SqlWork.com) 77,686 Reputation points Volunteer Moderator
2022-09-14T15:23:38.27+00:00 -
Anonymous
2022-09-15T07:26:31.31+00:00 Hi,@Anton Bröckel
if you checked the codes:public static async Task<PaginatedList<T>> CreateAsync(IQueryable<T> source, int pageIndex, int pageSize) { var count = await source.CountAsync(); var items = await source.Skip((pageIndex - 1) * pageSize).Take(pageSize).ToListAsync(); return new PaginatedList<T>(items, count, pageIndex, pageSize); }
you'll find you have to pass the value of pageIndex and pagesize to this method for paging;
If you want to assign the value of pageIndex and pagesize in your Index page and pass them to your controller.
you could add them in the query withasp-route-pageIndex=""
andasp-route-page-size=""
the uri looks as below now:
and you could reveive the arguements in DetailsController as below:public async Task<IActionResult> Details(int? id,int PageIndex,int Pagesize //other arguements) { ......... }
You could check this document for more details of Modelbinding
----------
If the answer is the right solution, please click "Accept Answer" and kindly upvote it. If you have extra questions about this answer, please click "Comment".
Note: Please follow the steps in our documentation to enable e-mail notifications if you want to receive the related email notification for this thread.Best regards,
RuikaiFeng