Try executing Entities.ClearItems( ) before your entities.ForEach(...) line.
How to clear the DataGrid table when new data is added.
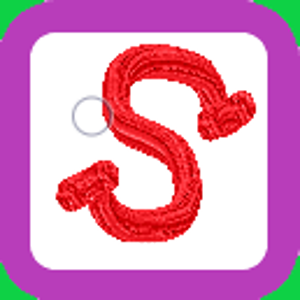
The main problem is related to clearing the existing table when adding new data from the SQL table. In my project, there is a table in which data is loaded when a button is pressed, the handler looks like this:
public sealed partial class OutputPages : Page, INotifyPropertyChanged
{
...
private string connectionString;
public event PropertyChangedEventHandler PropertyChanged;
private void OnPropertyChanged(string propertyName) =>
PropertyChanged?.Invoke(this, new PropertyChangedEventArgs(propertyName));
private ObservableCollection<Entity> _entities;
public ObservableCollection<Entity> Entities
{
...
}
public OutputPages()
{
this.InitializeComponent();
Entities = new ObservableCollection<Entity>();
}
private async void Button_Cl_Get_Date_Output_Page(object sender, RoutedEventArgs e)
{
try
{
var entities = await GetDateGrid_SQL();
entities.ForEach(o => Entities.Add(o));
}
catch(Exception ex)
{
...
}
}
...
public async Task<List<Entity>> GetDateGrid_SQL()
{
try
{
connectionString = @"...;";
var query = "SELECT * FROM MyTable_Name;";
var entitys = new List<Entity>();
using (var connections = new SqlConnection(connectionString))
{
await connections.OpenAsync();
using (SqlCommand command = new SqlCommand(query, connections))
{
using (SqlDataReader reader = await command.ExecuteReaderAsync())
{
while (await reader.ReadAsync())
{
var entity = new Entity
{
ID = reader.GetInt32(0)
};
if (!await reader.IsDBNullAsync(1))
entity.Name = reader.GetString(1);
if (!await reader.IsDBNullAsync(2))
entity.Designation = reader.GetString(2);
....
entitys.Add(entity);
}
}
}
}
return entitys;
}
catch (Exception ex)
{
throw;
}
}
}
In the example above, there is the GetDateGrid_SQL method - we get data in it, then this data is sent to the DataGrid table in the Button_Cl_Get_Date_Output_Page method. The problem is that when you click the button with the Button_Cl_Get_Date_Output_Page handler again, the data is added over the old data. That is, if the first click added three existing records, then the second click adds 3 more, but the old data is not deleted). Looked for built-in delete tools according to the DataGrid API, but nothing found yet, no delete or update tools. For DataGridView one could use
dataGridView.DataSource=null;
dataGridView.Rows.Clear();
but it doesn't work with this control.