Hi @Pradyut Sinha
My thoughts are:
- Declare classes and lists.
- Assign a value to the data.
- Generate JSON variables.
- Print it out
For demonstration purposes, I printed the JSON data as string data.
In order to keep the same format as JSON, I set an option.
var options = new JsonSerializerOptions { WriteIndented = true };
string jsonString = System.Text.Json.JsonSerializer.Serialize(outputdata, options);
Of course, you can also print it directly.
You can refer to the following code:
public class Information
{
public string atodatadotcontext { get; set; }
public Value[] value { get; set; }
}
public class Value
{
public string id { get; set; }
public string role { get; set; }
public GrantedTov2 grantedToV2 { get; set; }
public GrantedTo grantedTo { get; set; }
}
public class GrantedTov2
{
public User user { get; set; }
public SiteUser siteUser { get; set; }
}
public class User
{
public string atodatadottype { get; set; }
public string displayName { get; set; }
public string email { get; set; }
public string id { get; set; }
}
public class SiteUser
{
public string displayName { get; set; }
public string email { get; set; }
public string id { get; set; }
public string loginName { get; set; }
}
public class GrantedTo
{
public User1 user1 { get; set; }
}
public class User1
{
public string displayName { get; set; }
public string email { get; set; }
public string id { get; set; }
}
internal class Program
{
static void Main(string[] args)
{
var user1_1 = new User1
{
displayName = "Banerjee,Alapan[JJCUS NON - J & J]",
email= "ABaner62@ITS.JNJ.com",
id= "09748e1f-07c7-4b76-b239-2842882b9294"
};
var user1_2 = new User1
{
displayName = "Yousuf, Adnan [JJCUS NON-J&J]",
email= "AYousuf@ITS.JNJ.com",
id= "b4c566a9-610e-41cc-be33-0aa7ca15cfcb"
};
var user1_3 = new User1
{
displayName = "SA-PRD-oddev2-admin",
email = "SA-PRD-oddev2-admin@its.jnj.com",
id = "31964c3b-f2ed-455d-8dc7-5b12e8c6be46"
};
var user1 = new User
{
atodatadottype = "#microsoft.graph.sharePointIdentity",
displayName = "Banerjee, Alapan [JJCUS NON-J&J]",
email = "ABaner62@ITS.JNJ.com",
id = "09748e1f-07c7-4b76-b239-2842882b9294"
};
var user2 = new User
{
atodatadottype = "#microsoft.graph.sharePointIdentity",
displayName = "Yousuf, Adnan [JJCUS NON-J&J]",
email = "AYousuf@ITS.JNJ.com",
id = "b4c566a9-610e-41cc-be33-0aa7ca15cfcb"
};
var user3 = new User
{
atodatadottype = "#microsoft.graph.sharePointIdentity",
displayName = "SA-PRD-oddev2-admin",
email = "SA-PRD-oddev2-admin@its.jnj.com",
id = "31964c3b-f2ed-455d-8dc7-5b12e8c6be46"
};
var siteuser1 = new SiteUser
{
displayName= "Banerjee, Alapan [JJCUS NON-J&J]",
email = "ABaner62@ITS.JNJ.com",
id="21",
loginName= "i:0#.f|membership|abaner62@its.jnj.com"
};
var siteuser2 = new SiteUser
{
displayName = "Yousuf, Adnan [JJCUS NON-J&J]",
email = "AYousuf@ITS.JNJ.com",
id = "46",
loginName = "i:0#.f|membership|ayousuf@its.jnj.com"
};
var siteuser3 = new SiteUser
{
displayName = "SA-PRD-oddev2-admin",
email = "SA-PRD-oddev2-admin@its.jnj.com",
id = "3",
loginName = "i:0#.f|membership|sa-prd-oddev2-admin@its.jnj.com"
};
var grantedto1 = new GrantedTo
{
user1 = user1_1
};
var grantedto2 = new GrantedTo
{
user1 = user1_2
};
var grantedto3 = new GrantedTo
{
user1 = user1_3
};
var grantedtov2_1 = new GrantedTov2
{
user=user1,
siteUser=siteuser1
};
var grantedtov2_2 = new GrantedTov2
{
user = user2,
siteUser = siteuser2
};
var grantedtov2_3 = new GrantedTov2
{
user = user3,
siteUser = siteuser3
};
Value[] value1 = new Value[3];
value1[0] = new Value
{
id = "aTowIy5mfG1lbWJlcnNoaXB8YWJhbmVyNjJAaXRzLmpuai5jb20",
role = "[read]",
grantedToV2 = grantedtov2_1,
grantedTo=grantedto1
};
value1[1] = new Value
{
id = "aTowIy5mfG1lbWJlcnNoaXB8YXlvdXN1ZkBpdHMuam5qLmNvbQ",
role = "[owner]",
grantedToV2 = grantedtov2_2,
grantedTo = grantedto2
};
value1[2] = new Value
{
id = "aTowIy5mfG1lbWJlcnNoaXB8c2EtcHJkLW9kZGV2Mi1hZG1pbkBpdHMuam5qLmNvbQ",
role = "[owner]",
grantedToV2 = grantedtov2_2,
grantedTo = grantedto2
};
var inforamtion = new Information
{
atodatadotcontext = "https://graph.microsoft.com/v1.0/$metadata#users('31964c3b-f2ed-455d-8dc7-5b12e8c6be46')/drive/items('01FIJ7336ZHF5XAVMMUFCYDXFHXZRICATY')/permissions",
value=value1
};
var options = new JsonSerializerOptions { WriteIndented = true };
string jsonString = JsonSerializer.Serialize(inforamtion, options);
Console.WriteLine(jsonString);
Console.Read();
}
}
Result: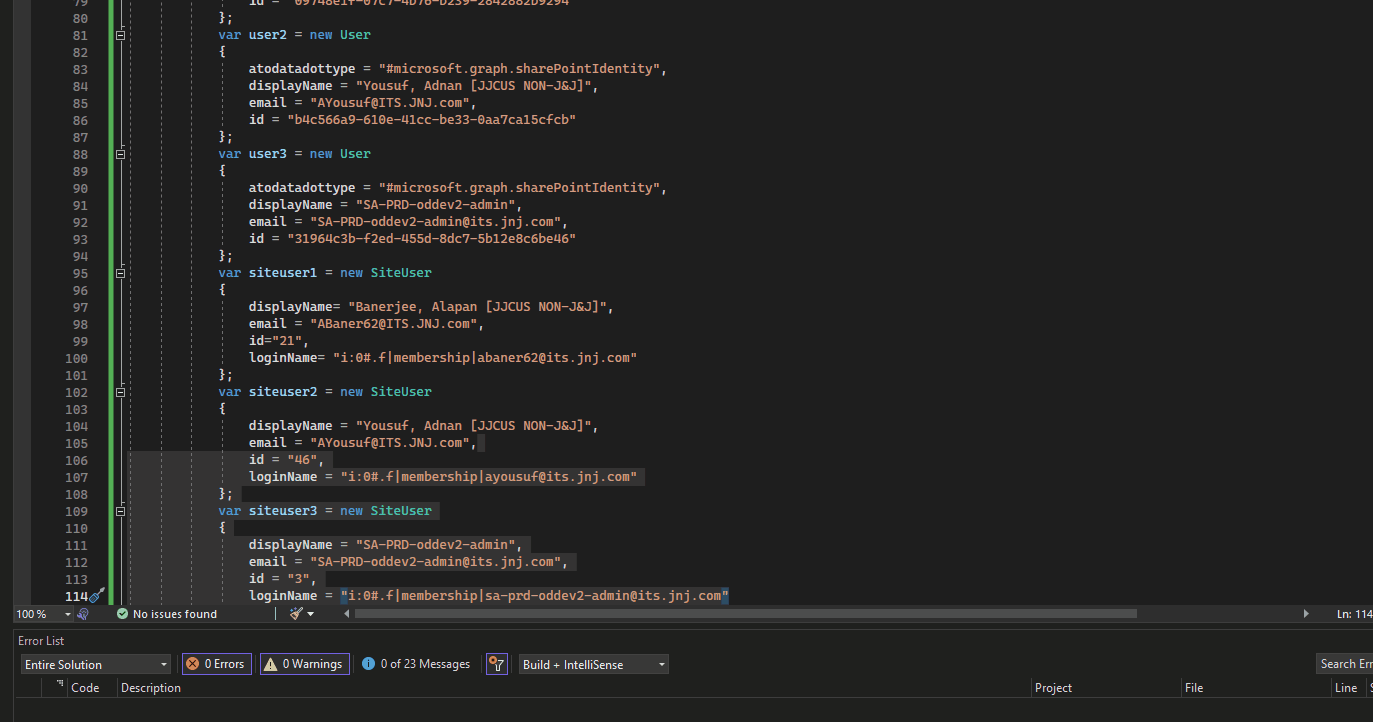
Best Regards
Qi You
If the answer is the right solution, please click "Accept Answer" and kindly upvote it. If you have extra questions about this answer, please click "Comment".
Note: Please follow the steps in our documentation to enable e-mail notifications if you want to receive the related email notification for this thread.