EF Core query for a specific condition in related entities and update parent entity
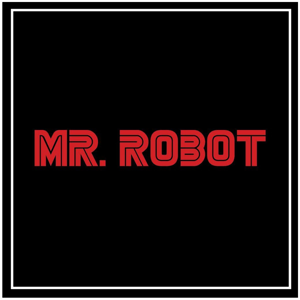
Cenk
956
Reputation points
Hello there,
In my Blazor Server application, I have Order and Order Details entities which are related as you can imagine. They both have their own repositories.
public class Order
{
public int Id { get; set; }
[Required]
public DateTime OrderDateTime { get; set; }
[Required]
[MaxLength(250)]
public int CustomerId { get; set; }
public string Status { get; set; }
[MaxLength(50)]
public string DoneBy { get; set; }
public List<OrderDetail> OrderDetails { get; set; }
public Customer Customer { get; set; }
}
public class OrderDetail
{
public int Id { get; set; }
[Required]
[MaxLength(100)]
public string ProductCode { get; set; }
[MaxLength(250)]
public string? ProductName { get; set; }
[Required]
public int Quantity { get; set; }
[Required]
public double BuyUnitPrice { get; set; }
public double CostRatio { get; set; }
public double UnitCost { get; set; }
public double TotalBuyPrice { get; set; }
public double? SellUnitPrice { get; set; }
public double? TotalSellPrice { get; set; }
[MaxLength(150)]
public string? ShippingNumber { get; set; }
public string? Status { get; set; }
[MaxLength(150)]
public string? TrackingNumber { get; set; }
[MaxLength(400)]
public string? Description { get; set; }
public string? Currency { get; set; }
public string? CustomerStockCode { get; set; }
public string? CustomerOrderNumber { get; set; }
public int IsActive { get; set; }
public double? TotalUnitCost { get; set; }
public int OrderId { get; set; }
public int VendorId { get; set; }
public string? Warehouse { get; set; }
public string? PaymentStatus { get; set; }
public Order Order { get; set; }
public Vendor Vendor { get; set; }
[DataType(DataType.DateTime)]
public DateTime? CompletionDateTime { get; set; }
}
I would like to update the Order entity status = Completed, only if all of the related Order Detail statuses are Completed. Let's say, order id = 1, and if all of the Order Details statuses = Completed which are associated with Order = 1.
How can I achieve this?
Thank you.