Why changing a form graphic property will affect the software?
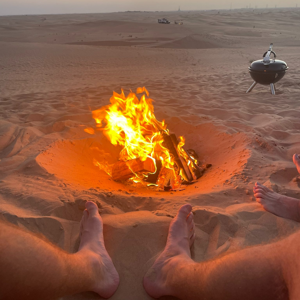
Description:
I create a VB.NET Windows Forms application [.net6+] that loads an HTML file using the HtmlAgilityPack library, extracts data from it, and displays it in a list box.
The form contains two combo boxes and a button. The first combo box allows the user to select a type of resource (marble, crystal, sulfur, or wine) and the second combo box allows the user to select a sorting parameter (mine level, carpentry level, or total level). When the user clicks the button, the application extracts data from the HTML document and displays it in the list box based on the user's selections. The class Island is defined as a container for the extracted data, which includes the island's coordinates, mine level, carpentry level, number of free slots, and total level (the sum of the mine and carpentry levels).
The code loads an HTML file ("pangaia2.html") and sets a label's text to the file name without the extension. It then determines the selected resource type and finds all island tiles in the HTML document that produce that type of resource. For each island tile, it checks if it produces the selected resource type, extracts the mine level and carpentry level, and calculates the number of free slots. If the island meets certain criteria (less than or equal to 7 occupied slots), it creates an Island object and adds it to a list. The list of Island objects is then sorted based on the selected sorting parameter, and the results are printed to the list box. Each entry in the list box displays the island's coordinates, mine level, carpentry level, and number of free slots.
The code is full working and fully tested.
Imports System.IO
Imports HtmlAgilityPack
Public Class Form1
Public Class Island
Public Property Coord As String
Public Property MineLevel As Integer
Public Property CarpentryLevel As Integer
Public Property FreeSlots As Integer
Public Property TotalLevel As Integer
End Class
Private Sub Button1_Click(sender As Object, e As EventArgs) Handles Button1.Click
ListBox1.Items.Clear()
Dim filePath As String = "pangaia2.html"
Dim fileName As String = Path.GetFileNameWithoutExtension(filePath)
Dim htmlDoc As New HtmlAgilityPack.HtmlDocument()
htmlDoc.Load(filePath)
' Set the label text to the file name without the extension
Label3.Text = fileName
' Ottieni il tipo di risorsa selezionato dall'utente
Dim selectedResourceType As String = ComboBox1.SelectedItem.ToString()
' Determina la classe CSS corrispondente alla risorsa selezionata
Dim resourceClass As String = ""
Select Case selectedResourceType
Case "Marmo"
resourceClass = "tradegood2"
Case "Cristallo"
resourceClass = "tradegood3"
Case "Zolfo"
resourceClass = "tradegood4"
Case "Vino"
resourceClass = "tradegood1"
End Select
' Ottieni tutti gli elementi islandTile che hanno il tipo di risorsa selezionato dall'utente
Dim islands As New List(Of Island)()
For Each tile As HtmlNode In htmlDoc.DocumentNode.SelectNodes($"//div[contains(@class, 'islandTile') and not(contains(@class, 'oceanTile')) and starts-with(@id, 'tile_')]")
' Check if this island produces the specific resource you're interested in
Dim tradeGoodType As String = tile.SelectSingleNode($".//div[contains(@class, 'tradegood')]/@class")?.GetAttributeValue("class", "")
If tradeGoodType <> $"tradegood {resourceClass}" Then
Continue For
End If
' Get the levels of mine and carpentry
Dim mineLevel As Integer
Integer.TryParse(tile.SelectSingleNode($".//div[contains(@class, '{resourceClass}')]/span")?.InnerText, mineLevel)
Dim carpentryLevel As Integer
Integer.TryParse(tile.SelectSingleNode($".//div[contains(@class, 'ikaeasy-resource-wood')]/span")?.InnerText, carpentryLevel)
' Get the number of occupied slots
Dim occupiedSlots As Integer
Integer.TryParse(tile.SelectSingleNode($".//div[contains(@class, 'cities')]/text()")?.InnerText, occupiedSlots)
Dim totalLevel As Integer = mineLevel + carpentryLevel
' Check if the island meets the criteria and add it to the list
If occupiedSlots <= 7 Then
Dim island As New Island With {
.Coord = tile.GetAttributeValue("title", ""),
.MineLevel = mineLevel,
.CarpentryLevel = carpentryLevel,
.FreeSlots = 16 - occupiedSlots,
.TotalLevel = totalLevel
}
islands.Add(island)
End If
Next
' Sort the islands by the selected parameter
Dim selectedSortParameter As String = ComboBox2.SelectedItem.ToString()
Select Case selectedSortParameter
Case "Miniera"
islands = islands.OrderByDescending(Function(i) i.MineLevel).ToList()
Case "Carpenteria"
islands = islands.OrderByDescending(Function(i) i.CarpentryLevel).ToList()
Case "Totale"
islands = islands.OrderByDescending(Function(i) i.TotalLevel).ToList()
End Select
' Print the results
For Each island In islands
ListBox1.Items.Add("Isola " & island.Coord & " | " & "Miniera: lvl " & island.MineLevel & " | " & " Falegnameria: lvl " & island.CarpentryLevel & " | " & " Posti Liberi: " & island.FreeSlots)
Next
End Sub
Issue:
Whenever Im changing a little thing in the form gui, during debug the software doesn't work anymore. I've been trying to look online, but to be honest, I couldn't find a reason to it. I registered a gif where I show the correct functioning, then Is enough to change the property FormBorderStyle to make the software to not work anymore.To make it work again, I need to undo the operation. This issue occur also by simply moving a label off some pixels. It shows no warnings/pop up/ messages or stack traces.
I did debug it and used as many breakpoints as i could in both situations, and in both situations I can see that the value are passed through, so the software does what is it built for. It just doesn't work as soon as i change something in the gui. Any hint or suggestion