Hi,@Nick R
I tried with a minimal example as below:
CardService:
public interface ICardService
{
void Initialize(List<int> innitialcards);
int GetCardId(int UserId);
}
public class CardService : ICardService
{
private List<int> Cards { get; set; }=new List<int>(){};
private Dictionary<int, int> User_Cards { get; set; }=new Dictionary<int, int>(){};
public int GetCardId(int UserId)
{
if (User_Cards.ContainsKey(UserId))
{
return User_Cards[UserId];
}
if (Cards.Count==0)
{
return 0;
}
var itemunm=Random.Shared.Next(0, Cards.Count);
var result=Cards[itemunm];
User_Cards.Add(UserId,Cards[itemunm]);
Cards.Remove(Cards[itemunm]);
return result;
}
public void Initialize(List<int> innitialcards)
{
Cards = innitialcards;
User_Cards.Clear();
}
}
Regist the service:(it's important to regist the service with Singleton,you could check the document related with servicelifetime)
builder.Services.AddSingleton<ICardService, CardService>();
Controller:
public class CardsController : ControllerBase
{
private readonly ICardService _cardService;
public CardsController(ICardService cardService)
{
_cardService = cardService;
}
[HttpGet]
public string Get(int UserId)
{
var cardId=_cardService.GetCardId(UserId);
if (cardId == 0)
{
return "no cards now";
}
return cardId.ToString();
}
[HttpPost]
public void InnitializeCards([FromBody] List<int> Cards)
{
_cardService.Initialize(Cards);
}
}
Result:
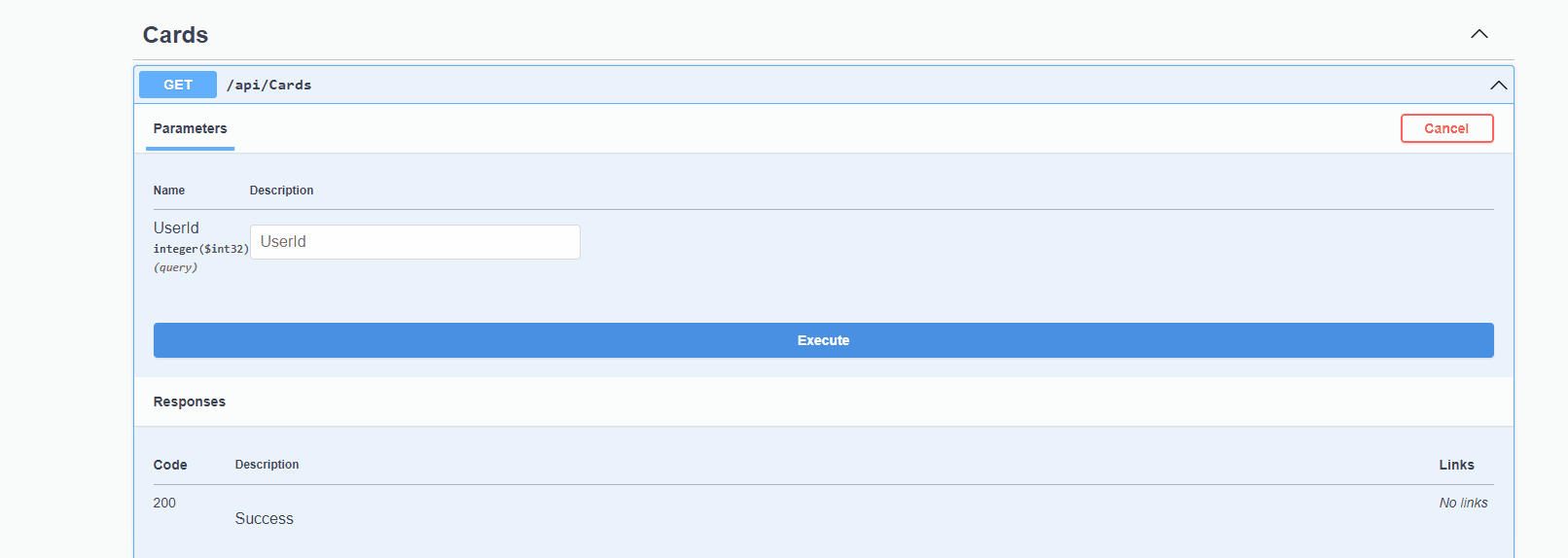
If the answer is the right solution, please click "Accept Answer" and kindly upvote it. If you have extra questions about this answer, please click "Comment".
Note: Please follow the steps in our documentation to enable e-mail notifications if you want to receive the related email notification for this thread.
Best regards,
Ruikai Feng