The button "Launch Demo Modal" is displayed, however a dialog is not produced.
What am I doing wrong, or need to add to my project?
Modal requires JavaScript reference within the project which usually doesn't linked by default. More specifically, within Blazor app in _Layout.cshtml
we need to include that reference to render modal by ourself.
Solution:
This issue can be resolve by two ways as following:
Way: 1:
One is by using internal JavaScript file references installing script file from NuGet package or manually adding script file and finally link within _Layout.cshtml
as following:

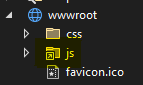
<script src="js\bootstrap.js"></script>
Way: 2:
Another way can be by using CDN reference. In this scenario you could include within your _Layout.cshtml` on `<head>
section as following:
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.4.1/css/bootstrap.min.css">
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.6.3/jquery.min.js"></script>
<script src="https://maxcdn.bootstrapcdn.com/bootstrap/3.4.1/js/bootstrap.min.js"></script>
Full Reference:
<head>
<meta charset="utf-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<base href="~/" />
<link rel="stylesheet" href="css/bootstrap/bootstrap.min.css" />
<link href="css/site.css" rel="stylesheet" />
<link href="BlazorServerApp.styles.css" rel="stylesheet" />
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.4.1/css/bootstrap.min.css">
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.6.3/jquery.min.js"></script>
<script src="https://maxcdn.bootstrapcdn.com/bootstrap/3.4.1/js/bootstrap.min.js"></script>
<component type="typeof(HeadOutlet)" render-mode="ServerPrerendered" />
</head>
Output:
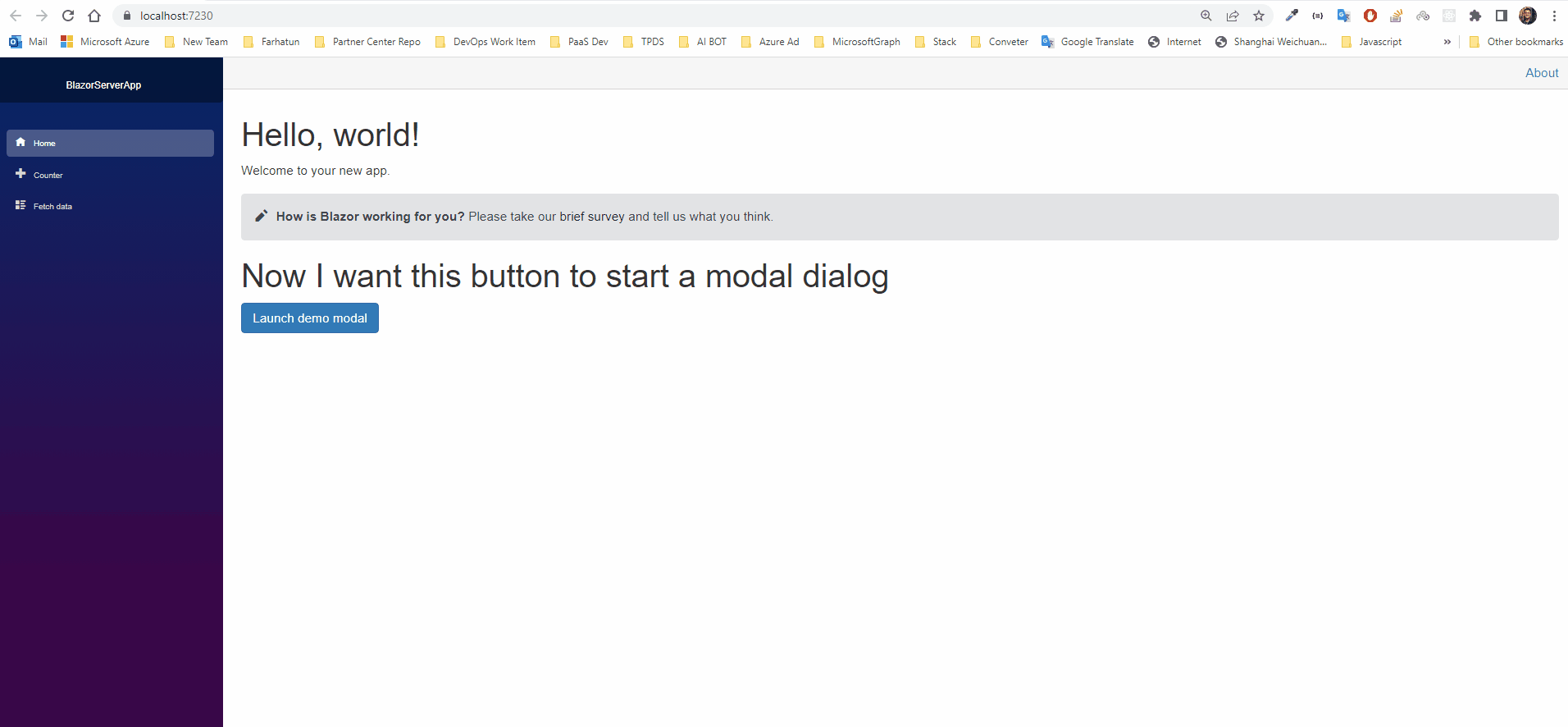
Note:
Make sure you don't have any script error while loading the page or clicking on the modal dialog. Please check following error and ensure you have resolve that.
