A community member has associated this post with a similar question:
Redirecting or showing message alert box when using parameterized query with DataReader
Only moderators can edit this content.
Redirecting or showing message alert box when using parameterized query with DataReader
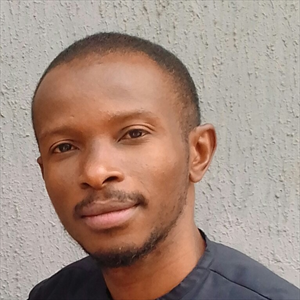
I had this code that displays record from database using DataAdapter, and I made it in such a way that when any user tries to go to the page directly by inserting the page address directly in the address bar of the browser, it will show a message box telling the user that the Session has timed out.
But in order to avoid SQL injection attack, I changed the display and decided to use DataReader and parameterized query. After I changed and used parameterized query with DataReader, I tried to navigate directly to the page by right clicking the page and viewing through the browser then I got this error:
Server Error in '/' Application.
Error:The parameterized query '(@passname nvarchar(4000))SELECT * FROM DetailTable
WHERE passname' expects the parameter '@passname', which was not supplied.
This is the initial code I had, and it was working but I have to change it and use parameterized query, because of an Sql injection attack
public void Details()
{
try
{
if (Session["index"] != null)
{
string connectionString = ConfigurationManager.ConnectionStrings["ConString"].ConnectionString;
using (SqlConnection con = new SqlConnection(connectionString))
{
con.Open();
SqlCommand cmd = new SqlCommand
{
CommandText = "SELECT * FROM DetailTable WHERE passname = '" + Session["index"] + "'",
Connection = con
};
SqlDataAdapter sda = new SqlDataAdapter();
DataSet ds = new DataSet();
sda.SelectCommand = cmd;
sda.Fill(ds, "detail");
if (ds.Tables[0].Rows.Count > 0)
{
orgName.Text = ds.Tables[0].Rows[0]["Name"].ToString();
title.Text = ds.Tables[0].Rows[0]["passname"].ToString();
datelbl.Text = ds.Tables[0].Rows[0]["Dated"].ToString();
timelbl.Text = ds.Tables[0].Rows[0]["timed"].ToString();
venuelbl.Text = ds.Tables[0].Rows[0]["venue"].ToString();
string eventname = ds.Tables[0].Rows[0]["Passtype"].ToString().Trim().ToLower();
if (eventname == "VISITOR")
{
datelbl.Visible = false;
timelbl.Visible = false;
}
else if (eventname == "RESIDENT")
{
datelbl.Visible = false;
timelbl.Visible = false;
}
else if (eventname == "OTHERS")
{
datelbl.Visible = false;
timelbl.Visible = false;
}
}
else
{
ScriptManager.RegisterStartupScript(this, this.GetType(), "Message", "alert('Record not found');", true);
}
}
}
else
{
ScriptManager.RegisterStartupScript(this, this.GetType(), "Message", "alert('Timed Out');", true);
}
}
catch (SqlException ex)
{
string msg = "Error:";
msg += ex.Message;
throw new Exception(msg);
}
}
Below is the updated code using parameterized query and DataReader.
public void Details()
{
try
{
string connectionString = ConfigurationManager.ConnectionStrings["ConString"].ConnectionString;
using (SqlConnection con = new SqlConnection(connectionString))
{
using (SqlCommand cmd = new SqlCommand("SELECT * FROM DetailTable WHERE passname = @passname", con))
{
cmd.Parameters.AddWithValue("@passname", Session["index"]);
con.Open();
SqlDataReader dr = cmd.ExecuteReader();
if (dr.Read())
{
orgName.Text = dr["Name"].ToString();
title.Text = dr["passname"].ToString();
datelbl.Text = dr["Dated"] == DBNull.Value ? (string)null : Convert.ToDateTime(dr["Dated"]).ToLocalTime().ToString("MMM d, yyyy");
timelbl.Text = dr["timed"] == DBNull.Value ? (string)null : Convert.ToDateTime(dr["timed"]).ToLocalTime().ToString("hh:mm tt");
venuelbl.Text = dr["venue"].ToString();
string eventname = dr["Passtype"].ToString().Trim().ToLower();
if (eventname == "VISITOR")
{
datelbl.Visible = false;
timelbl.Visible = false;
}
else if (eventname == "RESIDENT")
{
datelbl.Visible = false;
timelbl.Visible = false;
}
else if (eventname == "OTHERS")
{
datelbl.Visible = false;
timelbl.Visible = false;
}
}
con.Close();
}
}
}
catch (SqlException ex)
{
string msg = "Error:";
msg += ex.Message;
throw new Exception(msg);
}
}
Now do I get to check this in the page load event, and not in the display code?
So that it will redirect to another page or show me message box?
protected void Page_Load(object sender, EventArgs e)
{
if (Session["index"] == null)
{
ScriptManager.RegisterStartupScript(this, this.GetType(), "Message", "alert('Timed Out');", true);
}
else
{
Details();
}
}
When you look at the initial code, I have this declaration that if "Session is null", it should do do something.
if (Session["index"] != null)
{
}
I did the same declaration in the updated code but, it did not work. So I had to put the declaration (Session["index"] != null){ } in the page load event. It worked though but I don't know if it is the correct thing to do.