Hello guys,
I am trying to set the query string parameters required like below;
[Authorize]
[HttpGet]
[ProducesResponseType(typeof(ReconciliationResponseDto),StatusCodes.Status200OK)]
[ProducesResponseType(StatusCodes.Status400BadRequest)]
[ProducesResponseType(StatusCodes.Status401Unauthorized)]
[ProducesResponseType(StatusCodes.Status500InternalServerError)]
[ProducesResponseType(StatusCodes.Status418ImATeapot)]
[Route("reconciliation")]
public async Task<IActionResult> GameReconciliation([FromQuery] ReconciliationDto reconciliationDto)
{
using var recon = await _services.GameReconciliation(reconciliationDto);
...
Here is the DTO;
public class ReconciliationDto
{
/// <summary>
/// Reconciliation Start Date.
/// </summary>
/// <example>2023-03-13</example>
[BindRequired]
//[Required(ErrorMessage = "Please add Reconciliation Start Date to the request.")]
[DataType(DataType.Date)]
public DateTime StartReconDateTime { get; set; }
/// <summary>
/// Reconciliation End Date.
/// </summary>
/// <example>2023-03-29</example>
[BindRequired]
//[Required(ErrorMessage = "Please add Reconciliation End Date to the request.")]
[DataType(DataType.Date)]
public DateTime EndReconDateTime { get; set; }
/// <summary>
/// Status of the purchased online game.
/// </summary>
/// <example>1</example>
[BindRequired]
//[Required(ErrorMessage = "Please enter: 1-Confirm, 2-Cancel")]
[Range(1, 2, ErrorMessage = "Please enter: 1-Confirm, 2-Cancel")]
public int Status { get; set; }
}
But I am getting 500 instead of 400 Bad request. How can I fix this so that it validates the query string parameters?
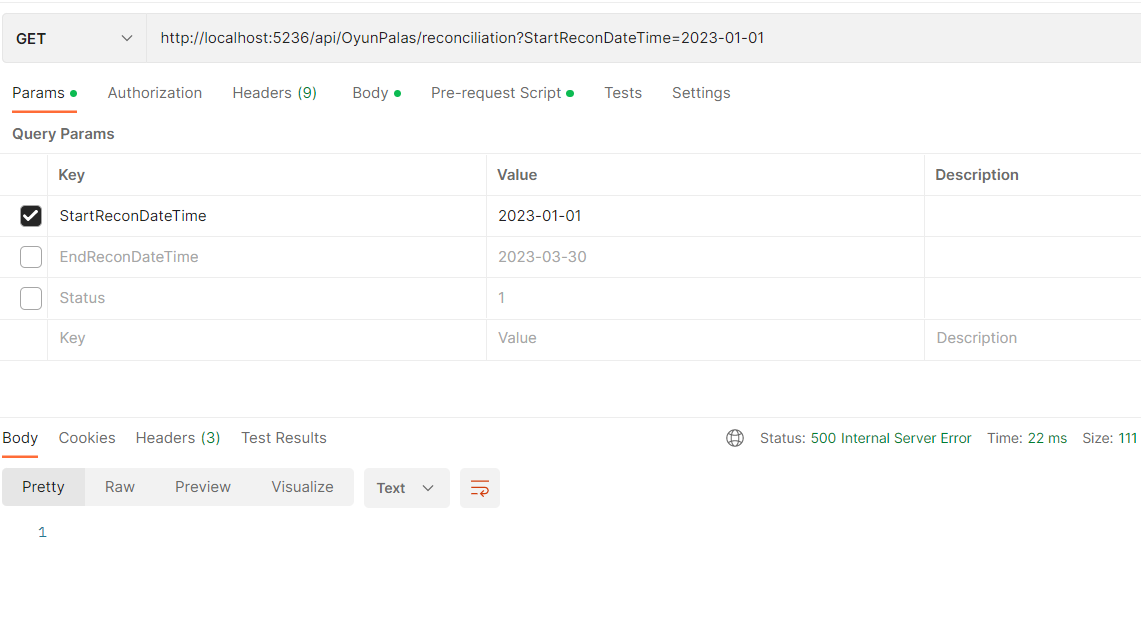
I think it is due to this;
builder.Services.Configure<ApiBehaviorOptions>(options
=> options.SuppressModelStateInvalidFilter = true);
When I remove this code above the response is like this;
{
"type": "https://tools.ietf.org/html/rfc7231#section-6.5.1",
"title": "One or more validation errors occurred.",
"status": 400,
"traceId": "00-59c4e66ac35ef0d009dc4760b72cc968-d8a263a291fa3401-00",
"errors": {
"Status": [
"A value for the 'Status' parameter or property was not provided."
],
"EndReconDateTime": [
"A value for the 'EndReconDateTime' parameter or property was not provided."
]
}
}
But I just want to display errors not the type, title traceId.