your code just wrote the file, then checked if it exists. why? is something else deleting the files? why write the file in the first place? why not stream to the excel reader to begin with? if you need to save the file, why open again? just open with read/write, and reset to position zero after copy
Blazor Server - How to get the latest uploaded file?
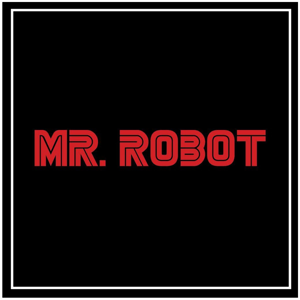
Cenk
956
Reputation points
Hi there,
In my Blazor Server application, I am uploading an Excel like this;
public async Task<int> UploadStocksFile(IFormFile file)
{
var untrustedFileName = file.FileName;
var totalRows = 0;
var duplicates = 0;
try
{
var path = Path.Combine(env.ContentRootPath,
env.EnvironmentName, "unsafe_uploads_stock",
untrustedFileName);
await using FileStream fs = new(path, FileMode.Create);
await file.CopyToAsync(fs);
logger.LogInformation("{untrustedFileName} saved at {Path}",
untrustedFileName, path);
var fi = new FileInfo(path);
// Check if the file exists
if (!fi.Exists)
throw new Exception("File " + path + " Does Not Exists");
//Check if file is an Excel File
if (untrustedFileName.Contains(".xls"))
{
using var ms = new MemoryStream();
await file.OpenReadStream().CopyToAsync(ms);
ExcelPackage.LicenseContext = LicenseContext.NonCommercial;
using var package = new ExcelPackage(ms);
var workSheet = package.Workbook.Worksheets["Stock"];
totalRows = workSheet.Dimension.Rows;
var gameList = new List<GameBank>();
for (var i = 2; i <= totalRows; i++)
{
gameList.Add(new GameBank
{
ProductDescription = workSheet.Cells[i, 1].Value.ToString(),
ProductCode = workSheet.Cells[i, 2].Value.ToString(),
UnitPrice = Convert.ToDouble(workSheet.Cells[i, 3].Value),
Quantity = Convert.ToInt16(workSheet.Cells[i, 4].Value),
Version = workSheet.Cells[i, 5].Value.ToString(),
Currency = workSheet.Cells[i, 6].Value.ToString(),
TotalPrice = Convert.ToDouble(workSheet.Cells[i, 7].Value),
Status = Convert.ToInt16(workSheet.Cells[i, 8].Value),
Used = Convert.ToInt16(workSheet.Cells[i, 9].Value),
RequestDateTime = DateTime.Now,
Signature = User.Identity.Name
});
gameList[i - 2].coupons = new GameBankPin
{
ExpiryDate = Convert.ToDateTime(workSheet.Cells[i, 10].Value),
Serial = workSheet.Cells[i, 11].Value.ToString(),
Pin = workSheet.Cells[i, 12].Value.ToString()
};
}
var existingRecords = await _stockUniqueSerialPinUseCase.ExecuteAsync(gameList);
if (existingRecords.Count > 0)
{
duplicates = existingRecords.Count;
}
}
}
catch (Exception ex)
{
logger.LogError("{untrustedFileName} error on upload (Err: 3): {Message}",
untrustedFileName, ex.Message);
}
return totalRows - 1 - duplicates;
}
I wonder if there is a way to get the latest uploaded Excel in another method?
Thank you.