Akash Madhu Thanks for posting your question in Microsoft Q&A. Based on the code snippet, I assume you are looking to create In-process Functions (.NET 6
) project with multiple triggers in the same project file.
I have modified the code snippet as below and added NuGet package Microsoft.Azure.WebJobs.Extensions.Storage
to the project and able to run in Visual Studio.
[FunctionName("SampleHttp")]
public static async Task<IActionResult> Run(
[HttpTrigger(AuthorizationLevel.Anonymous, "get", "post", Route = null)] HttpRequest req,
ILogger log)
{
log.LogInformation("C# HTTP trigger function processed a request.");
string name = req.Query["name"];
string requestBody = await new StreamReader(req.Body).ReadToEndAsync();
dynamic data = JsonConvert.DeserializeObject(requestBody);
name = name ?? data?.name;
string responseMessage = string.IsNullOrEmpty(name)
? "This HTTP triggered function executed successfully. Pass a name in the query string or in the request body for a personalized response."
: $"Hello, {name}. This HTTP triggered function executed successfully.";
return new OkObjectResult(responseMessage);
}
[FunctionName("SampleTimer")]
public static void SampleTimer(
[TimerTrigger("0 */5 * * * *")] TimerInfo myTimer,
ILogger log)
{
log.LogInformation($"Timer trigger function executed at: {DateTime.Now}");
}
[FunctionName("SampleQueue")]
public static void SampleQueue(
[QueueTrigger("myqueue-items", Connection = "AzureWebJobsStorage")] string myQueueItem,
ILogger log)
{
log.LogInformation($"Queue trigger function processed message: {myQueueItem}");
}
Output:
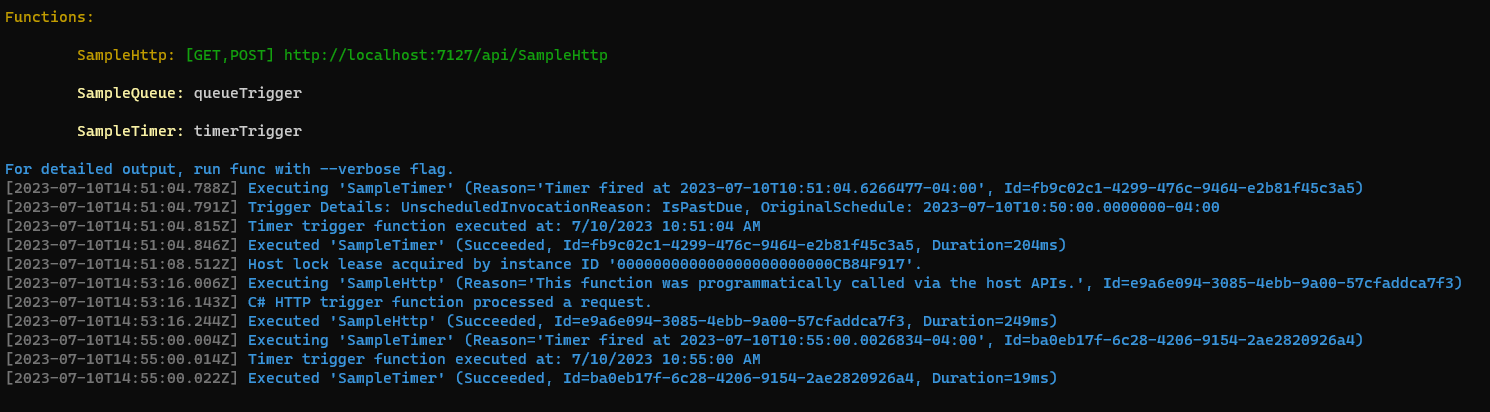
Can you follow the steps and the same code snippet and test it in your local development environment? If you face any issues in deploying or after the deployment to azure, provide the details such as full exception/error message including stack trace, deployment info (steps followed to deploy) etc. That would help in understanding the issue better.
Also, as suggested by Sander van de Velde, it is generally recommended to create multiple C# files in the same project and with this way, you can create a single Function app with multiple functions such as SampleHttp, SampleTimer, SampleQueue etc. in the resource group. Check out a sample reference and similar examples in the repo such as Azure serverless community library.
I hope this helps and let us know if you have any questions. Would be happy to answer any.