Hello there, I am Ravindra and I want to create or start meeting using graph API provided with the help of Python. Now, I have written code for it
import requests
import json
# Authentication details
client_id = "client_id"
client_secret = "client_secret"
tenant_id = "tenant_id"
resource = "https://graph.microsoft.com/"
# Get access token
auth_url = f"https://login.microsoftonline.com/{tenant_id}/oauth2/token"
auth_data = {
"grant_type": "client_credentials",
"client_id": client_id,
"client_secret": client_secret,
"resource": resource
}
auth_response = requests.post(auth_url, data=auth_data)
auth_response_data = auth_response.json()
access_token = auth_response_data["access_token"]
print(access_token)
# Set headers
headers = {
"Authorization": "Bearer " + access_token,
"Content-Type": "application/json"
}
# API endpoint to create a meeting
create_meeting_url = "https://graph.microsoft.com/v1.0/me/onlineMeetings"
# Set up the meeting payload
meeting_payload = {
"startDateTime": "2023-07-12T14:45:00",
"endDateTime": "2023-07-12T15:30:00",
"subject": "Testing sample meeting"
}
# Create the meeting
response = requests.post(create_meeting_url, headers=headers, data=json.dumps(meeting_payload))
if response.status_code == 201:
meeting_data = response.json()
meeting_id = meeting_data["id"]
print("Meeting created successfully. Meeting ID:", meeting_id)
else:
print("Failed to create meeting. Status code:", response.status_code)
It is giving me the following response
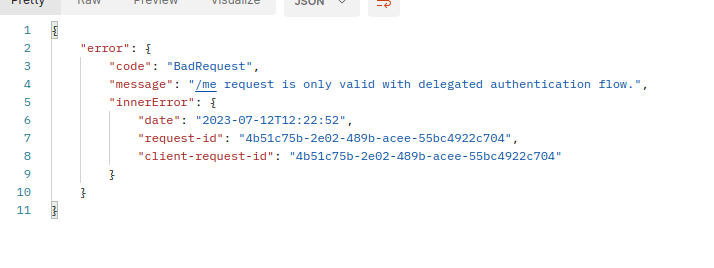
Please help me with this and give me the proper process of creating a call, recording it using Python, and getting the link to the recorded video after the call ends.
Thanks and Regards,
Ravindra Kanitkar