Hello,
I used an explicit index for editing a form. Why do we need an input hidden element when explicitly writing and pointing to a specific row for editing?
Thanks in advance
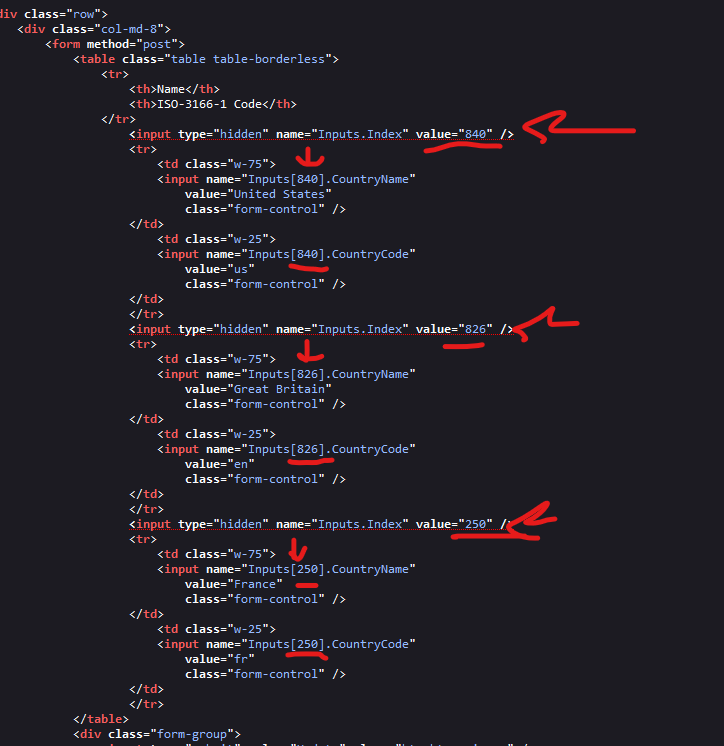
namespace CityBreaks.Models
{
public class Country
{
public int Id { get; set; }
public string CountryName { get; set; }
public string CountryCode { get; set; }
}
}
These two lines can point to a specific tag. So why do we need a hidden element?
<input name="Inputs[@country.Id].CountryCode"
<input name="Inputs[@country.Id].CountryName"
@page
@model CityBreaks.Pages.CountryManager.EditModel
@{
}
<h4>Edit Countries</h4>
<div class="row">
<div class="col-md-8">
<form method="post">
<table class="table table-borderless">
<tr>
<th>Name</th>
<th>ISO-3166-1 Code</th>
</tr>
@foreach (var country in Model.Inputs)
{
<input type="hidden" name="Inputs.Index" value="@country.Id" /> //Why do we need this tag when we explicitly write and point to specific row in our list ?
<tr>
<td class="w-75">
<input name="Inputs[@country.Id].CountryName"
value="@country.CountryName"
class="form-control" />
</td>
<td class="w-25">
<input name="Inputs[@country.Id].CountryCode"
value="@country.CountryCode"
class="form-control" />
</td>
</tr>
}
</table>
<div class="form-group">
<input type="submit" value="Update" class="btn btn-primary" />
</div>
</form>
</div>
</div>
@if (Model.Countries.Any())
{
<p>You submitted the following</p>
<ul>
@foreach (var country in Model.Countries)
{
<li>
<img src="/images/flags/@(country.CountryCode).png" />
@country.CountryName
</li>
}
</ul>
}
using CityBreaks.Models;
using Microsoft.AspNetCore.Mvc;
using Microsoft.AspNetCore.Mvc.RazorPages;
namespace CityBreaks.Pages.CountryManager
{
public class EditModel : PageModel
{
[BindProperty]
public List<InputModel> Inputs { get; set; }
public List<Country> Countries { get; set; } = new List<Country>();
public void OnGet()
{
Inputs = new List<InputModel> {
new InputModel{ Id = 840, CountryCode = "us", CountryName ="United States" },
new InputModel{ Id = 826, CountryCode = "en", CountryName = "Great Britain" },
new InputModel{ Id = 250, CountryCode = "fr", CountryName = "France" }
};
}
public void OnPost()
{
Countries = Inputs
.Where(x => !string.IsNullOrWhiteSpace(x.CountryCode))
.Select(x => new Country
{
Id = x.Id,
CountryCode = x.CountryCode,
CountryName = x.CountryName
}).ToList();
}
public class InputModel
{
public int Id { get; set; }
public string CountryName { get; set; }
public string CountryCode { get; set; }
}
}
}