Hi @Nandini S
how to delete employees associated with department , means I want to add one dropdown to selct deprtment name and i need to display employee list for which deprtment is selected and i need to delete that employees
You can use a partial view to display the employee list, after the drondownlist selected value changes, you can use JQuery ajax to call the handler method to filter the employee based on the selected department id property, then return a partial view.
In the partial view, you can add a column with a hyper link (delete button), and add the employee id as the route parameter, then after clicking the Delete button, it will go the delete handler the remove the specified employee from the database.
Code as below:
Create a _employeePV.cshtml partial view in the Pages/Shared folder:
@model List<RazorWebApp.Models.Employee>
<table class="table">
<thead>
<tr>
<th>
@Html.DisplayNameFor(model => model[0].EmployeeCode)
</th>
<th>
@Html.DisplayNameFor(model => model[0].Name)
</th>
<th>
@Html.DisplayNameFor(model => model[0].Gender)
</th>
<th>
@Html.DisplayNameFor(model => model[0].Email)
</th>
<th>
@Html.DisplayNameFor(model => model[0].Country)
</th>
<th>
@Html.DisplayNameFor(model => model[0].Department.DepartmentName)
</th>
<th></th>
</tr>
</thead>
<tbody>
@foreach (var item in Model)
{
<tr>
<td>
@Html.DisplayFor(modelItem => item.EmployeeCode)
</td>
<td>
@Html.DisplayFor(modelItem => item.Name)
</td>
<td>
@Html.DisplayFor(modelItem => item.Gender)
</td>
<td>
@Html.DisplayFor(modelItem => item.Email)
</td>
<td>
@Html.DisplayFor(modelItem => item.Country)
</td>
<td>
@Html.DisplayFor(modelItem => item.Department.DepartmentName)
</td>
<td>
<a asp-page="/Employees/Index" asp-page-handler="delete" asp-route-id="@item.EmployeeCode">Delete</a>
</td>
</tr>
}
</tbody>
</table>
Then in the Index.cshtml page: use partial tag to display the partial view, then in the dropdownlist change event, use jquery ajax to call the handler method to find employees by the department id.
@page
@model RazorWebApp.Pages.Employees.IndexModel
@addTagHelper *, Microsoft.AspNetCore.Mvc.TagHelpers
<select id="ddlDepartment" asp-items="Model.DepartmentOptions">
<option value="-1">Select Department</option>
</select>
<div id="divcontainer">
<partial name="../Pages/Shared/_employeePV" model="Model.Employees" />
</div>
@section Scripts{
<script>
$(function(){
$("#ddlDepartment").change(function(){
var selectedDepID = $(this).val();
$.ajax({
type: "Get",
url: "/Employees/Index?handler=FindEmployeeByDep",
beforeSend: function (xhr) {
xhr.setRequestHeader("RequestVerificationToken",
$('input:hidden[name="__RequestVerificationToken"]').val());
},
data: { "id": selectedDepID },
success: function (response) {
//clear the container and then append the partial page in the container.
$("#divcontainer").html("");
$("#divcontainer").html(response);
},
error: function (response) {
console.log(response.responseText);
}
});
});
});
</script>
}
Index.cshtml.cs page:
public class IndexModel : PageModel
{
private readonly ApplicationDbContext _context;
public IndexModel(ApplicationDbContext applicationDbContext)
{
_context=applicationDbContext;
}
public List<Employee> Employees { get; set; }
public List<SelectListItem> DepartmentOptions { get; set; }
public void OnGet()
{
DepartmentOptions = _context.Departments.Select(a =>
new SelectListItem
{
Value = a.Id.ToString(),
Text = a.DepartmentName
}).ToList();
Employees = _context.Employees.Include(c => c.Department).ToList();
}
public IActionResult OnGetFindEmployeeByDep(int id)
{
var emplist = new List<Employee>();
if(id == -1)
{ //return all employee
emplist = _context.Employees.Include(c => c.Department).ToList();
}
else
{
emplist = _context.Employees.Include(c => c.Department).Where(c => c.DepartmentId == id).ToList();
}
return Partial("../Pages/Shared/_employeePV", emplist);
}
public IActionResult OnGetDelete(string id)
{
var item = _context.Employees.Where(c=>c.EmployeeCode == id).FirstOrDefault();
_context.Employees.Remove(item);
_context.SaveChanges();
//query the latest data
Employees = _context.Employees.Include(c => c.Department).ToList();
DepartmentOptions = _context.Departments.Select(a =>
new SelectListItem
{
Value = a.Id.ToString(),
Text = a.DepartmentName
}).ToList();
return Page();
}
}
The result as below:
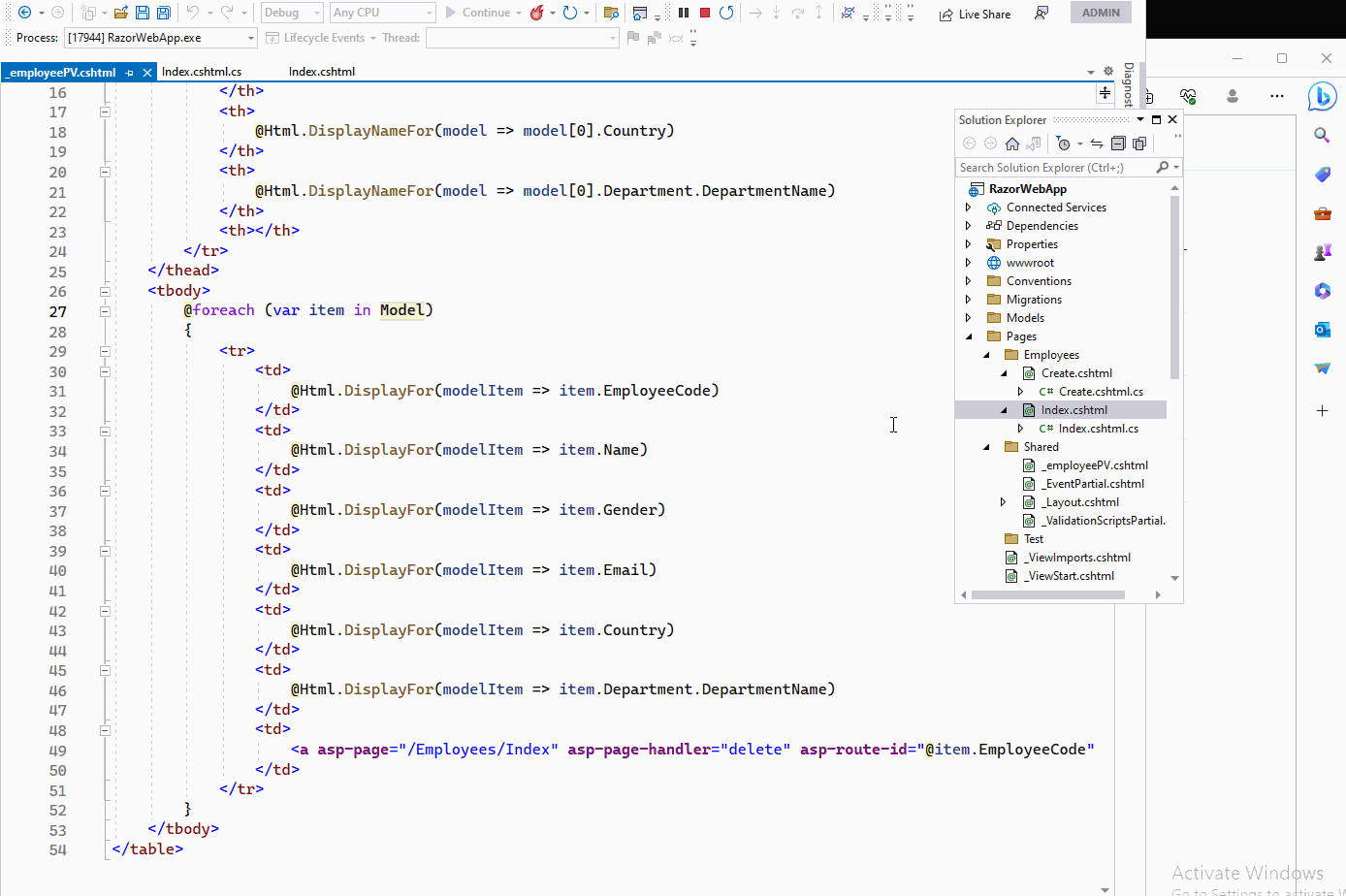
If you want to delete multiple employees at one time, in the partial view, you can add a column with checkbox, and modify the code as below:
_employeePV.cshtml:
<td>
<input class="chk_isDelete" type="checkbox" data-employeecode="@item.EmployeeCode" />
@*<a asp-page="/Employees/Index" asp-page-handler="delete" asp-route-id="@item.EmployeeCode">Delete</a>*@
</td>
Index.cshtml:
@page
@model RazorWebApp.Pages.Employees.IndexModel
@addTagHelper *, Microsoft.AspNetCore.Mvc.TagHelpers
<select id="ddlDepartment" asp-items="Model.DepartmentOptions">
<option value="-1">Select Department</option>
</select>
<input type="button" class="btn btn-primary" id="btndelete" value="Delete" />
<div id="divcontainer">
<partial name="../Pages/Shared/_employeePV" model="Model.Employees" />
</div>
@section Scripts{
<script>
$(function(){
$("#ddlDepartment").change(function(){
var selectedDepID = $(this).val();
$.ajax({
type: "Get",
url: "/Employees/Index?handler=FindEmployeeByDep",
beforeSend: function (xhr) {
xhr.setRequestHeader("RequestVerificationToken",
$('input:hidden[name="__RequestVerificationToken"]').val());
},
data: { "id": selectedDepID },
success: function (response) {
//clear the container and then append the partial page in the container.
$("#divcontainer").html("");
$("#divcontainer").html(response);
},
error: function (response) {
console.log(response.responseText);
}
});
});
$("#btndelete").click(function(){
var id = [];
$("#divcontainer input[type='checkbox']:checked").each(function (index, item) {
id.push($(item).attr("data-employeecode"));
});
$.ajax({
type: "Get",
url: "/Employees/Index?handler=delete",
beforeSend: function (xhr) {
xhr.setRequestHeader("RequestVerificationToken",
$('input:hidden[name="__RequestVerificationToken"]').val());
},
data: { "id": JSON.stringify(id) },
success: function (response) {
//clear the container and then append the partial page in the container.
//$("#divcontainer").html("");
//$("#divcontainer").html(response);
window.location.href="/Employees/Index"; //refresh the page
},
error: function (response) {
console.log(response.responseText);
}
});
});
});
</script>
}
Index.cshtml.cs
public IActionResult OnGetDelete(string id)
{
var ids = System.Text.Json.JsonSerializer.Deserialize<List<string>>(id);
var item = _context.Employees.Where(c=> ids.Contains(c.EmployeeCode)).ToList();
_context.Employees.RemoveRange(item);
_context.SaveChanges();
//query the latest data
Employees = _context.Employees.Include(c => c.Department).ToList();
DepartmentOptions = _context.Departments.Select(a =>
new SelectListItem
{
Value = a.Id.ToString(),
Text = a.DepartmentName
}).ToList();
return Page();
}
}
The result as below:
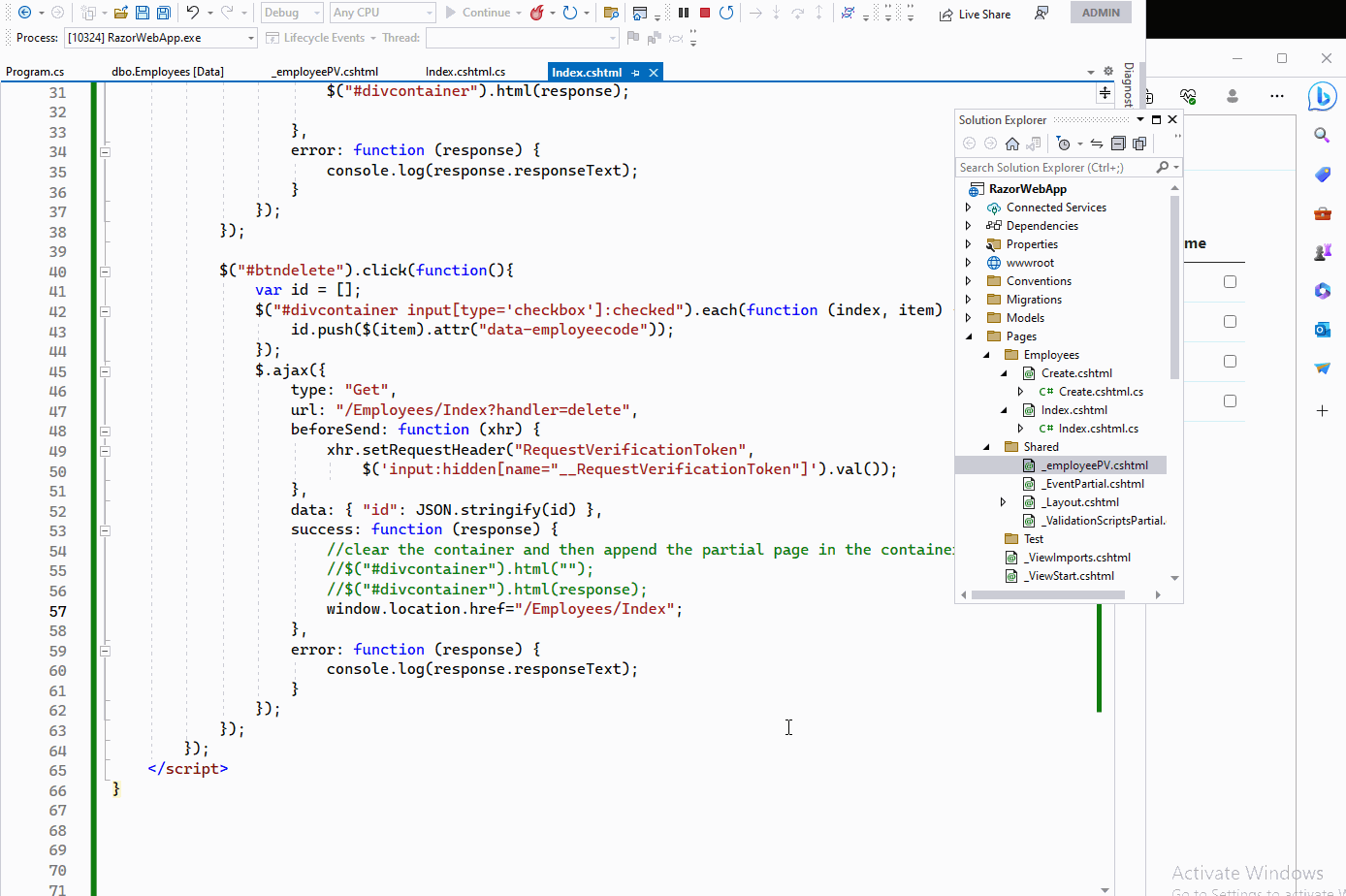
If the answer is the right solution, please click "Accept Answer" and kindly upvote it. If you have extra questions about this answer, please click "Comment".
Note: Please follow the steps in our documentation to enable e-mail notifications if you want to receive the related email notification for this thread.
Best regards,
Dillion