I am trying to implement push notification on my MAUI
application using FCM
. I follow this blog and I did everything as per the blog.
They suggest to install only 2 NuGet packages, Plugin.Firebase
and Plugin.Firebase.Crashlytics
. (I installed it by adding it on the .csproj like below)
<ItemGroup Condition="'$(TargetFramework)' == 'net7.0-ios' OR '$(TargetFramework)' == 'net7.0-android'">
<PackageReference Include="Plugin.Firebase" Version="2.0.3" />
<PackageReference Include="Plugin.Firebase.Crashlytics" Version="2.0.1" />
</ItemGroup>
After adding the packages I have removed Unsupported TargetFrameworks
by removing the MAC and windows framework code from .csproj file.
Then added the google-services.json
file to the root folder of the project. But GoogleServicesJson
Build Action is not showing in the list (This is the very first problem I am facing). So I added it on the .csproj
manually.
<ItemGroup Condition="'$(TargetFramework)' == 'net7.0-android'">
<GoogleServicesJson Include="google-services.json" />
</ItemGroup>
Then updated MauiProgram.cs
like below:
using Microsoft.Maui.LifecycleEvents;
using Plugin.Firebase.Auth;
using Plugin.Firebase.Bundled.Shared;
#if IOS
using Plugin.Firebase.Bundled.Platforms.iOS;
#else
using Plugin.Firebase.Bundled.Platforms.Android;
#endif
namespace MauiFirebaseDemo;
public static class MauiProgram
{
public static MauiApp CreateMauiApp()
{
var builder = MauiApp.CreateBuilder();
builder
.UseMauiApp<App>()
.RegisterFirebaseServices()
.ConfigureFonts(fonts =>
{
fonts.AddFont("OpenSans-Regular.ttf", "OpenSansRegular");
fonts.AddFont("OpenSans-Semibold.ttf", "OpenSansSemibold");
});
return builder.Build();
}
private static MauiAppBuilder RegisterFirebaseServices(this MauiAppBuilder builder)
{
builder.ConfigureLifecycleEvents(events => {
#if IOS
events.AddiOS(iOS => iOS.FinishedLaunching((app, launchOptions) => {
CrossFirebase.Initialize(CreateCrossFirebaseSettings());
return false;
}));
#else
events.AddAndroid(android => android.OnCreate((activity, _) =>
CrossFirebase.Initialize(activity, CreateCrossFirebaseSettings())));
#endif
});
builder.Services.AddSingleton(_ => CrossFirebaseAuth.Current);
return builder;
}
private static CrossFirebaseSettings CreateCrossFirebaseSettings()
{
return new CrossFirebaseSettings(isAuthEnabled: true,);
}
}
But a lot of properties in the new code are not exist. Check the below screenshots:
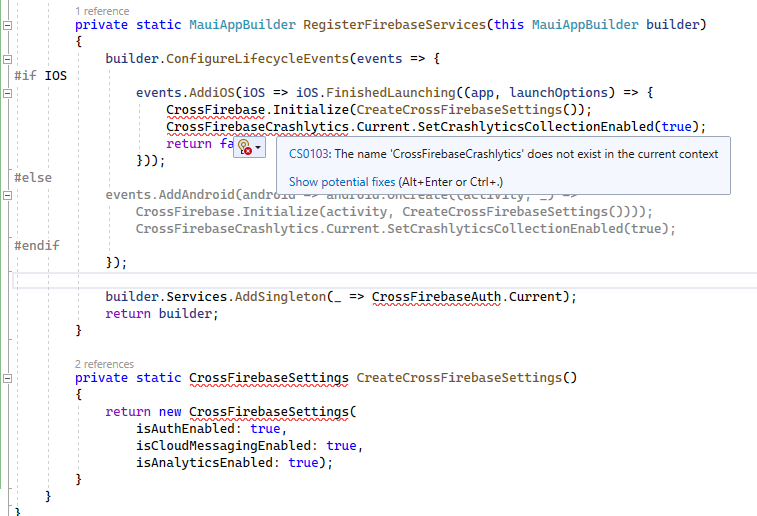
Using section also has the same kind issues: But the packages mention under using section are not installed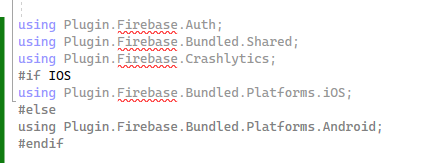
On android platform I have done the below set up.
At Platforms/Android/Resources/values
added the following line to strings.xml
:
<?xml version="1.0" encoding="utf-8" ?>
<resources>
<string name="com.google.firebase.crashlytics.mapping_file_id">none</string>
</resources>
Added the following ItemGroup to the.csproj file to prevent build errors:
<ItemGroup Condition="'$(TargetFramework)' == 'net7.0-android'">
<PackageReference Include="Xamarin.Kotlin.StdLib.Jdk7" Version="1.7.10" ExcludeAssets="build;buildTransitive" />
<PackageReference Include="Xamarin.Kotlin.StdLib.Jdk8" Version="1.7.10" ExcludeAssets="build;buildTransitive" />
</ItemGroup>
In the AndroidManifest.xml
. Added the following code snippet to the tag :
<receiver
android:name="com.google.firebase.iid.FirebaseInstanceIdInternalReceiver"
android:exported="false" />
<receiver
android:name="com.google.firebase.iid.FirebaseInstanceIdReceiver"
android:exported="true"
android:permission="com.google.android.c2dm.permission.SEND">
<intent-filter>
<action android:name="com.google.android.c2dm.intent.RECEIVE" />
<action android:name="com.google.android.c2dm.intent.REGISTRATION" />
<category android:name="${applicationId}" />
</intent-filter>
</receiver>
Then updated MainActivity.cs
like below:
using Android.App;
using Android.Content;
using Android.Content.PM;
using Android.OS;
using Plugin.Firebase.CloudMessaging;
namespace MauiFirebaseDemo;
[Activity(Theme = "@style/Maui.SplashTheme", MainLauncher = true, ConfigurationChanges = ConfigChanges.ScreenSize | ConfigChanges.Orientation | ConfigChanges.UiMode | ConfigChanges.ScreenLayout | ConfigChanges.SmallestScreenSize | ConfigChanges.Density)]
public class MainActivity : MauiAppCompatActivity
{
protected override void OnCreate(Bundle savedInstanceState)
{
base.OnCreate(savedInstanceState);
HandleIntent(Intent);
CreateNotificationChannelIfNeeded();
}
protected override void OnNewIntent(Intent intent)
{
base.OnNewIntent(intent);
HandleIntent(intent);
}
private static void HandleIntent(Intent intent)
{
FirebaseCloudMessagingImplementation.OnNewIntent(intent);
}
private void CreateNotificationChannelIfNeeded()
{
if (Build.VERSION.SdkInt >= BuildVersionCodes.O)
{
CreateNotificationChannel();
}
}
private void CreateNotificationChannel()
{
var channelId = $"{PackageName}.general";
var notificationManager = (NotificationManager)GetSystemService(NotificationService);
var channel = new NotificationChannel(channelId, "General", NotificationImportance.Default);
notificationManager.CreateNotificationChannel(channel);
FirebaseCloudMessagingImplementation.ChannelId = channelId;
//FirebaseCloudMessagingImplementation.SmallIconRef = Resource.Drawable.ic_push_small;
}
}
Here also I am getting the same kind of error on code and using section:
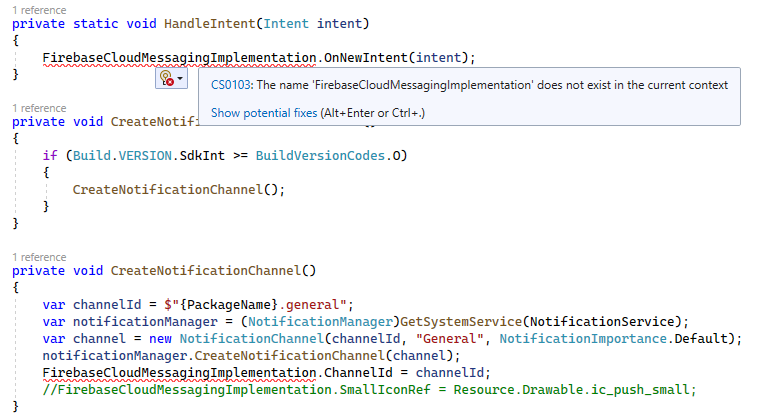
Under using section:

Is this the right approach to implement push notification on MAUI
? I saw a similar kind of implementation here too. How can I fix the error on MauiProgram.cs
and MainActivity.cs
? Should I install any other NuGet packages? How can I set GoogleServicesJson Build Action for google-services.json?
I have tried installing the Plugin.Firebase.CloudMessaging
and Plugin.Firebase.Auth
for resolving the errors on MauiProgram.cs
and MainActivity.cs
. And tried installing the Xamarin.GooglePlayServices.Basement
for resolving the GoogleServicesJson Build Action, but no lucks.
All the errors are does not exist in the current context
, that's why added screenshot in the question. Sample error details are added in text format below:
The name 'CrossFirebase' does not exist in the current context
The type or namespace name 'Firebase' does not exist in the namespace 'Plugin' (are you missing an assembly reference?)