I recommend using a strong typed data source, usually the average developer will use a DataTable. Let's look at using a DataTable in tangent with a BindingSource.
There are several language extensions in this case which may seem overboard but when placed into a separate class project allow them to be used in more than one project.
In this code sample, the DataGridView DataSource points to a BindingSource which has been loaded with a DataTable.
Then in TextChanged event we use a language extension to find text in the first column of the DataGridView. By default the filter is case insensitive.
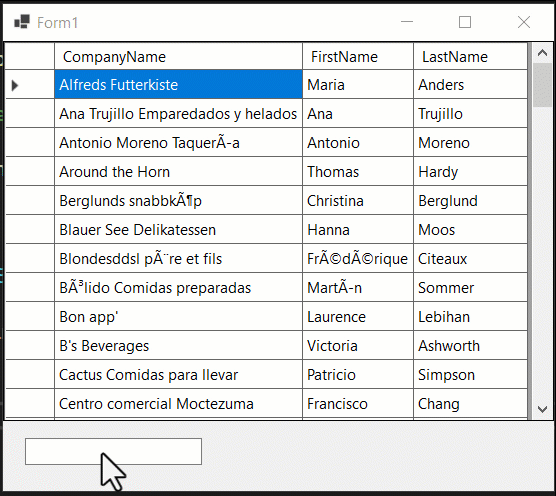
Full source is mixed in with another code sample here.
public partial class Form1 : Form
{
BindingSource _bindingSource = new();
public Form1()
{
InitializeComponent();
// GetCustomers returns a DataTable
_bindingSource.DataSource = DataOperations.GetCustomers();
dataGridView1.DataSource = _bindingSource;
// resize columns to fit column width from database
dataGridView1.ExpandColumns();
FilterTextBox.TextChanged += FilterTextBox_TextChanged;
}
private void FilterTextBox_TextChanged(object sender, EventArgs e)
{
_bindingSource.RowFilterStartsWith("CompanyName", FilterTextBox.Text);
}
}
public static class StringExtensions
{
public static string EscapeApostrophe(this string pSender)
=> pSender.Replace("'", "''");
}
public static class BindingSourceExtensions
{
public static DataTable DataTable(this BindingSource sender)
=> (DataTable)sender.DataSource;
public static DataView DataView(this BindingSource sender)
=> ((DataTable)sender.DataSource).DefaultView;
public static void RowFilterStartsWith(this BindingSource sender, string field, string value, bool caseSensitive = false)
{
sender.DataTable().CaseSensitive = caseSensitive;
sender.DataView().RowFilter = $"{field} LIKE '{value.EscapeApostrophe()}%'";
}
public static void RowFilterContains(this BindingSource sender, string field, string value, bool caseSensitive = false)
{
sender.DataTable().CaseSensitive = caseSensitive;
sender.DataView().RowFilter = $"{field} LIKE '%{value.EscapeApostrophe()}%'";
}
public static void RowFilterEndsWith(this BindingSource sender, string field, string value, bool caseSensitive = false)
{
sender.DataTable().CaseSensitive = caseSensitive;
sender.DataView().RowFilter = $"{field} LIKE '%{value.EscapeApostrophe()}'";
}
}