Hi @Donald Symmons,
I guess it is possible, my idea is that you can upload to database and then get the images from the database and then delete the unwanted ones. This method should be more convenient when you want to upload multiple images.
<asp:FileUpload ID="FileUpload1" runat="server" />
<asp:Button ID="btnUpload" runat="server" Text="Upload" OnClick="Upload" />
<br />
<asp:Label ID="lblMessage" runat="server" Text="" Font-Names="Arial"></asp:Label>
<hr />
<asp:GridView ID="GridView1" runat="server" AutoGenerateColumns="false" DataKeyNames="Id" OnRowDeleting="GridView1_RowDeleting">
<Columns>
<asp:TemplateField HeaderText="Image">
<ItemTemplate>
<asp:Image ID="Image1" runat="server" ImageUrl='<%# ToBase64(Eval("Data")) %>' />
</ItemTemplate>
</asp:TemplateField>
<asp:CommandField ShowDeleteButton="True" ButtonType="Button" />
</Columns>
</asp:GridView>
protected void Page_Load(object sender, EventArgs e)
{
if (!this.IsPostBack)
{
BindGridView();
}
}
protected string ToBase64(object data)
{
byte[] bytes = (byte[])data;
string base64String = Convert.ToBase64String(bytes, 0, bytes.Length);
return "data:image/png;base64," + base64String;
}
protected void Upload(object sender, EventArgs e)
{
string filePath = string.Empty;
string contenttype = string.Empty;
string filename = string.Empty;
byte[] bytes = null;
if (this.FileUpload1.HasFile)
{
filePath = FileUpload1.PostedFile.FileName;
filename = Path.GetFileName(filePath);
string ext = Path.GetExtension(filename).ToLower();
contenttype = string.Empty;
switch (ext)
{
case ".jpg":
contenttype = "image/jpg";
break;
case ".png":
contenttype = "image/png";
break;
case ".gif":
contenttype = "image/gif";
break;
}
using (Stream fs = FileUpload1.PostedFile.InputStream)
{
using (BinaryReader br = new BinaryReader(fs))
{
bytes = br.ReadBytes((Int32)fs.Length);
}
}
}
else
{
filename = "Default.png";
contenttype = "image/png";
System.Drawing.Image defaultImage = System.Drawing.Image.FromFile(Server.MapPath("~/Images/Default.png"));
using (MemoryStream ms = new MemoryStream())
{
defaultImage.Save(ms, System.Drawing.Imaging.ImageFormat.Jpeg);
bytes = ms.ToArray();
}
}
string query = "INSERT INTO tblFiles(Name, ContentType, Data) VALUES (@Name, @ContentType, @Data)";
string constr = ConfigurationManager.ConnectionStrings["ConString"].ConnectionString;
using (SqlConnection con = new SqlConnection(constr))
{
using (SqlCommand cmd = new SqlCommand(query))
{
cmd.Parameters.Add("@Name", SqlDbType.VarChar).Value = filename;
cmd.Parameters.Add("@ContentType", SqlDbType.VarChar).Value = contenttype;
cmd.Parameters.Add("@Data", SqlDbType.Binary).Value = bytes;
cmd.CommandType = CommandType.Text;
cmd.Connection = con;
con.Open();
cmd.ExecuteNonQuery();
con.Close();
}
}
lblMessage.ForeColor = Color.Green;
lblMessage.Text = "File Uploaded Successfully";
Response.Redirect(Request.Url.AbsoluteUri);
}
protected void GridView1_RowDeleting(object sender, GridViewDeleteEventArgs e)
{
DataTable dt = (DataTable)ViewState["Data"];
int index = Convert.ToInt32(e.RowIndex);
// Delete from ViewState.
dt.Rows[index].Delete();
ViewState["Data"] = dt;
if (GridView1.DataKeys[e.RowIndex].Value != DBNull.Value)
{
int id = Convert.ToInt32(GridView1.DataKeys[index].Value);
// Delete from Database.
string constr = ConfigurationManager.ConnectionStrings["ConString"].ConnectionString;
using (SqlConnection con = new SqlConnection(constr))
{
string query = "DELETE FROM tblFiles WHERE Id = @Id";
using (SqlCommand cmd = new SqlCommand(query))
{
cmd.Connection = con;
cmd.Parameters.AddWithValue("@Id", id);
con.Open();
cmd.ExecuteNonQuery();
con.Close();
}
}
}
BindGridView();
}
private void BindGridView()
{
string constr = ConfigurationManager.ConnectionStrings["ConString"].ConnectionString;
using (SqlConnection con = new SqlConnection(constr))
{
string query = "SELECT Id, Name, Data FROM tblFiles";
using (SqlCommand cmd = new SqlCommand(query))
{
cmd.Connection = con;
SqlDataAdapter sda = new SqlDataAdapter(cmd);
DataTable dt = new DataTable();
sda.Fill(dt);
ViewState["Data"] = dt;
GridView1.DataSource = dt;
GridView1.DataBind();
}
}
}
CREATE TABLE [dbo].[tblFiles] (
[Id] INT IDENTITY (1, 1) NOT NULL,
[Name] VARCHAR (50) NULL,
[ContentType] VARCHAR (100) NULL,
[Data] VARBINARY (MAX) NULL
);
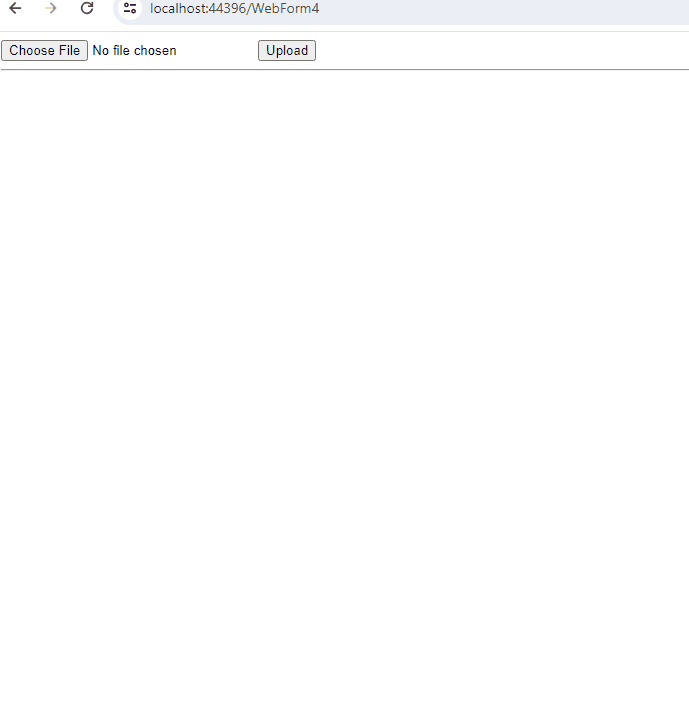
Best regards,
Lan Huang
If the answer is the right solution, please click "Accept Answer" and kindly upvote it. If you have extra questions about this answer, please click "Comment".
Note: Please follow the steps in our documentation to enable e-mail notifications if you want to receive the related email notification for this thread.