I am building a durable function using visual studio 2022 + .net 8.0 isolated. Currently the durable function contain a simple function to get the list info of 2 SharePoint lists, here what i have built so far:-
ActivityFunction.cs:-
using Microsoft.Azure.WebJobs;
using PnP.Core.Model.SharePoint;
using PnP.Core.Services;
using Microsoft.Extensions.Logging;
using Microsoft.Azure.WebJobs.Extensions.DurableTask;
namespace SPDashBoard
{
public class ActivityFunctions
{
private readonly IPnPContextFactory pnpContextFactory;
public ActivityFunctions(IPnPContextFactory pnpContextFactory)
{
this.pnpContextFactory = pnpContextFactory;
}
[FunctionName(nameof(GetListInfo))]
public async Task<IListDetails> GetListInfo([ActivityTrigger] string input,ILogger log)
{
log.LogWarning("About to call GetListInfo activity!");
using (var objPnPContext = await pnpContextFactory.CreateAsync("Default"))
{
IList lstTarget = await objPnPContext.Web.Lists.GetByTitleAsync(input, l => l.Title,
l => l.TemplateType, log => log.ItemCount);
await Task.Delay(1000);
return new IListDetails()
{
Title = lstTarget.Title,
TemplateType = lstTarget.TemplateType.ToString(),
ItemCount = lstTarget.ItemCount
};
}
}
}
}
OrchestrationFunctions.cs:-
using Microsoft.Azure.WebJobs;
using Microsoft.Azure.WebJobs.Extensions.DurableTask;
using Microsoft.Extensions.Logging;
using System;
using System.Collections.Generic;
using System.Threading.Tasks;
namespace SPDashBoard
{
public class OrchestratorFunctions
{
[FunctionName("GetListsDetailsChaining")]
public async Task<List<IListDetails>> GetListsDetailsChaining(
[Microsoft.Azure.WebJobs.Extensions.DurableTask.OrchestrationTrigger] IDurableOrchestrationContext context,ILogger log)
{
log = context.CreateReplaySafeLogger(log);
string[] listArr = new string[] { "WorkOrders", "Technicians" };
List<IListDetails> listDetails = new List<IListDetails>();
foreach (var strList in listArr)
{
IListDetails lstDetails = await context.CallActivityAsync<IListDetails>(nameof(ActivityFunctions.GetListInfo), strList);
listDetails.Add(lstDetails);
}
return listDetails;
}
}
}
Functions.cs
using Microsoft.Azure.WebJobs;
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using Microsoft.Azure.WebJobs.Extensions;
using Microsoft.Azure.Functions.Worker;
using Microsoft.Azure.WebJobs.Extensions.DurableTask;
using Microsoft.Extensions.Logging;
namespace SPDashBoard
{
public static class Functions
{
[FunctionName("GetListsDetailsChaining_SchduleStart")]
public static async Task<string> GetListsDetailsChaining_SchduleStart( [TimerTrigger("0 */5 * * * *")] TimerInfo myTimer, //[TimerTrigger("0 0 0 * * *")] TimerInfo myTimer,
[Microsoft.Azure.WebJobs.Extensions.DurableTask.DurableClient] IDurableOrchestrationClient starter,ILogger log)
{
string instanceId = string.Empty;
log.LogWarning("Starting the http starter Function = GetListDetails");
instanceId = await starter.StartNewAsync(nameof(OrchestratorFunctions.GetListsDetailsChaining), null);
DurableOrchestrationStatus durableOrchestrationStatus = await starter.GetStatusAsync(instanceId);
while (durableOrchestrationStatus.RuntimeStatus != OrchestrationRuntimeStatus.Completed)
{
await Task.Delay(200);
durableOrchestrationStatus = await starter.GetStatusAsync(instanceId);
}
return "done";
}
}
}
Startup.cs:-
using Microsoft.Azure.Functions.Extensions.DependencyInjection;
using Microsoft.Extensions.Configuration;
using Microsoft.Extensions.DependencyInjection;
using PnP.Core.Auth;
using System.Security.Cryptography.X509Certificates;
[assembly: FunctionsStartup(typeof(SPDashBoard.Startup))]
namespace SPDashBoard
{
class Startup : FunctionsStartup
{
public override void Configure(IFunctionsHostBuilder builder)
{
var config = builder.GetContext().Configuration;
var azureFunctionSettings = new AzureFunctionSettings();
config.Bind(azureFunctionSettings);
builder.Services.AddPnPCore(options =>
{
options.DisableTelemetry = true;
var authProvider = new X509CertificateAuthenticationProvider(azureFunctionSettings.ClientId,
azureFunctionSettings.TenantId,
StoreName.My,
StoreLocation.CurrentUser,
azureFunctionSettings.CertificateThumbprint);
options.DefaultAuthenticationProvider = authProvider;
options.Sites.Add("Default", new PnP.Core.Services.Builder.Configuration.PnPCoreSiteOptions
{
SiteUrl = azureFunctionSettings.SiteUrl,
AuthenticationProvider = authProvider
});
});
}
}
}
Program.cs
using Microsoft.Azure.Functions.Worker;
using Microsoft.Extensions.DependencyInjection;
using Microsoft.Extensions.Hosting;
var host = new HostBuilder()
.ConfigureFunctionsWebApplication()
.ConfigureServices(services =>
{
services.AddApplicationInsightsTelemetryWorkerService();
services.ConfigureFunctionsApplicationInsights();
})
.Build();
host.Run();
but when i run the visual studio project i got this message that "no job function found", as follow:-
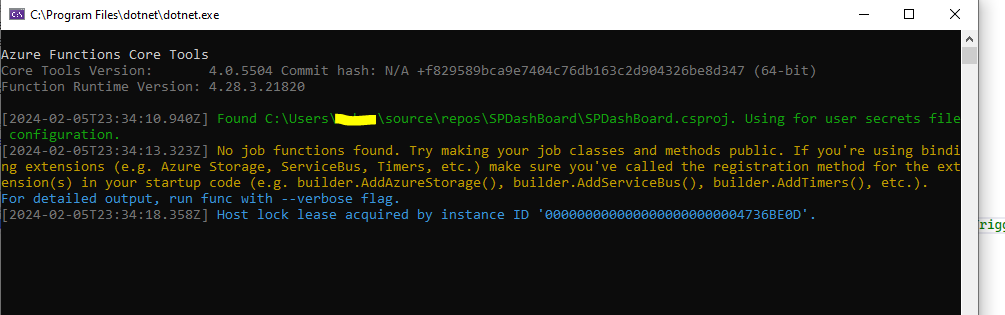
any advice? why my project did not understand the function i built to query the SharePoint lists?