Hi @Anthony Poudel
I am beginner in Asp dot net core and I want to use make login functionality in my project but don't have an idea on how to do it, so if you can help me it would be great.
Asp.net core is an API and Razor Class Library. we can use the Scaffold Identity to generate pages related to user management features, then use the provided methods to manages users, passwords, profile data, roles, claims, tokens, email confirmation, and more.
In this scenario, you have to use the identity related table such as the AspNetUsers table, if you want to add custom data to the Identity table, you can refer to this tutorial: Add, download, and delete custom user data to Identity in an ASP.NET Core project.
How can I use different table in asp dot net identity rather that identityUser table, what configuration need to be done in the dbconxtext, controller and program.cs file?
If you don't want to use Asp.Net Core Identity, and want to create the User table and the related pages (for example the register and login page) by yourself, it is similar to use EF core in asp.net core, you have to do the following:
1.Install the EF core Nuget packages (using the sql server database):
- Microsoft.AspNetCore.Diagnostics.EntityFrameworkCore
- Microsoft.EntityFrameworkCore.SqlServer
- Microsoft.EntityFrameworkCore.Design
2.Create the Uset Entity
public class User
{
public int ID { get; set; }
public string UserName { get; set; }
public string Password { get; set; }
}
3.Create ApplicationDBContext:
public class ApplicationDBContext : DbContext
{
public ApplicationDBContext(DbContextOptions<ApplicationDBContext> options) : base(options)
{
}
public DbSet<User> Users { get; set; }
protected override void OnModelCreating(ModelBuilder modelBuilder)
{
modelBuilder.Entity<User>().ToTable("UserTB");
}
}
4.Register the ApplicationDBContext in the Program.cs file:
// Add services to the container.
var connectionString = builder.Configuration.GetConnectionString("DefaultConnection") ?? throw new InvalidOperationException("Connection string 'DefaultConnection' not found.");
builder.Services.AddDbContext<ApplicationDBContext>(options =>
options.UseSqlServer(connectionString));
builder.Services.AddDatabaseDeveloperPageExceptionFilter();
5.Open the appsettings.json
file and add a connection string as shown in the following:
{
"ConnectionStrings": {
"DefaultConnection": "Server=(localdb)\\mssqllocaldb;Database=ContosoUniversity1;Trusted_Connection=True;MultipleActiveResultSets=true"
},
"Logging": {
"LogLevel": {
"Default": "Information",
"Microsoft": "Warning",
"Microsoft.Hosting.Lifetime": "Information"
}
},
"AllowedHosts": "*"
}
- Execute the following commands (in the Package Manager Console) to migration and generate the database and datatable:
add-migration generatedatabase
update-database
use SSMS check the database, the user table already generated.
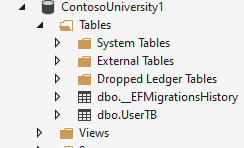
Then, you can create the relate pages to achieve the login functionality and inject the ApplicationDBContext to the controller or razor page and use it to access data in the database.
More detail information, you can refer to the following tutorial:
Tutorial: Get started with EF Core in an ASP.NET MVC web app
Razor Pages with Entity Framework Core in ASP.NET Core - Tutorial 1 of 8
If the answer is the right solution, please click "Accept Answer" and kindly upvote it. If you have extra questions about this answer, please click "Comment".
Note: Please follow the steps in our documentation to enable e-mail notifications if you want to receive the related email notification for this thread.
Best regards,
Dillion