I can not register with this code. I am encountering registration errors despite the notification popping up but i would get is error message and can't register, how to address issues with the server-side registration process or with how the form data is being handled in your server-side code.
This is in my register.cshtml.cs
// Licensed to the .NET Foundation under one or more agreements.
// The .NET Foundation licenses this file to you under the MIT license.
#nullable disable
using System;
using System.Collections.Generic;
using System.ComponentModel.DataAnnotations;
using System.Linq;
using System.Text;
using System.Text.Encodings.Web;
using System.Threading;
using System.Threading.Tasks;
using Microsoft.AspNetCore.Authentication;
using Microsoft.AspNetCore.Authorization;
using CDSWD_Inventory_System.Models;
using Microsoft.AspNetCore.Identity;
using Microsoft.AspNetCore.Identity.UI.Services;
using Microsoft.AspNetCore.Mvc;
using Microsoft.AspNetCore.Mvc.RazorPages;
using Microsoft.AspNetCore.WebUtilities;
using Microsoft.Extensions.Logging;
using Microsoft.AspNetCore.Mvc.ModelBinding.Validation;
using Microsoft.AspNetCore.Mvc.Rendering;
using CDSWD_Inventory_System.Repositories.Interfaces;
namespace CDSWD_Inventory_System.Areas.Identity.Pages.Account
{
public class RegisterModel : PageModel
{
private readonly SignInManager<ApplicationUser> _signInManager;
private readonly RoleManager<IdentityRole> _roleManager;
private readonly UserManager<ApplicationUser> _userManager;
private readonly IUserStore<ApplicationUser> _userStore;
private readonly IUserEmailStore<ApplicationUser> _emailStore;
private readonly ILogger<RegisterModel> _logger;
private readonly IEmailSender _emailSender;
private readonly IUnitOfWork _unitOfWork;
public RegisterModel(
UserManager<ApplicationUser> userManager,
IUserStore<ApplicationUser> userStore,
SignInManager<ApplicationUser> signInManager,
ILogger<RegisterModel> logger,
IEmailSender emailSender,
RoleManager<IdentityRole> roleManager,
IUnitOfWork unitOfWork)
{
_userManager = userManager;
_userStore = userStore;
_emailStore = GetEmailStore();
_signInManager = signInManager;
_logger = logger;
_emailSender = emailSender;
_roleManager = roleManager;
_unitOfWork = unitOfWork;
}
/// <summary>
/// This API supports the ASP.NET Core Identity default UI infrastructure and is not intended to be used
/// directly from your code. This API may change or be removed in future releases.
/// </summary>
[BindProperty]
public InputModel Input { get; set; }
/// <summary>
/// This API supports the ASP.NET Core Identity default UI infrastructure and is not intended to be used
/// directly from your code. This API may change or be removed in future releases.
/// </summary>
public string ReturnUrl { get; set; }
/// <summary>
/// This API supports the ASP.NET Core Identity default UI infrastructure and is not intended to be used
/// directly from your code. This API may change or be removed in future releases.
/// </summary>
public IList<AuthenticationScheme> ExternalLogins { get; set; }
/// <summary>
/// This API supports the ASP.NET Core Identity default UI infrastructure and is not intended to be used
/// directly from your code. This API may change or be removed in future releases.
/// </summary>
public class InputModel
{
/// <summary>
/// This API supports the ASP.NET Core Identity default UI infrastructure and is not intended to be used
/// directly from your code. This API may change or be removed in future releases.
/// </summary>
[Required]
[EmailAddress]
[Display(Name = "Email")]
public string Email { get; set; }
/// <summary>
/// This API supports the ASP.NET Core Identity default UI infrastructure and is not intended to be used
/// directly from your code. This API may change or be removed in future releases.
/// </summary>
[Required]
[StringLength(100, ErrorMessage = "The {0} must be at least {2} and at max {1} characters long.", MinimumLength = 6)]
[DataType(DataType.Password)]
[Display(Name = "Password")]
public string Password { get; set; }
/// <summary>
/// This API supports the ASP.NET Core Identity default UI infrastructure and is not intended to be used
/// directly from your code. This API may change or be removed in future releases.
/// </summary>
[DataType(DataType.Password)]
[Display(Name = "Confirm password")]
[Compare("Password", ErrorMessage = "The password and confirmation password do not match.")]
public string ConfirmPassword { get; set; }
public string? Role { get; set; }
[ValidateNever]
public IEnumerable<SelectListItem> RoleList { get; set; }
[Required]
public string FirstName { get; set; }
public string? MiddleName { get; set; }
public string? LastName { get; set; }
public string? Extension { get; set; }
public string? Barangay { get; set; }
public string? City { get; set; }
public string? PhoneNumber { get; set; }
public int? DepartmentId { get; set; }
[ValidateNever]
public IEnumerable<SelectListItem> DepartmentList { get; set; }
}
public async Task OnGetAsync(string returnUrl = null)
{
if (!_roleManager.RoleExistsAsync(SD.Role_Customer).GetAwaiter().GetResult())
{
_roleManager.CreateAsync(new IdentityRole(SD.Role_Customer)).GetAwaiter().GetResult();
_roleManager.CreateAsync(new IdentityRole(SD.Role_Admin)).GetAwaiter().GetResult();
_roleManager.CreateAsync(new IdentityRole(SD.Role_Enduser)).GetAwaiter().GetResult();
_roleManager.CreateAsync(new IdentityRole(SD.Role_Department)).GetAwaiter().GetResult();
}
Input = new()
{
RoleList = _roleManager.Roles.Select(x => x.Name).Select(i => new SelectListItem
{
Text = i,
Value = i
}),
DepartmentList = _unitOfWork.Department.GetAll().Select(i => new SelectListItem
{
Text = i.DepartmentName,
Value = i.DepartmentId.ToString()
})
};
ReturnUrl = returnUrl;
ExternalLogins = (await _signInManager.GetExternalAuthenticationSchemesAsync()).ToList();
}
public async Task<IActionResult> OnPostAsync(string returnUrl = null)
{
returnUrl ??= Url.Content("~/");
ExternalLogins = (await _signInManager.GetExternalAuthenticationSchemesAsync()).ToList();
if (ModelState.IsValid)
{
var user = CreateUser();
await _userStore.SetUserNameAsync(user, Input.Email, CancellationToken.None);
await _emailStore.SetEmailAsync(user, Input.Email, CancellationToken.None);
user.FirstName = Input.FirstName;
user.MiddleName = Input.MiddleName;
user.LastName = Input.LastName;
user.Extension = Input.Extension;
user.Barangay = Input.Barangay;
user.PhoneNumber = Input.PhoneNumber;
if (Input.Role == SD.Role_Department)
{
user.DepartmentId = Input.DepartmentId;
}
var result = await _userManager.CreateAsync(user, Input.Password);
if (result.Succeeded)
{
_logger.LogInformation("User created a new account with password.");
if (!String.IsNullOrEmpty(Input.Role))
{
await _userManager.AddToRoleAsync(user, Input.Role);
}
else
{
await _userManager.AddToRoleAsync(user, SD.Role_Customer);
}
// Set message to indicate success
ViewData["Message"] = "success";
var userId = await _userManager.GetUserIdAsync(user);
var code = await _userManager.GenerateEmailConfirmationTokenAsync(user);
code = WebEncoders.Base64UrlEncode(Encoding.UTF8.GetBytes(code));
var callbackUrl = Url.Page(
"/Account/ConfirmEmail",
pageHandler: null,
values: new { area = "Identity", userId = userId, code = code, returnUrl = returnUrl },
protocol: Request.Scheme);
await _emailSender.SendEmailAsync(Input.Email, "Confirm your email",
$"Please confirm your account by <a href='{HtmlEncoder.Default.Encode(callbackUrl)}'>clicking here</a>.");
if (_userManager.Options.SignIn.RequireConfirmedAccount)
{
return RedirectToPage("RegisterConfirmation", new { email = Input.Email, returnUrl = returnUrl });
}
else
{
await _signInManager.SignInAsync(user, isPersistent: false);
return LocalRedirect(returnUrl);
}
}
foreach (var error in result.Errors)
{
ModelState.AddModelError(string.Empty, error.Description);
}
}
// If we got this far, something failed, redisplay form
return Page();
}
private ApplicationUser CreateUser()
{
try
{
return Activator.CreateInstance<ApplicationUser>();
}
catch
{
throw new InvalidOperationException($"Can't create an instance of '{nameof(ApplicationUser)}'. " +
$"Ensure that '{nameof(ApplicationUser)}' is not an abstract class and has a parameterless constructor, or alternatively " +
$"override the register page in /Areas/Identity/Pages/Account/Register.cshtml");
}
}
private IUserEmailStore<ApplicationUser> GetEmailStore()
{
if (!_userManager.SupportsUserEmail)
{
throw new NotSupportedException("The default UI requires a user store with email support.");
}
return (IUserEmailStore<ApplicationUser>)_userStore;
}
}
}
and this is in my register.cshtml
@page
@model RegisterModel
@{
ViewData["Title"] = "Register";
}
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>@ViewData["Title"]</title>
<!-- Include Bootstrap CSS -->
<link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha1/dist/css/bootstrap.min.css">
<link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/sweetalert2@10">
<style>
body {
background-image: url('/assets/img/andrej-lisakov.jpg');
background-size: cover;
background-position: center;
margin: 0;
padding: 0;
min-height: 100vh;
display: flex;
justify-content: center;
align-items: center;
}
.container {
width: 80%; /* Adjust container width */
}
.card {
border-radius: 10px;
background-color: rgba(255, 255, 255, 0.8);
box-shadow: 0 0 10px rgba(0, 0, 0, 0.1);
}
.card-header {
background-color: #6c757d; /* Adjust header background color */
color: #fff;
border-top-left-radius: 10px;
border-top-right-radius: 10px;
font-size: 1.5rem; /* Reduce header font size */
padding: 0.75rem; /* Adjust header padding */
}
.form-label {
font-weight: bold;
}
.form-control {
border-radius: 8px; /* Elongate input fields */
}
/* Center align the form */
.card-body {
padding: 2rem; /* Adjust padding */
}
/* Center align the button */
#registerSubmit {
margin-top: 15px; /* Adjust the margin to center vertically */
width: 100%; /* Set button width to fill container */
font-weight: bold; /* Make button text bold */
}
</style>
</head>
<body>
<div class="container">
<div class="card shadow border-0 mt-4">
<div class="card-header bg-primary bg-gradient py-2">
<h4 class="text-white text-center mb-0">User Registration</h4>
</div>
<div class="card-body p-4">
<div class="row pt-0">
<div class="col-md-12">
<form id="registerForm" class="row" asp-route-returnUrl="@Model.ReturnUrl" method="post">
<h5 class="border-bottom pb-3 mb-4 text-secondary text-center">Create a new account</h5>
<div asp-validation-summary="ModelOnly" class="text-danger" role="alert"></div>
<div class="form-floating mb-3 col-md-12">
<input asp-for="Input.Email" class="form-control" autocomplete="username" aria-required="true" placeholder="Email" />
<label asp-for="Input.Email" class="ms-2 text-muted">Email</label>
<span asp-validation-for="Input.Email" class="text-danger"></span>
</div>
<div class="form-floating mb-3 col-md-6">
<input asp-for="Input.Password" class="form-control" autocomplete="new-password" aria-required="true" placeholder="password" />
<label asp-for="Input.Password" class="ms-2 text-muted">Password</label>
<span asp-validation-for="Input.Password" class="text-danger"></span>
</div>
<div class="form-floating mb-3 col-md-6">
<input asp-for="Input.ConfirmPassword" class="form-control" autocomplete="new-password" aria-required="true" placeholder="password" />
<label asp-for="Input.ConfirmPassword" class="ms-2 text-muted">Confirm Password</label>
<span asp-validation-for="Input.ConfirmPassword" class="text-danger"></span>
</div>
<div class="form-floating mb-3 col-md-6">
<input asp-for="Input.FirstName" class="form-control" placeholder="name@example.com" />
<label asp-for="Input.FirstName" class="ms-2 text-muted">First Name</label>
<span asp-validation-for="Input.FirstName" class="text-danger"></span>
</div>
<div class="form-floating mb-3 col-md-6">
<input asp-for="Input.MiddleName" class="form-control" placeholder="name@example.com" />
<label asp-for="Input.MiddleName" class="ms-2 text-muted">Middle Name</label>
<span asp-validation-for="Input.MiddleName" class="text-danger"></span>
</div>
<div class="form-floating mb-3 col-md-6">
<input asp-for="Input.LastName" class="form-control" placeholder="name@example.com" />
<label asp-for="Input.LastName" class="ms-2 text-muted">Last Name</label>
<span asp-validation-for="Input.LastName" class="text-danger"></span>
</div>
<div class="form-floating mb-3 col-md-6">
<input asp-for="Input.Extension" class="form-control" placeholder="name@example.com" />
<label asp-for="Input.Extension" class="ms-2 text-muted">Extension Name</label>
<span asp-validation-for="Input.Extension" class="text-danger"></span>
</div>
<div class="form-floating mb-3 col-md-6">
<input asp-for="Input.PhoneNumber" class="form-control" placeholder="name@example.com" />
<label asp-for="Input.PhoneNumber" class="ms-2 text-muted">Phone Number</label>
<span asp-validation-for="Input.PhoneNumber" class="text-danger"></span>
</div>
<div class="form-floating mb-3 col-md-6">
<input asp-for="Input.City" class="form-control" placeholder="name@example.com" />
<label asp-for="Input.City" class="ms-2 text-muted">City</label>
<span asp-validation-for="Input.City" class="text-danger"></span>
</div>
<div class="form-floating mb-3 col-md-6">
<input asp-for="Input.Barangay" class="form-control" placeholder="name@example.com" />
<label asp-for="Input.Barangay" class="ms-2 text-muted">Barangay</label>
<span asp-validation-for="Input.Barangay" class="text-danger"></span>
</div>
<div class="form-floating mb-3 col-md-6">
<select asp-for="Input.Role" asp-items="@Model.Input.RoleList" class="form-select">
<option disabled selected>-Select Role-</option>
</select>
</div>
<div class="form-floating mb-3 col-md-6 mx-auto">
<select asp-for="Input.DepartmentId" style="display:none;" asp-items="@Model.Input.DepartmentList" class="form-select">
<option disabled selected>-Select Department-</option>
</select>
</div>
<div class="d-flex justify-content-center">
<div class="col-md-5 col-s-10">
<button id="registerSubmit" type="submit" class="w-100 btn btn-primary">Register</button>
</div>
</div>
<br />
</form>
</div>
</div>
</div>
</div>
</div>
@section Scripts {
<partial name="_ValidationScriptsPartial" />
<!-- Include jQuery and SweetAlert -->
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
<script src="https://cdn.jsdelivr.net/npm/sweetalert2@10"></script>
<!-- JavaScript block -->
<script>
$(document).ready(function () {
// Hide/show department field based on role selection
$('#Input_Role').change(function () {
var selection = $('#Input_Role option:selected').text();
if (selection === 'Department') {
$('#Input_DepartmentId').show();
} else {
$('#Input_DepartmentId').hide();
}
});
// Handle form submission
$('#registerForm').submit(function (e) {
e.preventDefault(); // Prevent default form submission
var formData = new FormData($(this)[0]); // Get form data
// Perform AJAX request
$.ajax({
url: '/Account/Register',
method: 'POST',
data: formData,
processData: false,
contentType: false,
success: function (response, status, jqXHR) {
console.log(response); // Log the response for debugging
// Check if registration was successful
if (response && response.success) {
// Display success message using SweetAlert
Swal.fire({
title: 'Registration Successful',
icon: 'success',
showConfirmButton: true
}).then(function () {
// Redirect to the appropriate page
window.location.href = '/Home/Index';
});
} else {
// Display error message using SweetAlert
Swal.fire({
title: 'Registration Failed',
text: response && response.message ? response.message : 'An error occurred during registration.',
icon: 'error',
showConfirmButton: true
});
}
},
error: function (jqXHR, textStatus, errorThrown) {
// Log the error for debugging
console.error(textStatus, errorThrown);
// Display error message using SweetAlert
Swal.fire({
title: 'Registration Failed',
text: 'An error occurred during registration.',
icon: 'error',
showConfirmButton: true
});
}
});
});
});
</script>
}
</body>
</html>
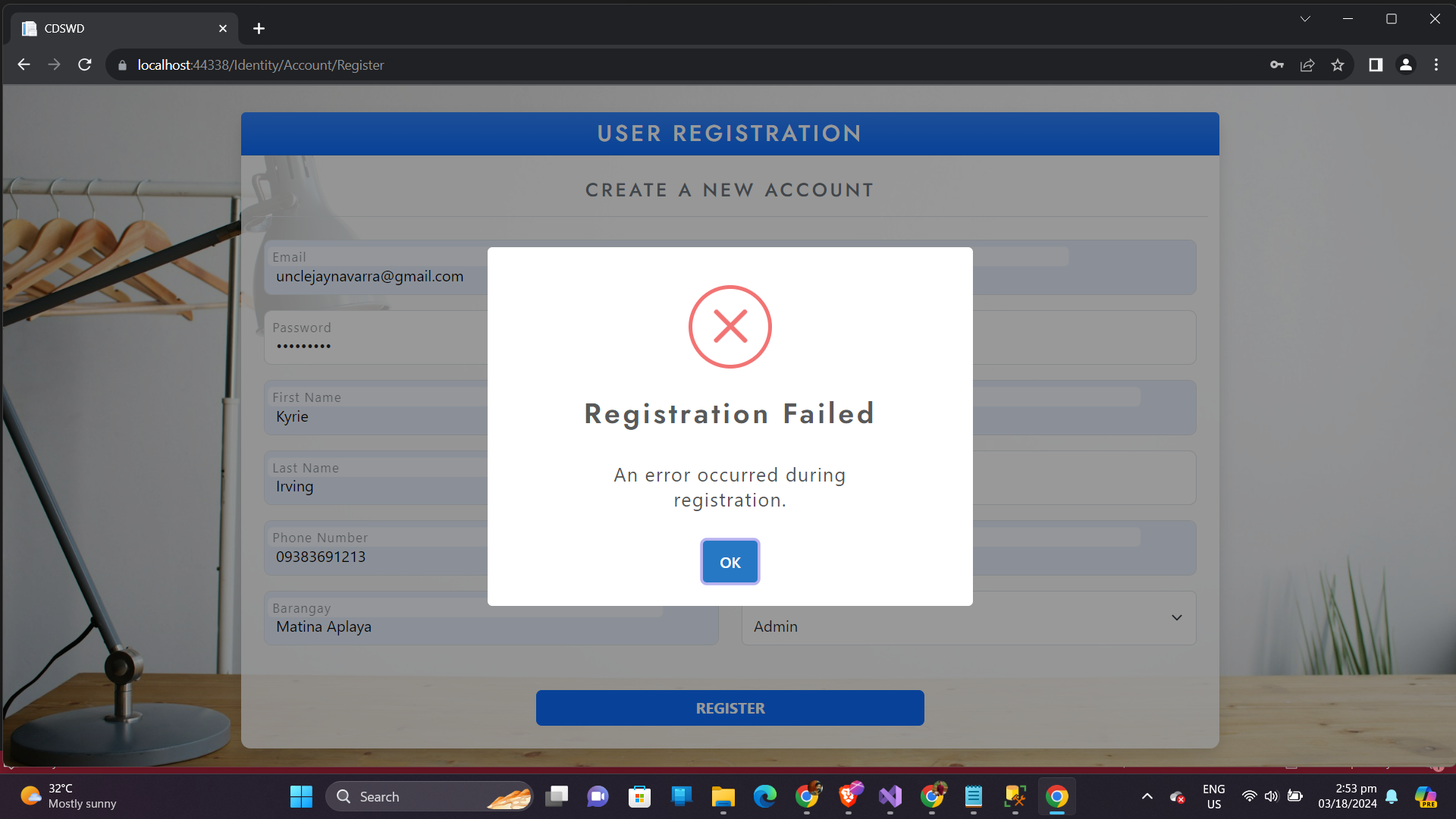
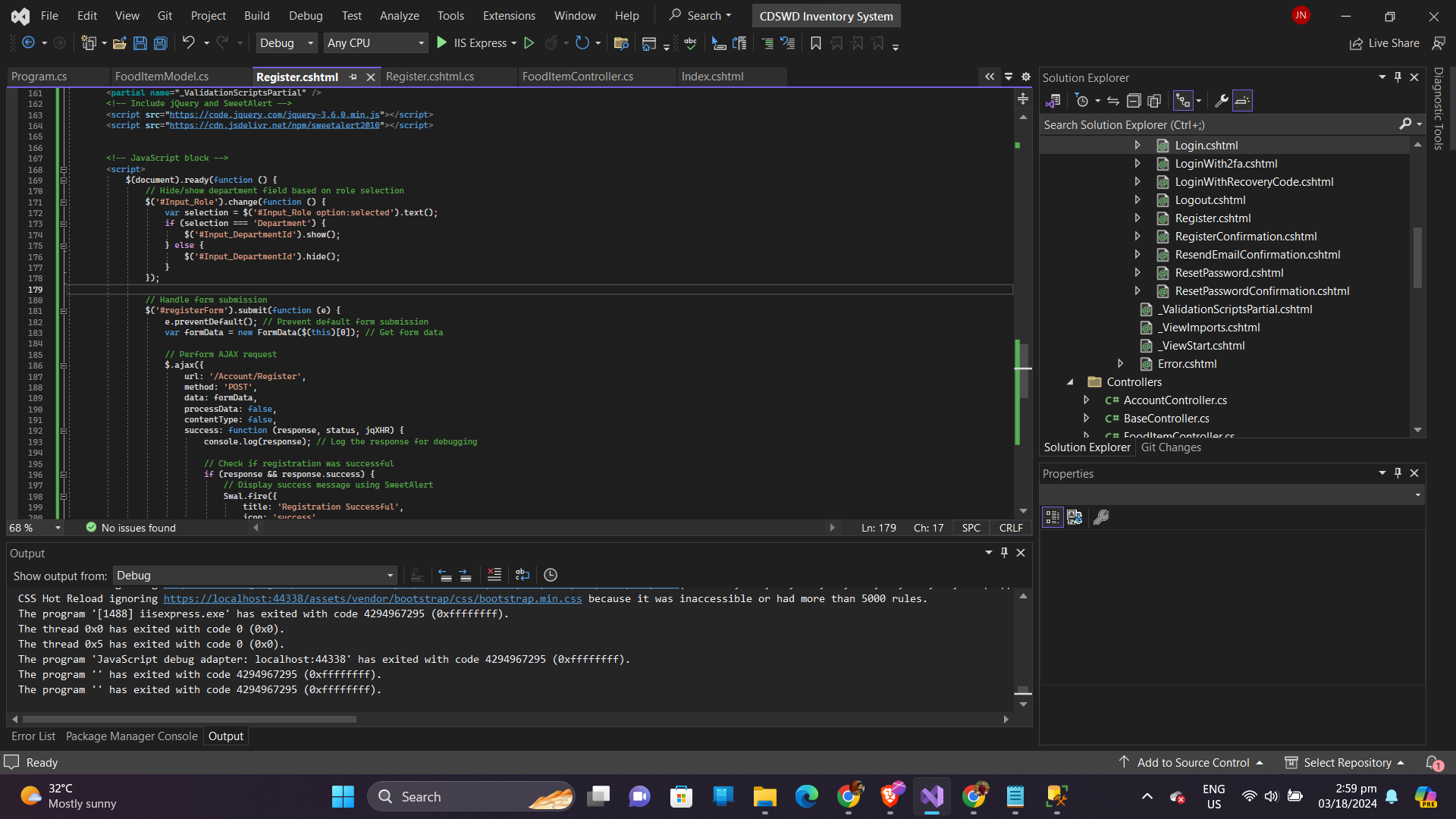
and as reference for my register page to appear the sweetalert is my login page.
Screenshot 2024-03-18 150750.png
how to implement the sweetalert to my register page be the same also as my login page as soon as i clicked the button.