You can see https://www.codeproject.com/Articles/67357/OpenGL-Oval
or from ChatGPT :
void DrawEllipse(float xCenter, float yCenter, float xRadius, float yRadius) {
int numSegments = 100; // The number of segments for the circle approximation
float angleStep = (2.0f * 3.14159f) / numSegments;
// Draw filled ellipse
glBegin(GL_TRIANGLE_FAN);
glVertex2f(xCenter, yCenter); // Center of the ellipse
for (int i = 0; i <= numSegments; i++) {
float angle = i * angleStep;
float x = xCenter + cosf(angle) * xRadius;
float y = yCenter + sinf(angle) * yRadius;
glVertex2f(x, y);
}
glEnd();
// Draw ellipse outline
glBegin(GL_LINE_LOOP);
for (int i = 0; i <= numSegments; i++) {
float angle = i * angleStep;
float x = xCenter + cosf(angle) * xRadius;
float y = yCenter + sinf(angle) * yRadius;
glVertex2f(x, y);
}
glEnd();
}
By testing in Nehe's Lesson 5 tutorial:
gluPerspective(45.0f,(GLfloat)width/(GLfloat)height,0.1f,100.0f);
replaced by
glOrtho(0.0f, width, height, 0.0f, -1.0f, 1.0f);
in DrawGLScene :
glColor3f(1.0f, 0.0f, 0.0f);
DrawEllipse(200.0f, 200.0f, 100.0f, 50.0f);
=>
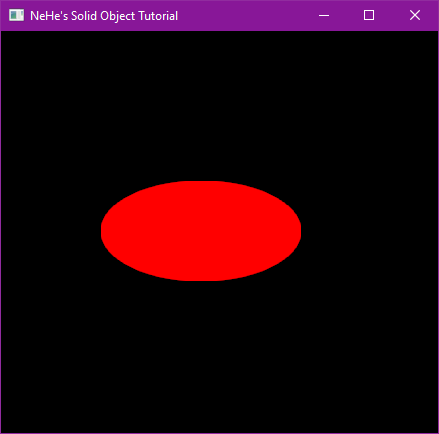