I can run the following query in Graph Explorer
https://graph.microsoft.com/v1.0/sites/546b0d08-4866-40fb-aa62-874af1bdeb54/pages
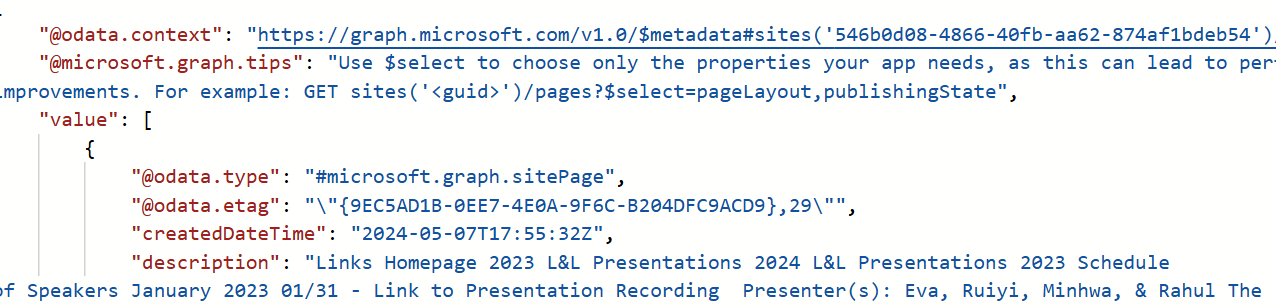
and in Python with
from msgraph.graph_service_client import GraphServiceClient
import asyncio
from azure.identity import AzureCliCredential, ChainedTokenCredential, ManagedIdentityCredential
managed_identity = ManagedIdentityCredential()
azure_cli = AzureCliCredential()
credentials = ChainedTokenCredential(managed_identity, azure_cli)
credentials = azure_cli
scopes = ['https://graph.microsoft.com/.default']
graph_client = GraphServiceClient(credentials, scopes)
MAIDAP_site_ID = '546b0d08-4866-40fb-aa62-874af1bdeb54'
async def get_site(site_id):
result = await graph_client.sites.by_site_id(site_id).get()
if result:
print(result)
return result
#This function run without permission issue
result_site = asyncio.run(get_site(MAIDAP_site_ID))
print(result_site)
which gives
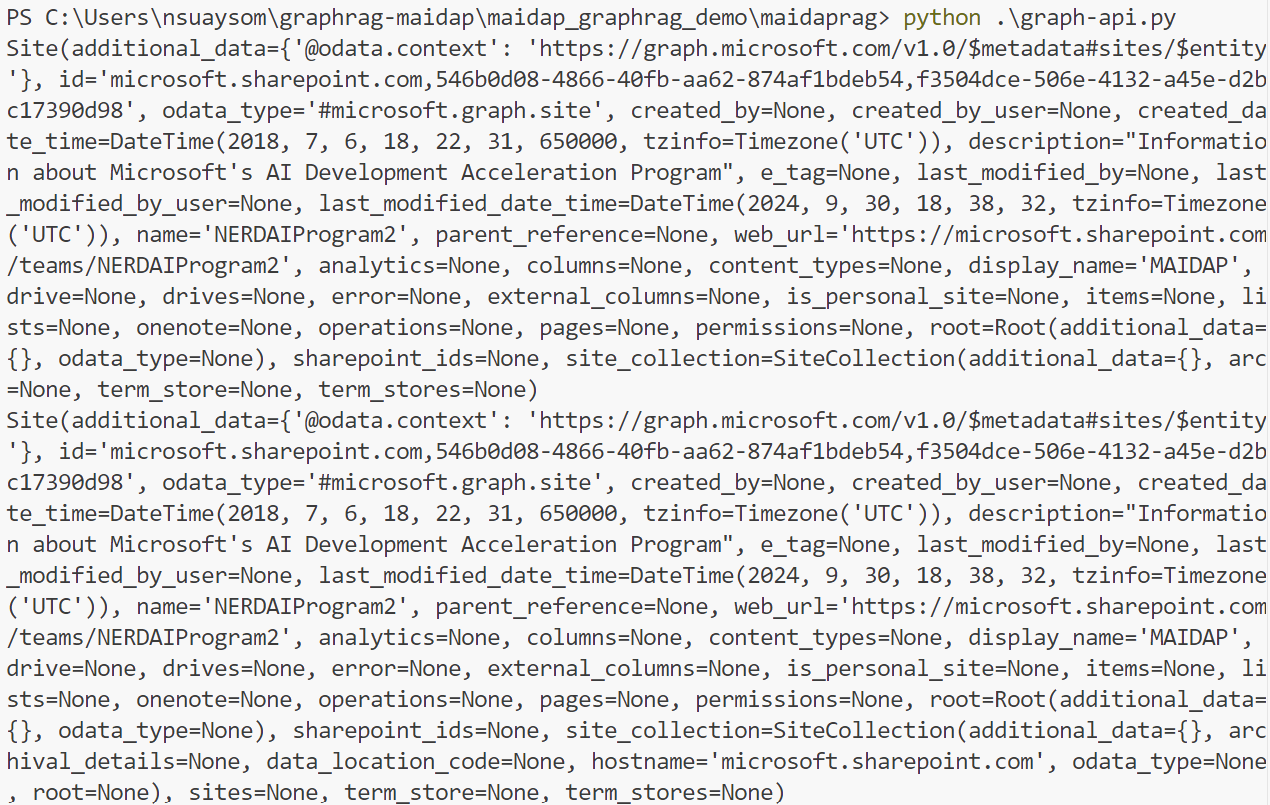
and that matches.
However, when running the following
https://graph.microsoft.com/v1.0/drives/b!CA1rVGZI-0CqYodK8b3rVM5NUPNuUDJBpF7SvBc5DZjZWxbIVPQ3SLHSrH4Y9T7E/items/01IXQQTLJO2NMT6LQSRJEKXVGXSZCXF4PM/children
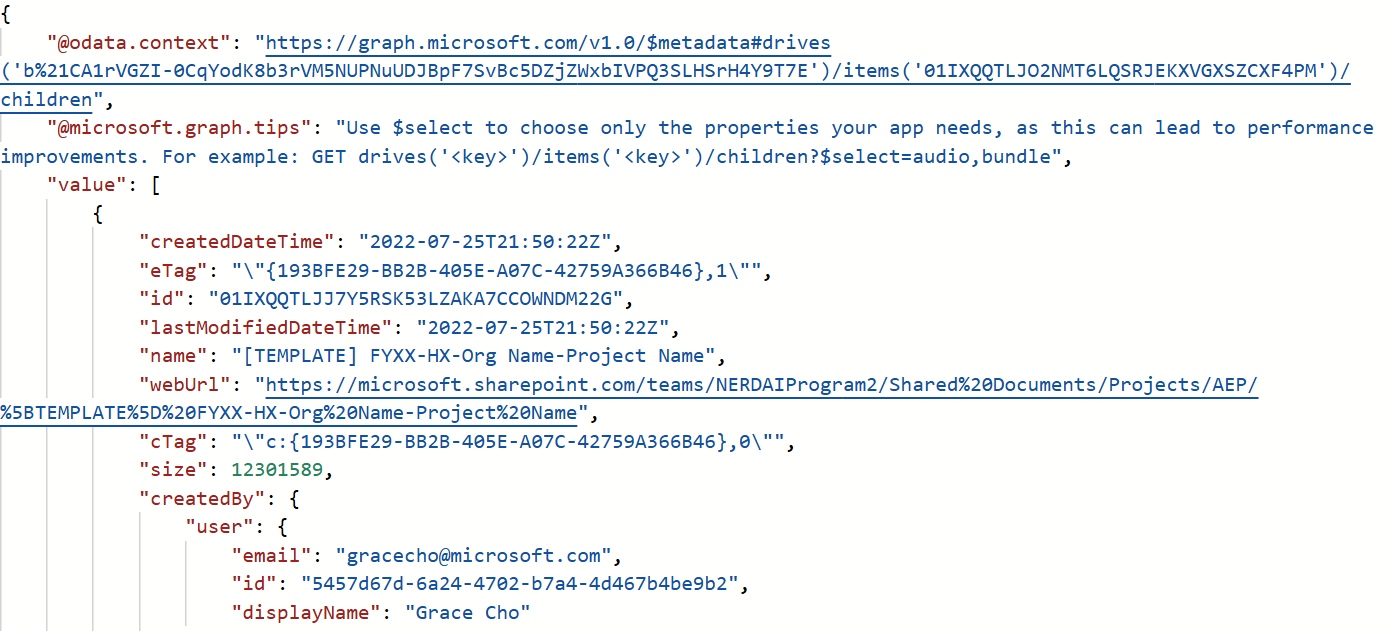
and get the correct results, however, when run in python with
from msgraph.graph_service_client import GraphServiceClientimport asynciofrom azure.identity import AzureCliCredential, ChainedTokenCredential, ManagedIdentityCredential
managed_identity = ManagedIdentityCredential()
azure_cli = AzureCliCredential()
credentials = ChainedTokenCredential(managed_identity, azure_cli)
credentials = azure_cli
scopes = ['https://graph.microsoft.com/.default']
graph_client = GraphServiceClient(credentials, scopes)
managed_identity = ManagedIdentityCredential()
azure_cli = AzureCliCredential()
credentials = ChainedTokenCredential(managed_identity, azure_cli)
credentials = azure_cli
scopes = ['https://graph.microsoft.com/.default']
Document_drive_ID = 'b!CA1rVGZI-0CqYodK8b3rVM5NUPNuUDJBpF7SvBc5DZjZWxbIVPQ3SLHSrH4Y9T7E'
Folder_item_ID = '01IXQQTLJO2NMT6LQSRJEKXVGXSZCXF4PM'
async def get_drive_item_children(drive_id, drive_item_id):
result = await graph_client.drives.by_drive_id(drive_id).items.by_drive_item_id(drive_item_id).children.get()
if result:
print(result)
return result
#This function gives us permission error
result_children = asyncio.run(get_drive_item_children(Document_drive_ID,Folder_item_ID))
print(result_children)
shown as follow
so I do have a permission in Graph Explorer, but not in Python for this query. Just wondering what's the best way to fix this issue?
