Hello everyone! I'm trying to implement the single sign-out into a client-side applications (React JS + @azure/msal-react) and I'm facing with some issues.
Before, lets ilustrate. When the logout is performed the Azure AD B2C open an iframe and send a HTTP POST request into my application, and the application should invalidade the session.
The flow works fine into a server-side applications (your cookie SameSite should be None)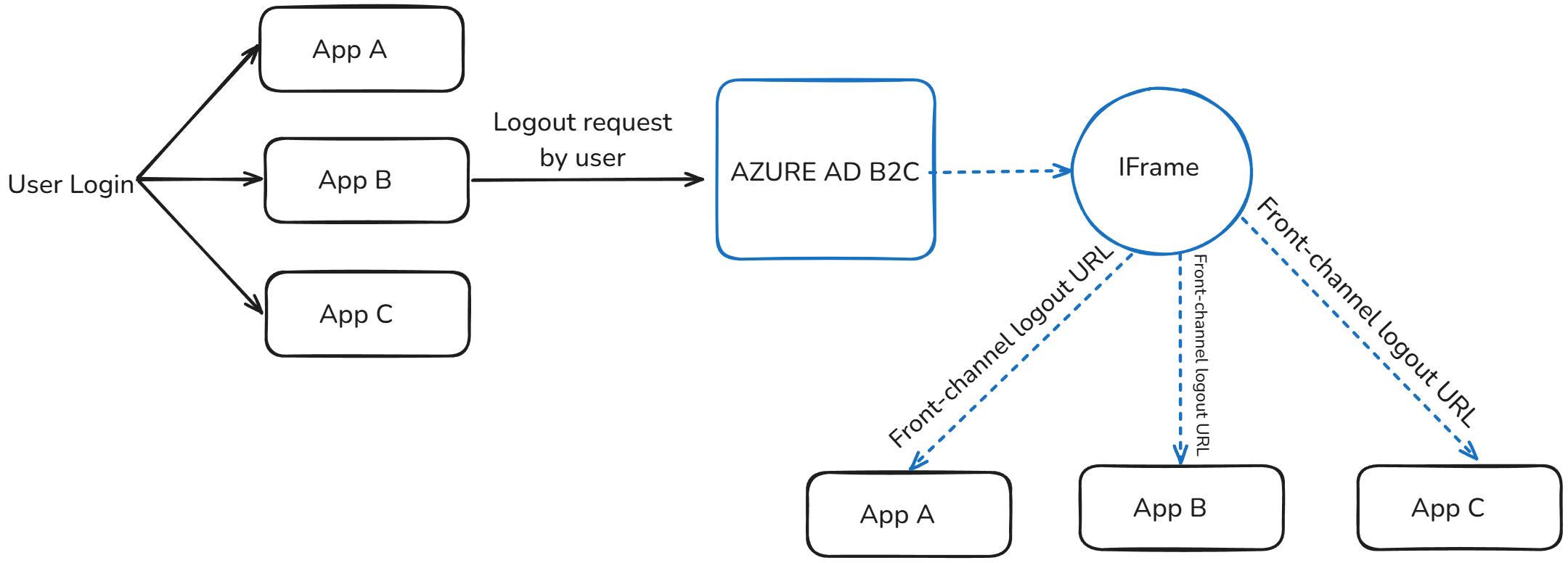
When one of my applications performs a logout, the Front-channel logout URL is called. However, in my client-side application, when the iframe tries to perform the logout or clear the user's cookies, it doesn't work because the iframe cannot access the domain cookies, which are blocked due to third-party cookie restrictions, keeping the user session active.
The problem still persists if the user navigates between routes or refreshes the page, because the session remains active in the cache manager.
Solutions I’ve Tried (None Worked):
I tried to implement a route, but it didn’t work.
WARNING: Skipping the server sign-out means the user's session will remain active on the server and can be signed back into your application without providing credentials again.
import React, { useEffect } from "react";
import { useMsal } from "@azure/msal-react";
import { BrowserUtils } from "@azure/msal-browser";
export function Logout() {
const { instance } = useMsal();
useEffect(() => {
instance.logoutRedirect({
account: instance.getActiveAccount(),
onRedirectNavigate: () => !BrowserUtils.isInIframe()
})
}, [ instance ]);
return (
<div>Logout</div>
)
}
The code above was found here
2. I tried to catch the events
I tried adding an event callback expecting to receive a logout or account removal event. Apparently, it's only used for managing the current application between browser tabs.
msalInstance.addEventCallback((message: EventMessage) => {
if (message.eventType === EventType.ACCOUNT_ADDED) {
// Update UI with new account
} else if (message.eventType === EventType.ACCOUNT_REMOVED) {
// Update UI with account logged out
} else if (message.eventType === EventType.ACTIVE_ACCOUNT_CHANGED) {
const accountInfo = msalInstance.getActiveAccount();
// Update UI with new active account info
}
});
This didn’t work either :/
Final Considerations
I tried modifying my custom policies to intercept the logout flow but without success.
Some info about my implementation:
- I use Custom Policies
- My profiles are correctly configured according to SSO recommendation here and here
- The full flow is working — all my applications can perform Single sign-in, and client-side applications can initiate Single sign-out.
If someone has solve the problem and can share the solution, I would be grateful ❤