Well, you can't really have an infinite sequence, since int
only has 32 bits. But you can go up to int.MaxValue
, using Enumerable.Range(0, int.MaxValue)
. There's no built-in function for this.
How can I enumerate an infinite sequence of integers in C#?
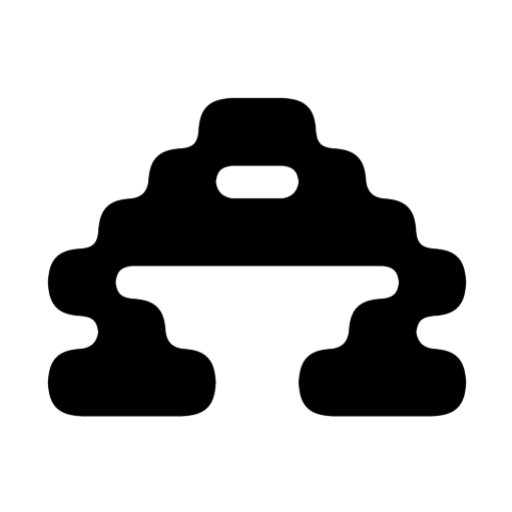
Is there a function in C# that returns an IEnumerator
of the infinite sequence of integers [0, 1, 2, 3, 4, 5 ...]
?
I'm currently doing
Enumerable.Range (0, 1000000000).Select (x => x * x).TakeWhile (x => (x <= limit))
to enumerate all squares up to limit
. I realize that this is effective, but if there's a built-in function that just counts up from 0
, I would prefer to use it.
3 answers
Sort by: Most helpful
-
Thomas Levesque 76 Reputation points MVP
2019-10-31T22:27:24.083+00:00 -
Kenneth Truyers 16 Reputation points
2019-10-31T22:13:20.217+00:00 Integers are not infinite, once you get to the end, it will overflow. So essentially the best you can do this:
Enumerable.Range (0, int.MaxValue).Select (x => x * x).TakeWhile (x => (x <= limit))
If you want larger ranges, you could choose different types:
Enumerable.Range (0, long.MaxValue).Select (x => x * x).TakeWhile (x => (x <= limit)) Enumerable.Range (0, double.MaxValue).Select (x => x * x).TakeWhile (x => (x <= limit))
Note that since you're squaring the values, the above samples would overflow at some point (namely at the square root of MaxValue).
-
Deleted
This answer has been deleted due to a violation of our Code of Conduct. The answer was manually reported or identified through automated detection before action was taken. Please refer to our Code of Conduct for more information.
Comments have been turned off. Learn more