Just allow the user to use their email as username. Trivial with a little javascript and a checkbox.
Being Able To Login Using UserName or Email address in ASP.NET Core MVC
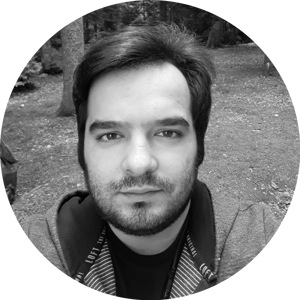
I want user to be able to login using UserName
or Email
in ASP.NET Core MVC. In case of using the [Required]
attribute, both the UserName
and the Email
must be entered in the model properties during login. While doing the form validation on the server-side, I need to perform model validation before accessing the data access layer in the application where the N-Tier architecture is implemented.
namespace LoginApplication.WebUI.Models
{
public class LoginModel
{
/// <summary>Which data annotation attribute should I use?</summary>
public string UserName { get; set; }
/// <summary>Which data annotation attribute should I use?</summary>
public string Email { get; set; }
[Required]
[DataType(DataType.Password)]
public string Password { get; set; }
}
}
namespace LoginApplication.WebUI.Controllers
{
public class AccountController : Controller
{
/* Other LOCs: Dependency Injection, "Login" Action HttpGet Method */
[HttpPost]
public async Task<IActionResult> Login(LoginModel model)
{
/* At this stage I need to validate the model correctly. */
if(ModelState.IsValid)
{
/* Other LOCs: User Query, Password Authentication, Page Redirection
Error Message Management, Log Management */
}
return View(model);
}
}
}
In this case, what attribute should I use defined in the System.ComponentModel.DataAnnotations
namespace? Or is there another way to work around this situation?
3 answers
Sort by: Most helpful
-
-
sipan1313 1 Reputation point
2022-02-08T13:40:02.483+00:00 -
Fahreddin Ekinci 0 Reputation points
2023-01-24T10:14:24.6266667+00:00 public class SignInViewModel { [Required] [Display(Name = "Kullanıcı Adı / Şifre")] public string Email { get; set; } [Required] [DataType(DataType.Password)] public string Password { get; set; } [Display(Name = "Seni Hatırlayalım mı ?")] public bool RememberMe { get; set; } }
[Route("/giris")] [Route("/login")] [HttpPost] [AllowAnonymous] [ValidateAntiForgeryToken] public async Task<IActionResult> Giris(SignInViewModel model, string returnUrl = null) { returnUrl = returnUrl ?? Url.Content("~/"); if (model.Email.IndexOf('@') > -1) { //E POSTA DOĞRULAMA string emailRegex = @"^([a-zA-Z0-9_\-\.]+)@((\[[0-9]{1,3}" + @"\.[0-9]{1,3}\.[0-9]{1,3}\.)|(([a-zA-Z0-9\-]+\" + @".)+))([a-zA-Z]{2,4}|[0-9]{1,3})(\]?)$"; Regex re = new Regex(emailRegex); if (!re.IsMatch(model.Email)) { ModelState.AddModelError("EPosta", "E-Posta doğrulanamadı"); } } else { //KULLANICI ADI DOĞRULAMA string emailRegex = @"^[a-zA-Z0-9]*$"; Regex re = new Regex(emailRegex); if (!re.IsMatch(model.Email)) { ModelState.AddModelError("EPosta", "kullanıcı adı doğrulanamadı"); } } if (ModelState.IsValid) { var userName = model.Email; if (userName.IndexOf('@') > -1) { var user = await _userManager.FindByEmailAsync(model.Email); if (user == null) { ModelState.AddModelError("", "değişik bir hata meydana geldi."); return View(model); } else { userName = user.UserName; } } var result = await _signInManager.PasswordSignInAsync(userName, model.Password, model.RememberMe, lockoutOnFailure: true);
hatalı giriş sonucu kullanıcı kilitleme aktif değilse
lockoutOnFailure false yapabilirsiniz.