How to reliably catch exceptions?
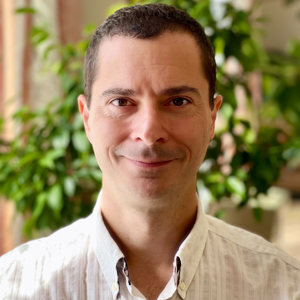
My app communicates with my server through a number of different endpoint, but they all go through the following method. I'm trying to catch exceptions when communicating to the server so I can convert them to an error response instead. When I run with a debug build this works fine, but when I run with a release build the catch
is never run. This isn't true for all exception handling in my code, but is try for this block. Does anyone know why this happens or have suggestions on how to fix it?
private async Task<HttpResponseMessage> PostAsync(string path, IMessage message)
{
var content = new StringContent(JsonConvert.SerializeObject(message), Encoding.UTF8, "application/json");
content.Headers.Add("x-functions-key", authKey);
try {
Console.WriteLine($"########## PostAsync A {path}");
var result = await client.PostAsync($"{ServiceUriBase}/{path}", content);
Console.WriteLine($"########## PostAsync B {path}");
return result;
} catch(Exception ex) {
Console.WriteLine($"########## {ex}");
return new HttpResponseMessage(System.Net.HttpStatusCode.BadRequest);
}
}
To clarify, with a release build, the PostAsync A
debug message is printed, but no other debug messages after that. So the code is not continuing successfully, and also not catching the exception. I do see a message in the console saying Unhandled managed exception...
, but that's my point; why is this unhandled? I'm trying to catch it.