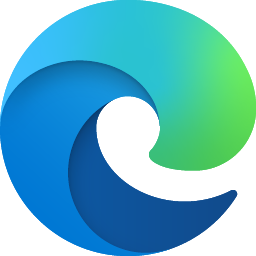
Collections data are stored in a SQLite database in the user's browser profile. The path of Collection file is like below:
C:\Users\UserName\AppData\Local\Microsoft\Edge\User Data\ProfileName\Collections\collectionsSQLite
When we open the SQLite database, we can see that the Collections urls are stored in items table, source column .
Then I think you can connect to the database and read data from it using C#. You can use package System.Data.SQLite. You can refer to this thread for related code.
Sample code:
using System;
using System.Text;
using System.Data;
using System.Data.SQLite;
namespace ConsoleApp1
{
class Program
{
static void Main(string[] args)
{
DataClass dc = new DataClass();
dc.selectQuery(@"SELECT source FROM items");
}
class DataClass
{
private SQLiteConnection sqlite;
public DataClass()
{
sqlite = new SQLiteConnection(@"Data Source=C:\Users\UserName\AppData\Local\Microsoft\Edge\User Data\Default\Collections\collectionsSQLite");
}
public DataTable selectQuery(string query)
{
DataTable dt = new DataTable();
SQLiteCommand selectCommand = new SQLiteCommand(query, sqlite);
try
{
sqlite.Open();
SQLiteDataReader reader = selectCommand.ExecuteReader();
while (reader.Read())
{
var url = reader.GetString(0);
Console.WriteLine(url);
}
sqlite.Close();
}
catch (SQLiteException e)
{
//Add your exception code here
}
return dt;
}
}
}
}
If the answer is the right solution, please click "Accept Answer" and kindly upvote it. If you have extra questions about this answer, please click "Comment".
Note: Please follow the steps in our documentation to enable e-mail notifications if you want to receive the related email notification for this thread.
Regards,
Yu Zhou