How to convert an API sample from c# to vb.net
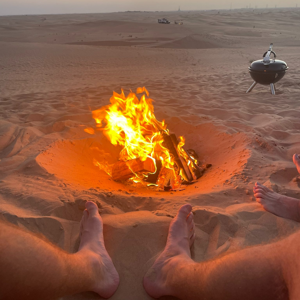
I'm using YouTube API v3 and using the endpoint Search:list I want to get the ID of the first video searched by a title. YouTube gives some code samples and this is one in c# that I'm trying to make it work in vb.net
using System;
using System.Collections.Generic;
using System.IO;
using System.Reflection;
using System.Threading;
using System.Threading.Tasks;
using Google.Apis.Auth.OAuth2;
using Google.Apis.Services;
using Google.Apis.Upload;
using Google.Apis.Util.Store;
using Google.Apis.YouTube.v3;
using Google.Apis.YouTube.v3.Data;
namespace Google.Apis.YouTube.Samples
{
/// <summary>
/// YouTube Data API v3 sample: search by keyword.
/// Relies on the Google APIs Client Library for .NET, v1.7.0 or higher.
/// See https://developers.google.com/api-client-library/dotnet/get_started
///
/// Set ApiKey to the API key value from the APIs & auth > Registered apps tab of
/// https://cloud.google.com/console
/// Please ensure that you have enabled the YouTube Data API for your project.
/// </summary>
internal class Search
{
[STAThread]
static void Main(string[] args)
{
Console.WriteLine("YouTube Data API: Search");
Console.WriteLine("========================");
try
{
new Search().Run().Wait();
}
catch (AggregateException ex)
{
foreach (var e in ex.InnerExceptions)
{
Console.WriteLine("Error: " + e.Message);
}
}
Console.WriteLine("Press any key to continue...");
Console.ReadKey();
}
private async Task Run()
{
var youtubeService = new YouTubeService(new BaseClientService.Initializer()
{
ApiKey = "REPLACE_ME",
ApplicationName = this.GetType().ToString()
});
var searchListRequest = youtubeService.Search.List("snippet");
searchListRequest.Q = "Google"; // Replace with your search term.
searchListRequest.MaxResults = 50;
// Call the search.list method to retrieve results matching the specified query term.
var searchListResponse = await searchListRequest.ExecuteAsync();
List<string> videos = new List<string>();
List<string> channels = new List<string>();
List<string> playlists = new List<string>();
// Add each result to the appropriate list, and then display the lists of
// matching videos, channels, and playlists.
foreach (var searchResult in searchListResponse.Items)
{
switch (searchResult.Id.Kind)
{
case "youtube#video":
videos.Add(String.Format("{0} ({1})", searchResult.Snippet.Title, searchResult.Id.VideoId));
break;
case "youtube#channel":
channels.Add(String.Format("{0} ({1})", searchResult.Snippet.Title, searchResult.Id.ChannelId));
break;
case "youtube#playlist":
playlists.Add(String.Format("{0} ({1})", searchResult.Snippet.Title, searchResult.Id.PlaylistId));
break;
}
}
Console.WriteLine(String.Format("Videos:\n{0}\n", string.Join("\n", videos)));
Console.WriteLine(String.Format("Channels:\n{0}\n", string.Join("\n", channels)));
Console.WriteLine(String.Format("Playlists:\n{0}\n", string.Join("\n", playlists)));
}
}
}
Using few online converters, I managed to get this vb.net code
Imports System
Imports System.Collections.Generic
Imports System.IO
Imports System.Reflection
Imports System.Threading
Imports System.Threading.Tasks
Imports Google.Apis.Auth.OAuth2
Imports Google.Apis.Services
Imports Google.Apis.Upload
Imports Google.Apis.Util.Store
Imports Google.Apis.YouTube.v3
Imports Google.Apis.YouTube.v3.Data
Public Class Form1
Private Async Sub Button1_Click(sender As Object, e As EventArgs) Handles Button1.Click
Try
Await Run()
Catch ex As AggregateException
For Each inner In ex.InnerExceptions
MsgBox("Error: " & inner.Message)
Next
End Try
End Sub
Private Async Function Run() As Task
Dim youtubeService = New YouTubeService(New BaseClientService.Initializer() With {
.ApiKey = "AIzaSyAzm9LUsClkwcEfgSD3wJhrIEPLC2rQkVw",
.ApplicationName = Me.[GetType]().ToString()
})
Dim searchListRequest = youtubeService.Search.List("snippet")
searchListRequest.Q = TextBox1.Text
searchListRequest.MaxResults = 1
Dim searchListResponse = Await searchListRequest.ExecuteAsync()
Dim videos As List(Of String) = New List(Of String)()
Dim channels As List(Of String) = New List(Of String)()
Dim playlists As List(Of String) = New List(Of String)()
For Each searchResult In searchListResponse.Items
videos.Add(String.Format("{0} ({1})", searchResult.Snippet.Title, searchResult.Id.VideoId))
Next
MsgBox(String.Format("Videos:" & vbLf & "{0}" & vbLf, String.Join(vbLf, videos)))
End Function
End Class
I setted a limit on searchListRequest.MaxResults to return me only 1 result, which means I want to get only the first searched video ID and searchListRequest.Q is connected to a textbox.
In this textbox I'll then write a qwery and I want that once I press the button, a msgbox will appear with the ID of the first searched video.
If now I want to add the video I've found to an existing playlist, I sow the endpoint playlist.list does the job, but how Am i able to implement it? There is no code sample in there. It just give me a requesto body. Do you think it would be possible to make it work both search a video and add it to a playlist? Thanks