ImageAttributes::GetAdjustedPalette method (gdiplusimageattributes.h)
The ImageAttributes::GetAdjustedPalette method adjusts the colors in a palette according to the adjustment settings of a specified category.
Syntax
Status GetAdjustedPalette(
[in, out] ColorPalette *colorPalette,
[in] ColorAdjustType colorAdjustType
);
Parameters
[in, out] colorPalette
Type: ColorPalette*
Pointer to a ColorPalette structure that on input, contains the palette to be adjusted and, on output, receives the adjusted palette.
[in] colorAdjustType
Type: ColorPalette
Element of the ColorAdjustType enumeration that specifies the category whose adjustment settings will be applied to the palette.
Return value
Type: Status
If the method succeeds, it returns Ok, which is an element of the Status enumeration.
If the method fails, it returns one of the other elements of the Status enumeration.
Remarks
An ImageAttributes object maintains color and grayscale settings for five adjustment categories: default, bitmap, brush, pen, and text. For example, you can specify a color-remap table for the default category, a different color-remap table for the bitmap category, and still a different color-remap table for the pen category.
When you call ImageAttributes::GetAdjustedPalette, you can specify the adjustment category that is used to adjust the palette colors. For example, if you pass ColorAdjustTypeBitmap to the ImageAttributes::GetAdjustedPalette method, then the adjustment settings of the bitmap category are used to adjust the palette colors.
Examples
The following example initializes a ColorPalette structure with four colors: aqua, black, red, and green. The code also creates an ImageAttributes object and sets its bitmap remap table so that green will be converted to blue. Then the code adjusts the palette colors by passing the address of the palette to the ImageAttributes::GetAdjustedPalette method of the ImageAttributes object. The code displays the four palette colors twice: once before the adjustment and once after the adjustment.
VOID Example_GetAdjustedPalette(HDC hdc)
{
Graphics graphics(hdc);
INT j;
// Create a palette that has four entries.
ColorPalette* palette =
(ColorPalette*)malloc(sizeof(ColorPalette) + 3 * sizeof(ARGB));
palette->Flags = 0;
palette->Count = 4;
palette->Entries[0] = 0xFF00FFFF; // aqua
palette->Entries[1] = 0xFF000000; // black
palette->Entries[2] = 0xFFFF0000; // red
palette->Entries[3] = 0xFF00FF00; // green
// Display the four palette colors with no adjustment.
SolidBrush brush(Color());
for(j = 0; j < 4; ++j)
{
brush.SetColor(palette->Entries[j]);
graphics.FillRectangle(&brush, 30*j, 0, 20, 20);
}
// Create a remap table that converts green to blue.
ColorMap map;
map.oldColor = Color(255, 0, 255, 0); // green
map.newColor = Color(255, 0, 0, 255); // blue
// Create an ImageAttributes object, and set its bitmap remap table.
ImageAttributes imAtt;
imAtt.SetRemapTable(1, &map, ColorAdjustTypeBitmap);
// Adjust the palette.
imAtt.GetAdjustedPalette(palette, ColorAdjustTypeBitmap);
// Display the four palette colors after the adjustment.
for(j = 0; j < 4; ++j)
{
brush.SetColor(palette->Entries[j]);
graphics.FillRectangle(&brush, 30*j, 30, 20, 20);
}
}
The following illustration shows the output of the preceding code. Note that the green in the original palette was changed to blue.
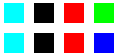
Requirements
Requirement | Value |
---|---|
Minimum supported client | Windows XP, Windows 2000 Professional [desktop apps only] |
Minimum supported server | Windows 2000 Server [desktop apps only] |
Target Platform | Windows |
Header | gdiplusimageattributes.h (include Gdiplus.h) |
Library | Gdiplus.lib |
DLL | Gdiplus.dll |
See also
Feedback
https://aka.ms/ContentUserFeedback.
Coming soon: Throughout 2024 we will be phasing out GitHub Issues as the feedback mechanism for content and replacing it with a new feedback system. For more information see:Submit and view feedback for