ImageAttributes::SetOutputChannel method (gdiplusimageattributes.h)
The ImageAttributes::SetOutputChannel method sets the CMYK output channel for a specified category.
Syntax
Status SetOutputChannel(
[in] ColorChannelFlags channelFlags,
[in, optional] ColorAdjustType type
);
Parameters
[in] channelFlags
Type: ColorChannelFlags
Element of the ColorChannelFlags enumeration that specifies the output channel.
[in, optional] type
Type: ColorAdjustType
Element of the ColorAdjustType enumeration that specifies the category for which the output channel is set. The default value is ColorAdjustTypeDefault.
Return value
Type: Status
If the method succeeds, it returns Ok, which is an element of the Status enumeration.
If the method fails, it returns one of the other elements of the Status enumeration.
Remarks
You can use the ImageAttributes::SetOutputChannel method to convert an image to a cyan-magenta-yellow-black (CMYK) color space and examine the intensities of one of the CMYK color channels. For example, suppose you create an ImageAttributes object and set its bitmap output channel to ColorChannelFlagsC. If you pass the address of that ImageAttributes object to the DrawImage method, the cyan component of each pixel is calculated, and each pixel in the rendered image is a shade of gray that indicates the intensity of its cyan channel. Similarly, you can render images that indicate the intensities of the magenta, yellow, and black channels.
An ImageAttributes object maintains color and grayscale settings for five adjustment categories: default, bitmap, brush, pen, and text. For example, you can specify an output channel for the default category and a different output channel for the bitmap category.
The default color- and grayscale-adjustment settings apply to all categories that don't have adjustment settings of their own. For example, if you never specify any adjustment settings for the bitmap category, then the default settings apply to the bitmap category.
As soon as you specify a color- or grayscale-adjustment setting for a certain category, the default adjustment settings no longer apply to that category. For example, suppose you specify a collection of adjustment settings for the default category. If you set the output channel for the bitmap category by passing ColorAdjustTypeBitmap to the ImageAttributes::SetOutputChannel method, then none of the default adjustment settings will apply to bitmaps.
Examples
The following example creates an Image object and calls the DrawImage method to draw the image. Then the code creates an ImageAttributes object and sets its bitmap output channel to cyan (ColorChannelFlagsC). The code calls DrawImage a second time, passing the address of the Image object and the address of the ImageAttributes object. The cyan channel of each pixel is calculated, and the rendered image shows the intensities of the cyan channel as shades of gray. The code calls DrawImage three more times to show the intensities of the magenta, yellow, and black channels.
VOID Example_SetOutputChannel(HDC hdc)
{
Graphics graphics(hdc);
Image image(L"Mosaic2.bmp");
// Draw the image unaltered.
graphics.DrawImage(&image, 10, 10, width, height);
UINT width = image.GetWidth();
UINT height = image.GetHeight();
ImageAttributes imAtt;
// Draw the image, showing the intensity of the cyan channel.
imAtt.SetOutputChannel(ColorChannelFlagsC, ColorAdjustTypeBitmap);
graphics.DrawImage(
&image,
Rect(110, 10, width, height), // dest rect
0, 0, width, height, // source rect
UnitPixel,
&imAtt);
// Draw the image, showing the intensity of the magenta channel.
imAtt.SetOutputChannel(ColorChannelFlagsM, ColorAdjustTypeBitmap);
graphics.DrawImage(
&image,
Rect(210, 10, width, height), // dest rect
0, 0, width, height, // source rect
UnitPixel,
&imAtt);
// Draw the image, showing the intensity of the yellow channel.
imAtt.SetOutputChannel(ColorChannelFlagsY, ColorAdjustTypeBitmap);
graphics.DrawImage(
&image,
Rect(10, 110, width, height), // dest rect
0, 0, width, height, // source rect
UnitPixel,
&imAtt);
// Draw the image, showing the intensity of the black channel.
imAtt.SetOutputChannel(ColorChannelFlagsK, ColorAdjustTypeBitmap);
graphics.DrawImage(
&image,
Rect(110, 110, width, height), // dest rect
0, 0, width, height, // source rect
UnitPixel,
&imAtt);
}
The following illustration shows the output of the preceding code.
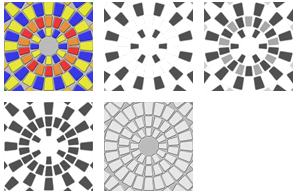
Requirements
Requirement | Value |
---|---|
Minimum supported client | Windows XP, Windows 2000 Professional [desktop apps only] |
Minimum supported server | Windows 2000 Server [desktop apps only] |
Target Platform | Windows |
Header | gdiplusimageattributes.h (include Gdiplus.h) |
Library | Gdiplus.lib |
DLL | Gdiplus.dll |
See also
ImageAttributes::ClearOutputChannel