Note
Access to this page requires authorization. You can try signing in or changing directories.
Access to this page requires authorization. You can try changing directories.
The ImageAttributes::SetThreshold method sets the threshold (transparency range) for a specified category.
Syntax
Status SetThreshold(
[in] REAL threshold,
[in, optional] ColorAdjustType type
);
Parameters
[in] threshold
Type: REAL
REAL number that specifies the threshold value.
[in, optional] type
Type: ColorAdjustType
Element of the ColorAdjustType enumeration that specifies the category for which the color threshold is set. The default value is ColorAdjustTypeDefault.
Return value
Type: Status
If the method succeeds, it returns Ok, which is an element of the Status enumeration.
If the method fails, it returns one of the other elements of the Status enumeration.
Remarks
The threshold is a value from 0 through 1 that specifies a cutoff point for each color component. For example, suppose the threshold is set to 0.7, and suppose you are rendering a color whose red, green, and blue components are 230, 50, and 220. The red component, 230, is greater than 0.7×255, so the red component will be changed to 255 (full intensity). The green component, 50, is less than 0.7×255, so the green component will be changed to 0. The blue component, 220, is greater than 0.7×255, so the blue component will be changed to 255.
An ImageAttributes object maintains color and grayscale settings for five adjustment categories: default, bitmap, brush, pen, and text. For example, you can specify a threshold for the default category, a threshold for the bitmap category, and still a different threshold for the pen category.
The default color- and grayscale-adjustment settings apply to all categories that don't have adjustment settings of their own. For example, if you never specify any adjustment settings for the pen category, then the default settings apply to the pen category.
As soon as you specify a color- or grayscale-adjustment setting for a certain category, the default adjustment settings no longer apply to that category. For example, suppose you specify a collection of adjustment settings for the default category. If you set the threshold for the pen category by passing ColorAdjustTypePen to the ImageAttributes::SetThreshold method, then none of the default adjustment settings will apply to pens.
Examples
The following example creates an Image object based on a .bmp file. The code also creates an ImageAttributes object and sets its bitmap threshold value to 0.6. Then the code draws the image twice: once with no color adjustment and once with the adjustment specified by the threshold.
VOID Example_SetThreshold(HDC hdc)
{
Graphics graphics(hdc);
// Create an Image object based on a .bmp file.
// The image has one stripe with RGB components (160, 0, 0)
// and one stripe with RGB components (0, 140, 0).
Image image(L"RedGreenThreshold.bmp");
// Create an ImageAttributes object, and set its bitmap threshold to 0.6.
ImageAttributes imAtt;
imAtt.SetThreshold(0.6f, ColorAdjustTypeBitmap);
// Draw the image with no color adjustment.
graphics.DrawImage(&image, 10, 10, image.GetWidth(), image.GetHeight());
// Draw the image with the threshold applied.
// 160 > 0.6*255, so the red stripe will be changed to full intensity.
// 140 < 0.6*255, so the green stripe will be changed to zero intensity.
graphics.DrawImage(&image,
Rect(100, 10, image.GetWidth(), image.GetHeight()), // dest rect
0, 0, image.GetWidth(), image.GetHeight(), // source rect
UnitPixel,
&imAtt);
}
The following illustration shows the output of the preceding code. Note that the red was converted to maximum intensity, and the green was converted to zero intensity.
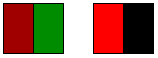
Requirements
Requirement | Value |
---|---|
Minimum supported client | Windows XP, Windows 2000 Professional [desktop apps only] |
Minimum supported server | Windows 2000 Server [desktop apps only] |
Target Platform | Windows |
Header | gdiplusimageattributes.h (include Gdiplus.h) |
Library | Gdiplus.lib |
DLL | Gdiplus.dll |
See also
ImageAttributes::ClearColorKey