ID2D1RenderTarget::D rawRectangle(constD2D1_RECT_F&,ID2D1Brush*,FLOAT,ID2D1StrokeStyle*) (d2d1.h)
Dibuja el contorno de un rectángulo que tiene las dimensiones y el estilo de trazo especificados.
Sintaxis
void DrawRectangle(
const D2D1_RECT_F & rect,
ID2D1Brush *brush,
FLOAT strokeWidth,
ID2D1StrokeStyle *strokeStyle
);
Parámetros
rect
Tipo: [in] const D2D1_RECT_F &
Dimensiones del rectángulo que se van a dibujar, en píxeles independientes del dispositivo.
brush
Tipo: [in] ID2D1Brush*
Pincel usado para pintar el trazo del rectángulo.
strokeWidth
Tipo: [in] FLOAT
Ancho del trazo, en píxeles independientes del dispositivo. El valor debe ser mayor o igual que 0,0f. Si no se especifica este parámetro, el valor predeterminado es 1.0f. El trazo se centra en la línea.
strokeStyle
Tipo: [in, opcional] ID2D1StrokeStyle*
Estilo de trazo que se va a pintar o NULL para pintar un trazo sólido.
Valor devuelto
None
Observaciones
Cuando se produce un error en este método, no devuelve un código de error. Para determinar si se produjo un error en un método de dibujo (como DrawRectangle), compruebe el resultado devuelto por el método ID2D1RenderTarget::EndDraw o ID2D1RenderTarget::Flush .
Ejemplos
En el ejemplo siguiente se usa un ID2D1HwndRenderTarget para dibujar y rellenar varios rectángulos. En este ejemplo se genera la salida que se muestra en la ilustración siguiente.
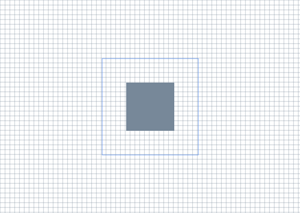
// This method discards device-specific
// resources if the Direct3D device disappears during execution and
// recreates the resources the next time it's invoked.
HRESULT DemoApp::OnRender()
{
HRESULT hr = S_OK;
hr = CreateDeviceResources();
if (SUCCEEDED(hr))
{
m_pRenderTarget->BeginDraw();
m_pRenderTarget->SetTransform(D2D1::Matrix3x2F::Identity());
m_pRenderTarget->Clear(D2D1::ColorF(D2D1::ColorF::White));
D2D1_SIZE_F rtSize = m_pRenderTarget->GetSize();
// Draw a grid background.
int width = static_cast<int>(rtSize.width);
int height = static_cast<int>(rtSize.height);
for (int x = 0; x < width; x += 10)
{
m_pRenderTarget->DrawLine(
D2D1::Point2F(static_cast<FLOAT>(x), 0.0f),
D2D1::Point2F(static_cast<FLOAT>(x), rtSize.height),
m_pLightSlateGrayBrush,
0.5f
);
}
for (int y = 0; y < height; y += 10)
{
m_pRenderTarget->DrawLine(
D2D1::Point2F(0.0f, static_cast<FLOAT>(y)),
D2D1::Point2F(rtSize.width, static_cast<FLOAT>(y)),
m_pLightSlateGrayBrush,
0.5f
);
}
// Draw two rectangles.
D2D1_RECT_F rectangle1 = D2D1::RectF(
rtSize.width/2 - 50.0f,
rtSize.height/2 - 50.0f,
rtSize.width/2 + 50.0f,
rtSize.height/2 + 50.0f
);
D2D1_RECT_F rectangle2 = D2D1::RectF(
rtSize.width/2 - 100.0f,
rtSize.height/2 - 100.0f,
rtSize.width/2 + 100.0f,
rtSize.height/2 + 100.0f
);
// Draw a filled rectangle.
m_pRenderTarget->FillRectangle(&rectangle1, m_pLightSlateGrayBrush);
// Draw the outline of a rectangle.
m_pRenderTarget->DrawRectangle(&rectangle2, m_pCornflowerBlueBrush);
hr = m_pRenderTarget->EndDraw();
}
if (hr == D2DERR_RECREATE_TARGET)
{
hr = S_OK;
DiscardDeviceResources();
}
return hr;
}
Para ver un tutorial relacionado, consulta Creación de una aplicación direct2D sencilla.
Requisitos
Requisito | Value |
---|---|
Cliente mínimo compatible | Windows 7, Windows Vista con SP2 y Platform Update para Windows Vista [aplicaciones de escritorio | Aplicaciones para UWP] |
Servidor mínimo compatible | Windows Server 2008 R2, Windows Server 2008 con SP2 y Actualización de plataforma para Windows Server 2008 [aplicaciones de escritorio | Aplicaciones para UWP] |
Plataforma de destino | Windows |
Encabezado | d2d1.h |
Library | D2d1.lib |
Archivo DLL | D2d1.dll |
Consulte también
Creación de aplicación Direct2D simpl
Comentarios
https://aka.ms/ContentUserFeedback.
Próximamente: A lo largo de 2024 iremos eliminando gradualmente GitHub Issues como mecanismo de comentarios sobre el contenido y lo sustituiremos por un nuevo sistema de comentarios. Para más información, vea:Enviar y ver comentarios de