ImageAttributes::SetOutputChannelColorProfile メソッド (gdiplusimageattributes.h)
ImageAttributes::SetOutputChannelColorProfile メソッドは、指定したカテゴリの出力チャネルカラー プロファイル ファイルを設定します。
構文
Status SetOutputChannelColorProfile(
[in] const WCHAR *colorProfileFilename,
[in, optional] ColorAdjustType type
);
パラメーター
[in] colorProfileFilename
型: const WCHAR*
カラー プロファイル ファイルのパス名。 カラー プロファイル ファイルが %SystemRoot%\System32\Spool\Drivers\Color ディレクトリにある場合は、このパラメーターにファイル名を指定できます。 それ以外の場合、このパラメーターは、完全修飾パス名を指定する必要があります。
[in, optional] type
種類: ColorAdjustType
出力チャネルカラー プロファイル ファイルが設定されるカテゴリを指定する ColorAdjustType 列挙体の要素。 既定値は ColorAdjustTypeDefault です。
戻り値
種類: 状態
メソッドが成功した場合は、Status 列挙の要素である Ok を返します。
メソッドが失敗した場合は、 Status 列挙体の他の要素のいずれかを返します。
注釈
ImageAttributes::SetOutputChannel メソッドと ImageAttributes::SetOutputChannelColorProfile メソッドを使用して、イメージをシアンマゼンタイエローブラック (CMYK) 色空間に変換し、CMYK カラー チャネルの 1 つの強度を調べることができます。 たとえば、次の手順を実行するコードを記述するとします。
- Image オブジェクトを作成します。
- ImageAttributes オブジェクトを作成します。
- ColorChannelFlagsC を ImageAttributes オブジェクトの ImageAttributes::SetOutputChannel メソッドに渡します。
- カラー プロファイル ファイルのパス名を ImageAttributes オブジェクトの ImageAttributes::SetOutputChannelColorProfile メソッドに渡します。
- Image オブジェクトと ImageAttributes オブジェクトのアドレスを DrawImage メソッドに渡します。
ImageAttributes オブジェクトは、既定、ビットマップ、ブラシ、ペン、テキストの 5 つの調整カテゴリの色とグレースケールの設定を維持します。 たとえば、既定のカテゴリに出力チャネルカラープロファイルファイルを指定し、ビットマップカテゴリに別の出力チャンネルカラープロファイルファイルを指定できます。
既定の色調整とグレースケール調整設定は、独自の調整設定を持たないすべてのカテゴリに適用されます。 たとえば、ビットマップ カテゴリの調整設定を指定しない場合、既定の設定はビットマップ カテゴリに適用されます。
特定のカテゴリに対して色調整またはグレースケール調整設定を指定するとすぐに、既定の調整設定がそのカテゴリに適用されなくなります。 たとえば、既定のカテゴリの調整設定のコレクションを指定するとします。 ColorAdjustTypeBitmap を ImageAttributes::SetOutputChannelColorProfile メソッドに渡してビットマップ カテゴリの出力チャネルカラー プロファイル ファイルを設定した場合、既定の調整設定はビットマップに適用されません。
例
次の例では、 Image オブジェクトを作成し、 DrawImage メソッドを呼び出してイメージを描画します。 次に、 ImageAttributes オブジェクトを作成し、 ImageAttributes::SetOutputChannelColorProfile メソッドを呼び出してビットマップ カテゴリのプロファイル ファイルを指定します。 ImageAttributes::SetOutputChannel の呼び出しにより、出力チャネル (ビットマップ カテゴリの場合) がシアンに設定されます。 このコードは、Image オブジェクトのアドレスと ImageAttributes オブジェクトのアドレスを渡して、DrawImage を 2 回目に呼び出します。 各ピクセルのシアンチャネルが計算され、レンダリングされた画像はシアンチャネルの強度を灰色の網掛けとして示します。 このコードでは 、さらに DrawImage を 3 回呼び出して、マゼンタ、黄色、黒のチャネルの強度を示します。
VOID Example_SetOutputProfile(HDC hdc)
{
Graphics graphics(hdc);
Image image(L"Mosaic2.bmp");
ImageAttributes imAtt;
UINT width = image.GetWidth();
UINT height = image.GetHeight();
// Draw the image unaltered.
graphics.DrawImage(&image, 10, 10, width, height);
imAtt.SetOutputChannelColorProfile(
L"TEKPH600.ICM", ColorAdjustTypeBitmap);
// Draw the image, showing the intensity of the cyan channel.
imAtt.SetOutputChannel(ColorChannelFlagsC, ColorAdjustTypeBitmap);
graphics.DrawImage(
&image,
Rect(110, 10, width, height), // dest rect
0, 0, width, height, // source rect
UnitPixel,
&imAtt);
// Draw the image, showing the intensity of the magenta channel.
imAtt.SetOutputChannel(ColorChannelFlagsM, ColorAdjustTypeBitmap);
graphics.DrawImage(
&image,
Rect(210, 10, width, height), // dest rect
0, 0, width, height, // source rect
UnitPixel,
&imAtt);
// Draw the image, showing the intensity of the yellow channel.
imAtt.SetOutputChannel(ColorChannelFlagsY, ColorAdjustTypeBitmap);
graphics.DrawImage(
&image,
Rect(10, 110, width, height), // dest rect
0, 0, width, height, // source rect
UnitPixel,
&imAtt);
// Draw the image, showing the intensity of the black channel.
imAtt.SetOutputChannel(ColorChannelFlagsK, ColorAdjustTypeBitmap);
graphics.DrawImage(
&image,
Rect(110, 110, width, height), // dest rect
0, 0, width, height, // source rect
UnitPixel,
&imAtt);
}
上記のコードは、Mosaic2.bmp ファイルと Tekph600.icm と共に、次の出力を生成しました。
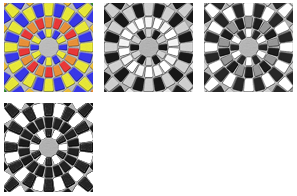
要件
要件 | 値 |
---|---|
サポートされている最小のクライアント | Windows XP、Windows 2000 Professional [デスクトップ アプリのみ] |
サポートされている最小のサーバー | Windows 2000 Server [デスクトップ アプリのみ] |
対象プラットフォーム | Windows |
ヘッダー | gdiplusimageattributes.h (Gdiplus.h を含む) |
Library | Gdiplus.lib |
[DLL] | Gdiplus.dll |
こちらもご覧ください
ImageAttributes::ClearOutputChannel
フィードバック
https://aka.ms/ContentUserFeedback」を参照してください。
以下は間もなく提供いたします。2024 年を通じて、コンテンツのフィードバック メカニズムとして GitHub の issue を段階的に廃止し、新しいフィードバック システムに置き換えます。 詳細については、「フィードバックの送信と表示