進行状況インジケーター — MRTK2
シーンの例
進行状況インジケーターの使用方法の例は、ProgressIndicatorExamples
シーンで確認できます。 このシーンでは、SDK に含まれる進行状況インジケーター プレハブがそれぞれ示されます。 また、シーン読み込みなどの一般的な非同期タスクと共に、進行状況インジケーターを使用する方法も示されます。
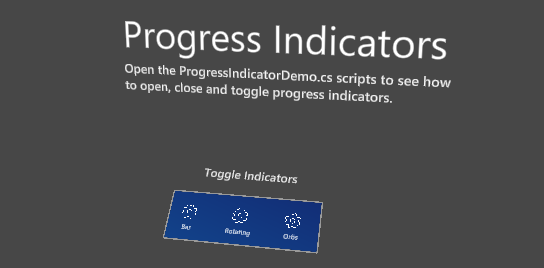
例: 進行状況インジケーターを開く、更新 & 閉じる
進行状況インジケーターには、IProgressIndicator
インターフェイスが実装されます。 このインターフェイスは、GetComponent
を使用して、GameObject から取得できます。
[SerializedField]
private GameObject indicatorObject;
private IProgressIndicator indicator;
private void Start()
{
indicator = indicatorObject.GetComponent<IProgressIndicator>();
}
IProgressIndicator.OpenAsync()
および IProgressIndicator.CloseAsync()
メソッドは Tasks を返します。 非同期メソッドでは、これらの Tasks を待機することをお勧めします。
MRTK の既定の進行状況インジケーター プレハブは、シーンに配置すると非アクティブになります。
IProgressIndicator.OpenAsync()
メソッドが呼び出されると、進行状況インジケーターでその GameObject が自動的にアクティブ化および非アクティブ化されます (このパターンは、IProgressIndicator インターフェイスの要件ではありません)。
インジケーターの Progress
プロパティを 0 から 1 の値に設定して、表示される進行状況を更新します。 その Message
プロパティを設定して、表示されるメッセージを更新します。 実装が異なると、このコンテンツがさまざまな方法で表示される可能性があります。
private async void OpenProgressIndicator()
{
await indicator.OpenAsync();
float progress = 0;
while (progress < 1)
{
progress += Time.deltaTime;
indicator.Message = "Loading...";
indicator.Progress = progress;
await Task.Yield();
}
await indicator.CloseAsync();
}
インジケーターの状態
インジケーターの State
プロパティによって、有効な操作が決定されます。 無効なメソッドを呼び出すと、通常、インジケーターからエラーが報告され、アクションが実行されることはありません。
State | 有効な操作 |
---|---|
ProgressIndicatorState.Opening |
AwaitTransitionAsync() |
ProgressIndicatorState.Open |
CloseAsync() |
ProgressIndicatorState.Closing |
AwaitTransitionAsync() |
ProgressIndicatorState.Closed |
OpenAsync() |
使用する前に、インジケーターが完全に開かれているか、閉じられていることを確認するには、AwaitTransitionAsync()
を使用できます。
private async void ToggleIndicator(IProgressIndicator indicator)
{
await indicator.AwaitTransitionAsync();
switch (indicator.State)
{
case ProgressIndicatorState.Closed:
await indicator.OpenAsync();
break;
case ProgressIndicatorState.Open:
await indicator.CloseAsync();
break;
}
}