ImageAttributes::ClearColorMatrices 方法 (gdiplusimageattributes.h)
ImageAttributes::ClearColorMatrices 方法清除指定类别的颜色调整矩阵和灰度调整矩阵。
语法
Status ClearColorMatrices(
[in, optional] ColorAdjustType type
);
parameters
[in, optional] type
类型: ColorAdjustType
ColorAdjustType 枚举的元素,该元素指定清除调整矩阵的类别。 默认值为 ColorAdjustTypeDefault。
返回值
类型: 状态
如果该方法成功,则返回 Ok,这是 Status 枚举的元素。
如果方法失败,它将返回 Status 枚举的其他元素之一。
注解
ImageAttributes 对象维护五个调整类别的颜色和灰度设置:默认、位图、画笔、笔和文本。 例如,可以为默认类别指定一对 (颜色和灰度) 调整矩阵,为位图类别指定另一对调整矩阵,为笔类别指定另一对调整矩阵。
默认的颜色和灰度调整设置适用于没有自己调整设置的所有类别。 例如,如果从未为笔类别指定任何调整设置,则默认设置适用于笔类别。
为特定类别指定颜色或灰度调整设置后,默认调整设置将不再应用于该类别。 例如,假设指定一对 (调整矩阵的颜色和灰度) ,并为默认类别指定一个伽玛值。 如果通过调用 ImageAttributes::SetColorMatrices 为笔类别设置一对调整矩阵,则默认调整矩阵将不适用于笔。 如果稍后通过调用 ImageAttributes::ClearColorMatrices 清除笔调整矩阵,则笔类别不会还原默认调整矩阵;而笔类别将没有调整矩阵。 同样,笔类别不会还原默认伽玛值;相反,笔类别将没有伽玛值。
示例
以下示例从 .emf 文件创建 Image 对象。 该代码还会创建 ImageAttributes 对象。 首次调用 ImageAttributes::SetColorMatrices 设置 ImageAttributes 对象的默认颜色调整矩阵和默认灰度调整矩阵。 第二次调用 ImageAttributes::SetColorMatrices 设置 ImageAttributes 对象的笔颜色调整矩阵和笔灰度调整矩阵。 这四个矩阵的执行方式如下:
- 默认颜色:将红色分量乘以 1.5。
- 默认灰度:将绿色分量乘以 1.5。
- 笔颜色:将蓝色分量乘以 1.5。
- 笔灰度:将红色、绿色和蓝色分量乘以 1.5。
代码调用 DrawImage 一次,无需颜色调整就绘制图像。 然后,代码再调用 DrawImage 三次,每次传递 Image 对象的地址和 ImageAttributes 对象的地址。 第二次绘制图像 (设置默认矩阵) 调用后,所有颜色的红色分量增加 50%,所有灰色的绿色分量增加 50%。 第三次在设置笔矩阵) 调用后 (绘制图像时,由笔绘制的所有颜色的蓝色分量增加 50%,由笔绘制的所有灰色的红色、绿色和蓝色分量增加了 50%。 第四次在调用 ImageAttributes::ClearColorMatrices) 后 (绘制图像时,不会对笔绘制的颜色和灰色应用任何调整。
VOID Example_SetClearColorMatrices(HDC hdc)
{
Graphics graphics(hdc);
Image image(L"TestMetafile6.emf");
ImageAttributes imAtt;
RectF rect;
Unit unit;
image.GetBounds(&rect, &unit);
ColorMatrix defaultColorMatrix = { // Multiply red component by 1.5.
1.5f, 0.0f, 0.0f, 0.0f, 0.0f,
0.0f, 1.0f, 0.0f, 0.0f, 0.0f,
0.0f, 0.0f, 1.0f, 0.0f, 0.0f,
0.0f, 0.0f, 0.0f, 1.0f, 0.0f,
0.0f, 0.0f, 0.0f, 0.0f, 1.0f};
ColorMatrix defaultGrayMatrix = { // Multiply green component by 1.5.
1.0f, 0.0f, 0.0f, 0.0f, 0.0f,
0.0f, 1.5f, 0.0f, 0.0f, 0.0f,
0.0f, 0.0f, 1.0f, 0.0f, 0.0f,
0.0f, 0.0f, 0.0f, 1.0f, 0.0f,
0.0f, 0.0f, 0.0f, 0.0f, 1.0f};
ColorMatrix penColorMatrix = { // Multiply blue component by 1.5.
1.0f, 0.0f, 0.0f, 0.0f, 0.0f,
0.0f, 1.0f, 0.0f, 0.0f, 0.0f,
0.0f, 0.0f, 1.5f, 0.0f, 0.0f,
0.0f, 0.0f, 0.0f, 1.0f, 0.0f,
0.0f, 0.0f, 0.0f, 0.0f, 1.0f};
ColorMatrix penGrayMatrix = { // Multiply all components by 1.5.
1.5f, 0.0f, 0.0f, 0.0f, 0.0f,
0.0f, 1.5f, 0.0f, 0.0f, 0.0f,
0.0f, 0.0f, 1.5f, 0.0f, 0.0f,
0.0f, 0.0f, 0.0f, 1.0f, 0.0f,
0.0f, 0.0f, 0.0f, 0.0f, 1.0f};
// Set the default color- and grayscale-adjustment matrices.
imAtt.SetColorMatrices(
&defaultColorMatrix,
&defaultGrayMatrix,
ColorMatrixFlagsAltGray,
ColorAdjustTypeDefault);
graphics.DrawImage(&image, 10.0f, 10.0f, rect.Width, rect.Height);
graphics.DrawImage(
&image,
RectF(10.0f, 50.0f, rect.Width, rect.Height), // destination rectangle
rect.X, rect.Y, // upper-left corner of source rectangle
rect.Width, // width of source rectangle
rect.Height, // height of source rectangle
UnitPixel,
&imAtt);
// Set the pen color- and grayscale-adjustment matrices.
imAtt.SetColorMatrices(
&penColorMatrix,
&penGrayMatrix,
ColorMatrixFlagsAltGray,
ColorAdjustTypePen);
graphics.DrawImage(
&image,
RectF(10.0f, 90.0f, rect.Width, rect.Height), // destination rectangle
rect.X, rect.Y, // upper-left corner of source rectangle
rect.Width, // width of source rectangle
rect.Height, // height of source rectangle
UnitPixel,
&imAtt);
imAtt.ClearColorMatrices(ColorAdjustTypePen);
graphics.DrawImage(
&image,
RectF(10.0f, 130.0f, rect.Width, rect.Height), // destination rectangle
rect.X, rect.Y, // upper-left corner of source rectangle
rect.Width, // width of source rectangle
rect.Height, // height of source rectangle
UnitPixel,
&imAtt);
}
下图显示了上述代码的输出。
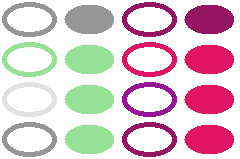
要求
最低受支持的客户端 | Windows XP、Windows 2000 Professional [仅限桌面应用] |
最低受支持的服务器 | Windows 2000 Server [仅限桌面应用] |
目标平台 | Windows |
标头 | gdiplusimageattributes.h (包括 Gdiplus.h) |
Library | Gdiplus.lib |
DLL | Gdiplus.dll |
另请参阅
ImageAttributes::ClearColorMatrix
ImageAttributes::SetColorMatrices
ImageAttributes::SetColorMatrix