進度指示器 — MRTK2
範例場景
您可以在場景中找到如何使用進度指標的 ProgressIndicatorExamples
範例。 此場景示範 SDK 中包含的每個進度指示器預製專案。 它也會示範如何使用進度指標搭配一些常見的異步工作,例如場景載入。
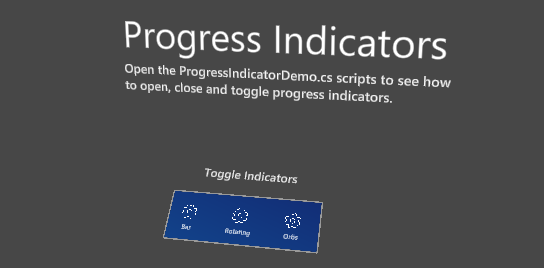
範例:開啟、更新 & 關閉進度指示器
進度指標會實作 IProgressIndicator
介面。 這個介面可以使用 從 GameObject GetComponent
擷取。
[SerializedField]
private GameObject indicatorObject;
private IProgressIndicator indicator;
private void Start()
{
indicator = indicatorObject.GetComponent<IProgressIndicator>();
}
IProgressIndicator.OpenAsync()
和 IProgressIndicator.CloseAsync()
方法會傳回Tasks。 建議您在異步方法中等候這些工作。
在場景中放置 MRTK 的預設進度指示器預製項目應該為非使用中。 呼叫其 IProgressIndicator.OpenAsync()
方法時,進度指標會自動啟動並停用其遊戲物件。 (此模式不是 IProgressIndicator 介面的需求。)
將指標的 Progress
屬性設定為 0-1 的值,以更新其顯示的進度。 設定其 Message
屬性以更新其顯示的訊息。 不同的實作可能會以不同的方式顯示此內容。
private async void OpenProgressIndicator()
{
await indicator.OpenAsync();
float progress = 0;
while (progress < 1)
{
progress += Time.deltaTime;
indicator.Message = "Loading...";
indicator.Progress = progress;
await Task.Yield();
}
await indicator.CloseAsync();
}
指標狀態
指標的 State
屬性會決定哪些作業有效。 呼叫無效的方法通常會造成指標回報錯誤,且不採取任何動作。
狀態 | 有效作業 |
---|---|
ProgressIndicatorState.Opening |
AwaitTransitionAsync() |
ProgressIndicatorState.Open |
CloseAsync() |
ProgressIndicatorState.Closing |
AwaitTransitionAsync() |
ProgressIndicatorState.Closed |
OpenAsync() |
AwaitTransitionAsync()
可用來確保指標在使用之前已完全開啟或關閉。
private async void ToggleIndicator(IProgressIndicator indicator)
{
await indicator.AwaitTransitionAsync();
switch (indicator.State)
{
case ProgressIndicatorState.Closed:
await indicator.OpenAsync();
break;
case ProgressIndicatorState.Open:
await indicator.CloseAsync();
break;
}
}