I gave up using raw SQL because I had to change too many things on the page.
EF Core - updated data retrieve problem
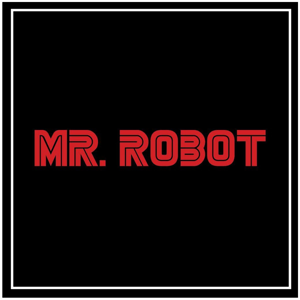
Hello,
I am trying to get updated data like this in my Blazor Server application:
async Task PassiveDetail(OrderDetail orderDetail)
{
if (orderDetail == _detailToInsert)
{
_detailToInsert = null;
}
await PassiveOrderDetailUseCase.ExecuteAsync(orderDetail); //Updates a value
_orders = await ViewAllOrdersUseCase.ExecuteAsync(user); // Retrieves data but not updated!
StateHasChanged();
}
Here is how I update a value:
public async Task PassiveOrderDetailAsync(OrderDetail orderDetail)
{
var detail = await this._db.OrdersDetail.FindAsync(orderDetail.Id);
if (detail != null)
{
detail.IsActive = 0; // 0-Passive
await _db.SaveChangesAsync();
}
}
Here is how I retrieve:
public async Task<IEnumerable<Order?>> GetAllOrders(ClaimsPrincipal user)
{
if (user.IsInRole("Administrators"))
{
return await _db.Orders.Include(d => d.OrderDetails.Where(od => od.IsActive == 1)).ThenInclude(v => v.Vendor).ToListAsync(); ;
}
return await _db.Orders.Include(d => d.OrderDetails.Where(od => od.IsActive == 1)).ThenInclude(v => v.Vendor).ToListAsync();
}
When I refresh the page the data is updated, what is the problem? Any ideas?
9 answers
Sort by: Newest
-
-
Cenk 956 Reputation points
2022-09-21T04:42:28.127+00:00 Hi @Zhi Lv - MSFT ,
When I add
AsNoTracking()
the query in my previous post gets onlyIsActive = 1
that is what I want but somehow doesn't get theCustomer
which is why I want to include it.Order
have 2 children,Customer
andOrderDetail
.OrderDetail
has one,Vendor
. Couldn't manage to includeCustomer
tough to the query. -
Cenk 956 Reputation points
2022-09-20T20:42:22.617+00:00 Seems like the loading of related data. How to add Customer to this linq? Couldn't manage.
public class Order { public int Id { get; set; } [Required] public DateTime OrderDateTime { get; set; } [Required] [MaxLength(250)] public int CustomerId { get; set; } public string Status { get; set; } [MaxLength(50)] public string DoneBy { get; set; } public List<OrderDetail> OrderDetails { get; set; } public Customer Customer { get; set; } } public class OrderDetail { public int Id { get; set; } [Required] [MaxLength(100)] public string ProductCode { get; set; } [Required] [MaxLength(250)] public string ProductName { get; set; } [Required] public int Quantity { get; set; } [Required] public double BuyUnitPrice { get; set; } public double CostRatio { get; set; } public double UnitCost { get; set; } public double TotalBuyPrice { get; set; } public double? SellUnitPrice { get; set; } public double? TotalSellPrice { get; set; } [MaxLength(150)] public string? ShippingNumber { get; set; } public string? Status { get; set; } [MaxLength(150)] public string? TrackingNumber { get; set; } [MaxLength(400)] public string? Description { get; set; } public string? Currency { get; set; } public string? CustomerStockCode { get; set; } public string? CustomerOrderNumber { get; set; } public int IsActive { get; set; } public double? TotalUnitCost { get; set; } public int OrderId { get; set; } public int VendorId { get; set; } public Order Order { get; set; } public Vendor Vendor { get; set; } } public class Customer { public int Id { get; set; } [Required] public long TaxNumber { get; set; } [Required] public string TaxAdministration { get; set; } [Required] public string Name { get; set; } [Required] public string Address { get; set; } [Required] public string DeliveryAddress { get; set; } [Required] [RegularExpression(@"^((?!\.)[\w-_.]*[^.])(@\w+)(\.\w+(\.\w+)?[^.\W])$", ErrorMessage = "Invalid email address.")] public string Email { get; set; } [Required] public string PhoneNumber { get; set; } [Required] public string MainResponsibleName { get; set; } public string AssistantResponsibleName { get; set; } } public class Vendor { public int Id { get; set; } [Required] public string Name { get; set; } [Required] public string Address { get; set; } [Required] [RegularExpression(@"^((?!\.)[\w-_.]*[^.])(@\w+)(\.\w+(\.\w+)?[^.\W])$", ErrorMessage = "Invalid email address.")] public string Email { get; set; } [Required] public string PhoneNumber { get; set; } [Required] public string MainResponsibleName { get; set; } public string AssistantResponsibleName { get; set; } public List<OrderDetail> OrderDetails { get; set; } } Linq: return await _db.Orders .Include(d => d.OrderDetails.Where(od => od.IsActive == 1)) .ThenInclude(v => v.Vendor) .AsNoTracking() .ToListAsync();
-
Cenk 956 Reputation points
2022-09-20T14:35:58.03+00:00 We cannot run your code and you refuse to share the relevant bits so we cannot review the code. It seems to me the ExecuteAsync() methods do not function as you expect or the data is set correctly but your UI is not refreshing the content.
Here is the ExecuteAsync for getting data:
public class ViewAllOrdersUseCase : IViewAllOrdersUseCase { private readonly IOrderRepository _orderRepository; public ViewAllOrdersUseCase(IOrderRepository orderRepository) { _orderRepository = orderRepository; } public async Task<IEnumerable<Order?>> ExecuteAsync(ClaimsPrincipal user) { return await _orderRepository.GetAllOrders(user); } }
Here is ExecuteAsync for updating:
public class PassiveOrderDetailUseCase : IPassiveOrderDetailUseCase { private readonly IOrderDetailRepository _orderDetailRepository; public PassiveOrderDetailUseCase(IOrderDetailRepository orderDetailRepository) { _orderDetailRepository = orderDetailRepository; } public async Task ExecuteAsync(OrderDetail orderDetail) { await _orderDetailRepository.PassiveOrderDetailAsync(orderDetail); } }
top secret codes are revealed as you wish :)
-
Cenk 956 Reputation points
2022-09-20T06:31:36.813+00:00 @Zhi Lv - MSFT do you have a solution for this issue? Why the query gets IsActive = 0?