Hi,
My application has a strange behaviour for a function I put with the help of @AgaveJoe . Definition link to generate a variable ECO [WebApiSqlite][1]
Normally the ECO variable is generated when I validate the checkboxValue1 and then I validate the Edit page.
Now the ECO variable is generated without validating the checkboxValue1 variable. The variable ECO is generated when I validate the Edit page without the checkboxValue1 variable being validated.
Edit.razor
<FormEdit ButtonText="Update" dev="dev"
OnValidSubmit="@EditDeveloper" />
@code {
[Parameter] public int developerId { get; set; }
Developer dev = new Developer();
protected async override Task OnParametersSetAsync()
{
dev = await http.GetFromJsonAsync<Developer>($"api/developer/{developerId}");
}
async Task EditDeveloper()
{
await http.PutAsJsonAsync("api/developer", dev);
await http.GetAsync($"api/developer/SelectEcoById/{developerId}");
await js.InvokeVoidAsync("alert", $"Updated Successfully!");
uriHelper.NavigateTo("developer");
}
}
as you can see on picture below ECOSelected is false.
![250593-image.png][3]
the uriHelper.NavigateTo("developer");
The fetchData .razor is active to update and fetch the data and ECOSelected become true.
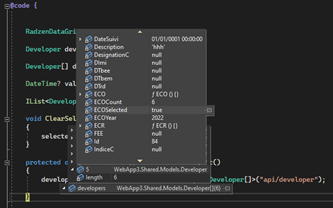
How to avoid what the variable ECOSelected stay false when checkboxValue is false? and
when if checkboxValue1 = true then ECOSelected = true?
ECOSelected ECO variable is defined in model class
public bool ECOSelected { get; set; }
[NotMapped]
public string ECO
{
get
{
if (ECOSelected)
{
return $"{ECOCount.ToString().PadLeft(3, '0')}/{ECOYear}";
}
return string.Empty;
}
set { }
}
The controller
[HttpGet("SelectEcoById/{id}")]
public async Task<ActionResult<Developer>> SelectEcoById(int id)
{
var developer = await _context.Developers.FindAsync(id);
if (developer == null)
{
return NotFound();
}
if (!developer.ECOSelected)
{
var values = await GenerateEcoByIdAsync(id);
developer.ECOYear = values.Year;
developer.ECOCount = values.Count;
developer.ECOSelected = true;
await _context.SaveChangesAsync();
}
return developer;
}
[1]: https://github.com/mjgebhard/WebApiSqlite+ [3]: /api/attachments/250593-image.png?platform=QnA