Hi @Keith Viking , first of all, this is the tutorial for using Azure AD to protect the Web API. Here's the detailed steps of what we need to do.
- Going to Azure AD to register an Azure AD app, no need to set redirect URL.
- Create a client secret for authorization, copy and save the secret value here.
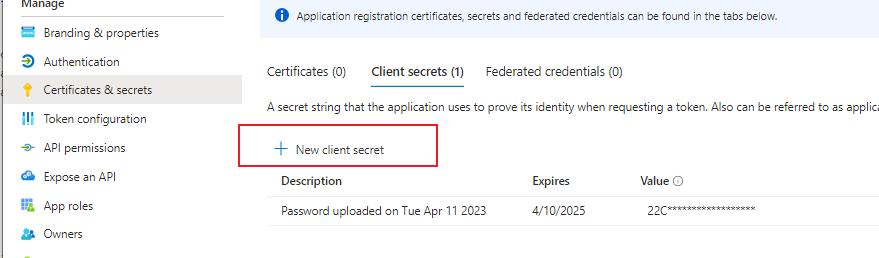
- Exposing an API, since you don't want a user to sign in, so you have to use client credential flow to generate access token, therefore you need to create a role instead of creating a scope.
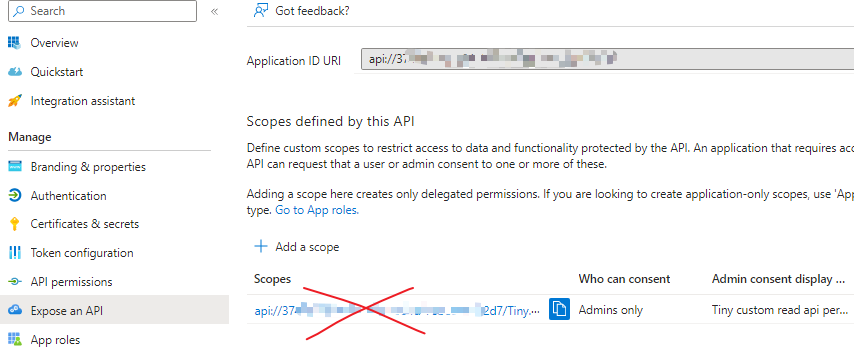
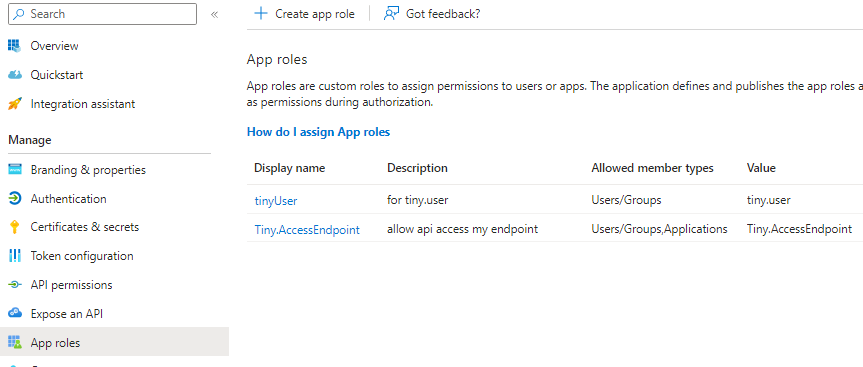
- Add API permission.
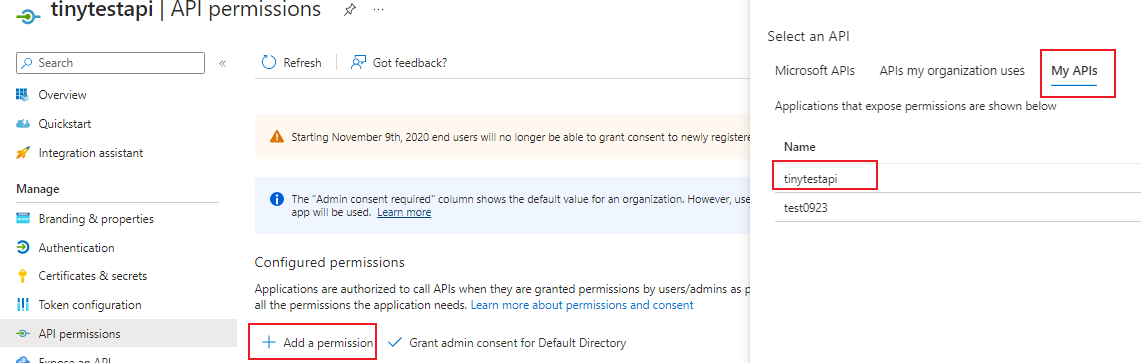
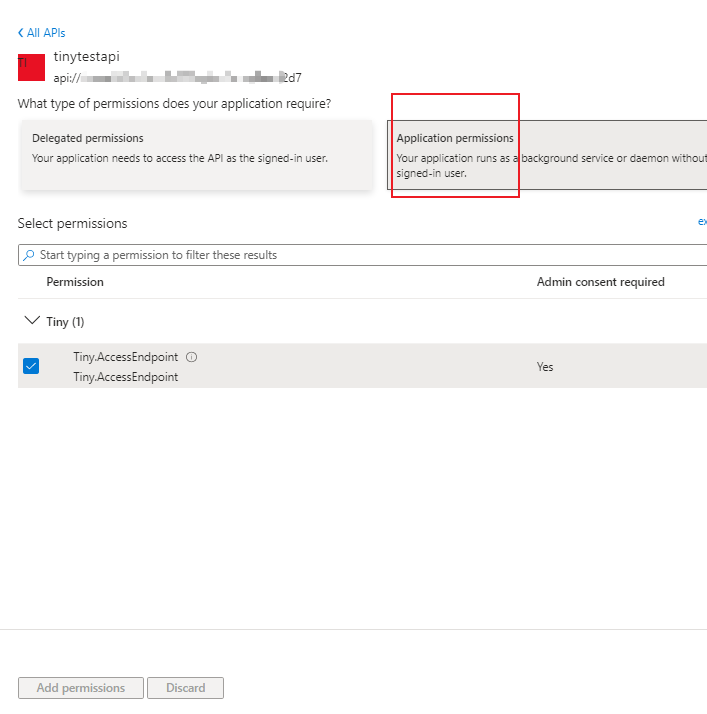
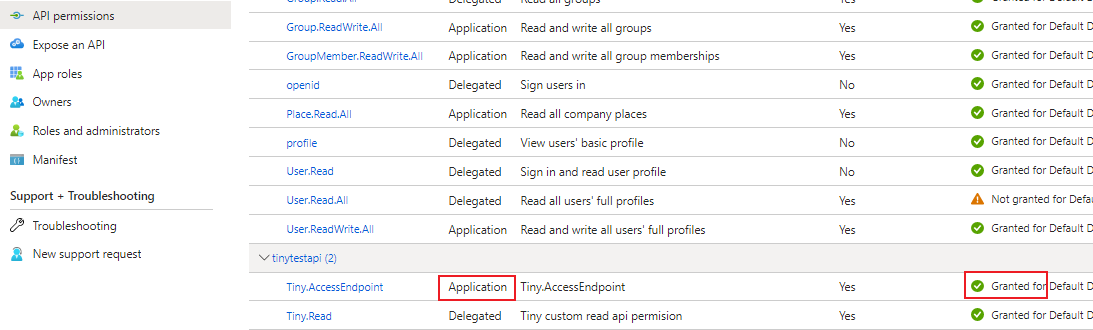
- Change Web API code. For a new .net 7 web API, having code below in the Program.cs, and add configurations in appsettings.json. Don't forget to add
[Authorize]
attribute in Controller.
using Microsoft.Identity.Web;
var builder = WebApplication.CreateBuilder(args);
// Add services to the container.
builder.Services.AddControllers();
builder.Services.AddAuthentication(JwtBearerDefaults.AuthenticationScheme)
.AddMicrosoftIdentityWebApi(builder.Configuration.GetSection("AzureAd"));
builder.Services.AddEndpointsApiExplorer();
....
....
app.UseHttpsRedirection();
app.UseAuthentication();
app.UseAuthorization();
"AzureAd": {
"Instance": "https://login.microsoftonline.com/",
"ClientId": "azure_ad_app_client_id",
"ClientSecret": "client_secret",
"Domain": "tenant_id",
"TenantId": "tenant_id",
"Audience": "api://client_id"
},
- Generate access token and calling the Web API. We can use code below to generate access token. We can also send http request to generate the token.
using Azure.Identity;
var scopes = new[] { "https://client_id/.default" };
var tenantId = "tenant_name.onmicrosoft.com";
var clientId = "your_azuread_clientid";
var clientSecret = "client_secret";
var clientSecretCredential = new ClientSecretCredential(
tenantId, clientId, clientSecret);
var tokenRequestContext = new TokenRequestContext(scopes);
var token = clientSecretCredential.GetTokenAsync(tokenRequestContext).Result.Token;
//or send post request
POST https://login.microsoftonline.com/{tenant}/oauth2/v2.0/token HTTP/1.1
Content-Type: application/x-www-form-urlencoded
client_id=azure_ad_client_id
&scope=https://client_id/.default
&client_secret=client_secret
&grant_type=client_credentials
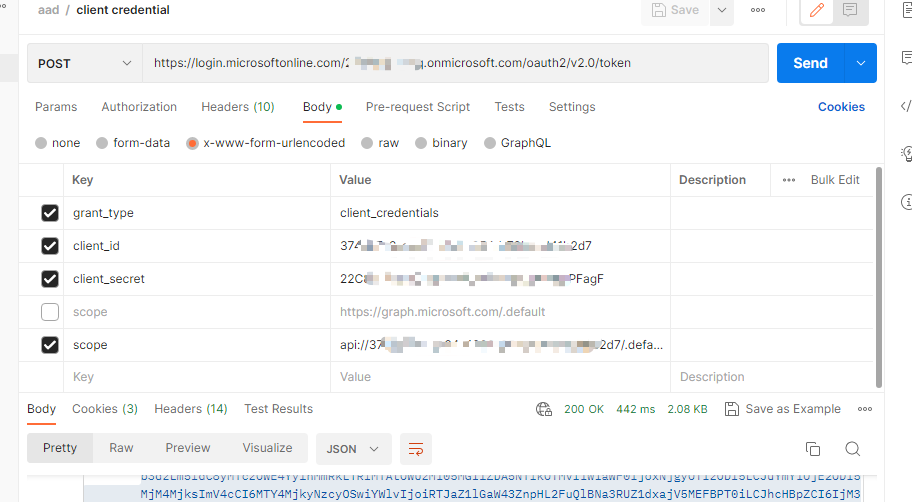
Finally using the token we got to call the web API:
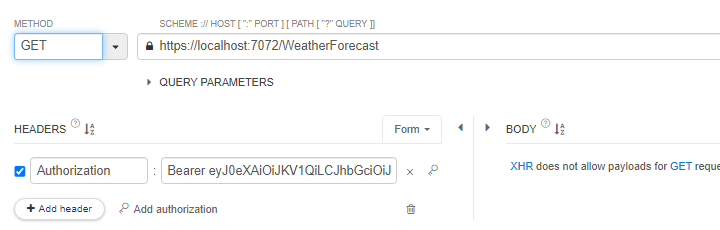
=============================================
If the answer is helpful, please click "Accept Answer" and upvote it.
Note: Please follow the steps in our documentation to enable e-mail notifications if you want to receive the related email notification for this thread.
Best Regards,
TinyWang