Hello Community,
I am trying to write a PowerShell script that uses the Microsoft Graph API to reset the password of an Azure AD user. However, I am consistently receiving an Authorization_RequestDenied
error, despite having configured the permissions for my application in Azure AD. I would greatly appreciate your assistance in resolving this issue.
Problem Summary:
I am using a PowerShell script to make a PATCH request to the Microsoft Graph API and the purpose of the script is to reset an Azure AD user's password. The script utilizes the client credentials grant flow to authenticate via an application (service principal). The application has been granted the User.ReadWrite.All
permission for Microsoft Graph, and admin consent has been provided. Despite the above configuration, my script returns an Authorization_RequestDenied
error when I attempt to make the PATCH request.
My sample code is
param (
[Parameter(Mandatory = $true)]
[string] $aadUserPrincipalName,
[Parameter(Mandatory = $true)]
[string] $newPassword
)
# Connect to Azure Account using Service Principal
$tenantId = "<tenantID>"
$appId = "<app id>"
$appSecret = "<appsecret>"
$securePassword = ConvertTo-SecureString -String $appSecret -AsPlainText -Force
$credential = New-Object -TypeName PSCredential -ArgumentList $appId, $securePassword
# Login into Azure
Connect-AzAccount -ServicePrincipal -TenantId $tenantId -Credential $credential
# Get an OAuth token for Microsoft Graph
$tokenResponse = Invoke-RestMethod -Uri "https://login.microsoftonline.com/$tenantId/oauth2/v2.0/token" -Method Post -Body @{
grant_type = "client_credentials"
client_id = $appId
client_secret = $appSecret
scope = "https://graph.microsoft.com/.default"
}
# Set Authorization header for Graph API
$headers = @{
"Authorization" = "Bearer $($tokenResponse.access_token)"
"Content-Type" = "application/json"
}
# Get the user's Object Id from UserPrincipalName
$user = Invoke-RestMethod -Uri "https://graph.microsoft.com/v1.0/users/$aadUserPrincipalName" -Headers $headers
$userObjectId = $user.id
# Set the user's new password using Microsoft Graph API
$uri = "https://graph.microsoft.com/v1.0/users/$userObjectId"
$body = @{
passwordProfile = @{
forceChangePasswordNextSignIn = $false
password = $newPassword
}
} | ConvertTo-Json
# Call Microsoft Graph API to update the password
Invoke-RestMethod -Uri $uri -Method PATCH -Headers $headers -Body $body -ContentType "application/json"
I tried adding different API permission to the applications, below are the privileges assigned at the moment
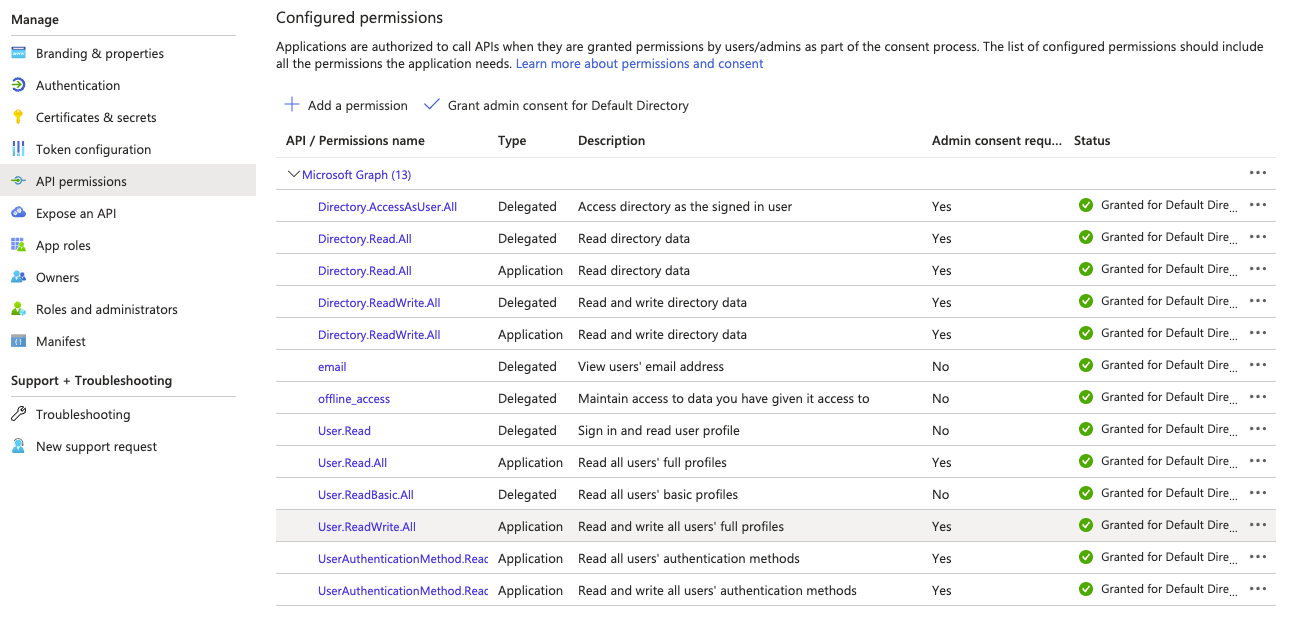
Can you please help me understand and troubleshoot the issue. Please let me know if any additional details is required to troubleshoot this issue.
Thank you in advance.

Above is the error screenshot for the output.